Unblock Thread with Dispatcher.BeginInvoke
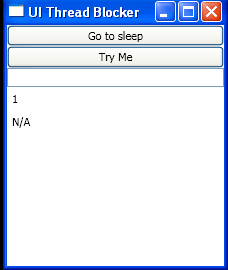
<Window x:Class="WPFThreading.UnblockThread"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="UI Thread Blocker" Height="275" Width="225">
<StackPanel>
<Button Name="button1" Click="button1_click">Go to sleep</Button>
<Button Name="button2" Click="button2_click">Try Me</Button>
<TextBox Name="textbox1"/>
<Label Name="UIThreadLabel"></Label>
<Label Name="BackgroundThreadLabel"></Label>
</StackPanel>
</Window>
//File:Window.xaml.cs
using System.Windows;
using System.Windows.Threading;
using System.Threading;
namespace WPFThreading
{
public partial class UnblockThread : System.Windows.Window
{
private delegate void SimpleDelegate();
public UnblockThread()
{
InitializeComponent();
this.UIThreadLabel.Content = this.Dispatcher.Thread.ManagedThreadId;
this.BackgroundThreadLabel.Content = "N/A";
}
private void LongRunningProcess()
{
SimpleDelegate del1 = delegate(){ this.BackgroundThreadLabel.Content = Thread.CurrentThread.ManagedThreadId; };
this.Dispatcher.BeginInvoke(DispatcherPriority.Send, del1);
Thread.Sleep(5000);
SimpleDelegate del2 = delegate() {this.textbox1.Text = "Done Sleeping...";};
this.Dispatcher.BeginInvoke(DispatcherPriority.Send, del2);
}
private void button1_click(object sender, RoutedEventArgs e)
{
SimpleDelegate del = new SimpleDelegate(LongRunningProcess);
del.BeginInvoke(null, null);
}
private void button2_click(object sender, RoutedEventArgs e)
{
this.textbox1.Text = "Hello WPF";
}
}
}
Related examples in the same category