Sound And Video Playback in Code
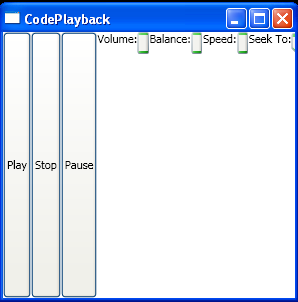
<Window x:Class="SoundAndVideo.CodePlayback"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="CodePlayback" Height="300" Width="300"
xmlns:local="clr-namespace:SoundAndVideo">
<Window.Resources>
<local:TimeSpanConverter x:Key="TimeSpanConverter"></local:TimeSpanConverter>
</Window.Resources>
<StackPanel Orientation="Horizontal">
<MediaElement Name="media" LoadedBehavior="Manual" UnloadedBehavior="Close" Source="test.mp3" MediaOpened="media_MediaOpened"></MediaElement>
<Button Click="cmdPlay_Click">Play</Button>
<Button Click="cmdStop_Click">Stop</Button>
<Button Click="cmdPause_Click">Pause</Button>
<TextBlock>Volume:</TextBlock>
<Slider Minimum="0" Maximum="1" Value="{Binding ElementName=media, Path=Volume, Mode=TwoWay}"></Slider>
<TextBlock>Balance: </TextBlock>
<Slider Minimum="-1" Maximum="1" Value="{Binding ElementName=media, Path=Balance, Mode=TwoWay}"></Slider>
<TextBlock>Speed: </TextBlock>
<Slider Minimum="0" Maximum="2" Value="{Binding ElementName=media, Path=SpeedRatio}"></Slider>
<TextBlock>Seek To: </TextBlock>
<Slider Minimum="0" Name="sliderPosition" TickPlacement="BottomRight" TickFrequency="1"
ValueChanged="sliderPosition_ValueChanged"></Slider>
<TextBlock Name="lblTime"></TextBlock>
</StackPanel>
</Window>
//File:Window.xaml.cs
using System;
using System.Collections.Generic;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Shapes;
using System.Windows.Media.Animation;
using System;
using System.Collections.Generic;
using System.Text;
using System.Windows.Data;
namespace SoundAndVideo
{
public partial class CodePlayback : System.Windows.Window
{
public CodePlayback()
{
InitializeComponent();
}
private void sliderSpeed_ValueChanged(object sender, RoutedEventArgs e)
{
media.SpeedRatio = ((Slider)sender).Value;
}
private void cmdPlay_Click(object sender, RoutedEventArgs e)
{
media.Play();
}
private void cmdPause_Click(object sender, RoutedEventArgs e)
{
media.Pause();
}
private void cmdStop_Click(object sender, RoutedEventArgs e)
{
media.Stop();
media.SpeedRatio = 1;
}
private void media_MediaOpened(object sender, RoutedEventArgs e)
{
sliderPosition.Maximum = media.NaturalDuration.TimeSpan.TotalSeconds;
}
private void sliderPosition_ValueChanged(object sender, RoutedEventArgs e)
{
media.Pause();
media.Position = TimeSpan.FromSeconds(sliderPosition.Value);
media.Play();
}
}
public class TimeSpanConverter : IValueConverter
{
public object Convert(object value, Type targetType, object parameter,System.Globalization.CultureInfo culture)
{
try
{
TimeSpan time = (TimeSpan)value;
return time.TotalSeconds;
}
catch
{
return 0;
}
}
public object ConvertBack(object value, Type targetType, object parameter,System.Globalization.CultureInfo culture)
{
double seconds = (double)value;
return TimeSpan.FromSeconds(seconds);
}
}
}
Related examples in the same category