RoutedEvents: Drag And Drop
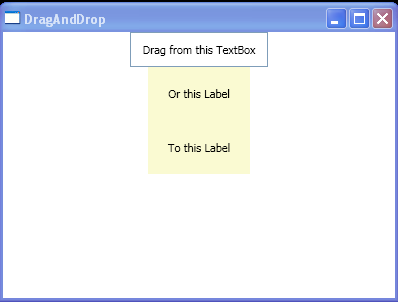
<Window x:Class="RoutedEvents.DragAndDrop"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="DragAndDrop" Height="300" Width="400">
<StackPanel>
<TextBox Padding="10" VerticalAlignment="Center" HorizontalAlignment="Center">Drag from this TextBox</TextBox>
<Label Padding="20" Background="LightGoldenrodYellow" VerticalAlignment="Center" HorizontalAlignment="Center"
MouseDown="lblSource_MouseDown">Or this Label</Label>
<Label Background="LightGoldenrodYellow"
VerticalAlignment="Center" HorizontalAlignment="Center" Padding="20"
AllowDrop="True" Drop="lblTarget_Drop">To this Label</Label>
</StackPanel>
</Window>
//File:Window.xaml.cs
using System;
using System.Collections.Generic;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Shapes;
namespace RoutedEvents
{
public partial class DragAndDrop : System.Windows.Window
{
public DragAndDrop()
{
InitializeComponent();
}
private void lblSource_MouseDown(object sender, MouseButtonEventArgs e)
{
Label lbl = (Label)sender;
DragDrop.DoDragDrop(lbl, lbl.Content, DragDropEffects.Copy);
}
private void lblTarget_Drop(object sender, DragEventArgs e)
{
((Label)sender).Content = e.Data.GetData(DataFormats.Text);
}
private void lblTarget_DragEnter(object sender, DragEventArgs e)
{
if (e.Data.GetDataPresent(DataFormats.Text))
e.Effects = DragDropEffects.Copy;
else
e.Effects = DragDropEffects.None;
}
}
}
Related examples in the same category