ThreadPool.QueueUserWorkItem
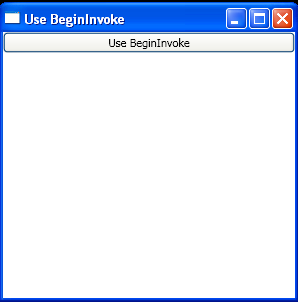
<Window x:Class="DispatcherExamples.MyWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Use BeginInvoke" Height="300" Width="300"
>
<Grid>
<StackPanel>
<Button x:Name="useBeginInvokeButton" Content="Use BeginInvoke" />
</StackPanel>
</Grid>
</Window>
//File:Window.xaml.cs
using System;
using System.Collections.Generic;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Shapes;
using System.Windows.Threading;
using System.Threading;
namespace DispatcherExamples
{
public partial class MyWindow : System.Windows.Window
{
public MyWindow()
{
InitializeComponent();
useBeginInvokeButton.Click += new RoutedEventHandler(useBeginInvokeButton_Click);
}
void useBeginInvokeButton_Click(object sender, RoutedEventArgs e)
{
ThreadPool.QueueUserWorkItem(delegate
{
MyDelegateType methodForUiThread = delegate
{
this.Background = new SolidColorBrush(Color.FromRgb(3,2, 1));
};
this.Dispatcher.BeginInvoke(DispatcherPriority.Normal, methodForUiThread);
});
}
public delegate void MyDelegateType();
}
}
Related examples in the same category