Items that can be placed in a StatusBar
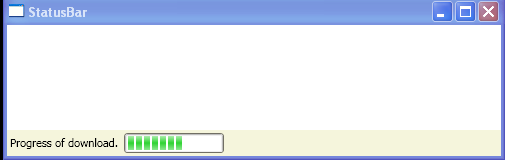
<Window xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
x:Class="StatusBarSimple.Window1"
Title ="StatusBar">
<Window.Resources>
<Style x:Key="StatusBarSeparatorStyle" TargetType="Separator">
<Setter Property="Background" Value="LightBlue" />
<Setter Property="Control.Width" Value="1"/>
<Setter Property="Control.Height" Value="20"/>
</Style>
</Window.Resources>
<StatusBar Name="sbar" Grid.Column="0" Grid.Row="2" Grid.ColumnSpan="2"
VerticalAlignment="Bottom" Background="Beige" >
<StatusBarItem>
<Button Content="click" Click="MakeProgressBar"/>
</StatusBarItem>
<StatusBarItem>
<Separator Style="{StaticResource StatusBarSeparatorStyle}"/>
</StatusBarItem>
</StatusBar>
</Window>
//File:Window.xaml.cs
using System;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Controls.Primitives;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Media.Imaging;
using System.Windows.Shapes;
namespace StatusBarSimple
{
public partial class Window1 : Window
{
private void MakeProgressBar(object sender, RoutedEventArgs e)
{
sbar.Items.Clear();
TextBlock txtb = new TextBlock();
txtb.Text = "Progress of download.";
sbar.Items.Add(txtb);
ProgressBar progressbar = new ProgressBar();
progressbar.Width = 100;
progressbar.Height = 20;
Duration duration = new Duration(TimeSpan.FromSeconds(5));
DoubleAnimation doubleanimation = new DoubleAnimation(100.0, duration);
progressbar.BeginAnimation(ProgressBar.ValueProperty,doubleanimation);
ToolTip ttprogbar = new ToolTip();
ttprogbar.Content = "Shows the progress of a download.";
progressbar.ToolTip = (ttprogbar);
sbar.Items.Add(progressbar);
}
}
}
Related examples in the same category