DispatcherTimer and EventHandler
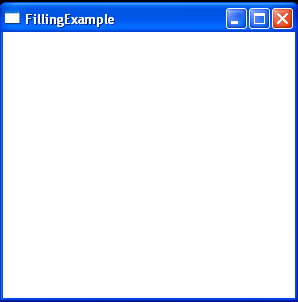
<Window x:Class="Holding.Window2"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="FillingExample" Height="300" Width="300">
<Canvas>
<Ellipse Name="myEllipse" Height="100" Fill="Red">
<Ellipse.Triggers>
<EventTrigger RoutedEvent="Ellipse.Loaded">
<BeginStoryboard>
<Storyboard>
<DoubleAnimation BeginTime="0:0:2" Duration="0:0:5"
Storyboard.TargetProperty="(Ellipse.Width)"
From="10" To="300" FillBehavior="Stop" />
</Storyboard>
</BeginStoryboard>
</EventTrigger>
</Ellipse.Triggers>
</Ellipse>
</Canvas>
</Window>
//File:Window.xaml.cs
using System;
using System.Windows;
using System.Windows.Threading;
using System.Diagnostics;
namespace Holding
{
public partial class Window2 : Window
{
DispatcherTimer t = new DispatcherTimer();
DateTime start;
public Window2()
{
InitializeComponent();
t.Tick += new EventHandler(OnTimerTick);
t.Interval = TimeSpan.FromSeconds(0.5);
t.Start();
start = DateTime.Now;
}
void OnTimerTick(object sender, EventArgs e)
{
TimeSpan elapsedTime = DateTime.Now - start;
Debug.WriteLine(elapsedTime.ToString() + ": " + myEllipse.Width);
}
}
}
Related examples in the same category