Use the content-scrolling methods of the ScrollViewer class
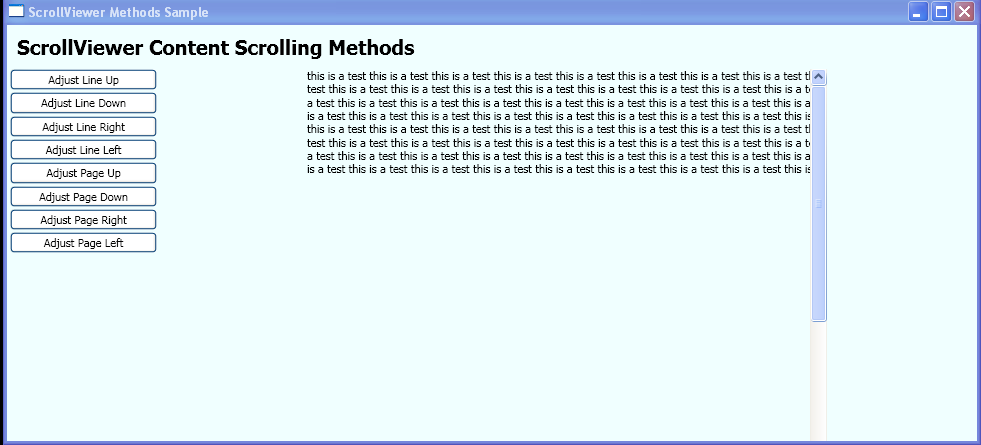
<Window xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
x:Class="ScrollViewer_Methods.Window1"
Title="ScrollViewer Methods Sample"
Loaded="onLoad">
<DockPanel Background="Azure">
<TextBlock DockPanel.Dock="Top" FontSize="20" FontWeight="Bold" Margin="10">ScrollViewer Content Scrolling Methods</TextBlock>
<StackPanel DockPanel.Dock="Left" Width="150">
<Button Margin="3,0,0,2" Background="White" Click="svLineUp">Adjust Line Up</Button>
<Button Margin="3,0,0,2" Background="White" Click="svLineDown">Adjust Line Down</Button>
<Button Margin="3,0,0,2" Background="White" Click="svLineRight">Adjust Line Right</Button>
<Button Margin="3,0,0,2" Background="White" Click="svLineLeft">Adjust Line Left</Button>
<Button Margin="3,0,0,2" Background="White" Click="svPageUp">Adjust Page Up</Button>
<Button Margin="3,0,0,2" Background="White" Click="svPageDown">Adjust Page Down</Button>
<Button Margin="3,0,0,2" Background="White" Click="svPageRight">Adjust Page Right</Button>
<Button Margin="3,0,0,2" Background="White" Click="svPageLeft">Adjust Page Left</Button>
<TextBlock Name="txt2" TextWrapping="Wrap"/>
</StackPanel>
<Border BorderBrush="Black" Height="520" Width="520" VerticalAlignment="Top">
<ScrollViewer VerticalScrollBarVisibility="Visible" HorizontalScrollBarVisibility="Auto" Name="sv1">
<TextBlock TextWrapping="Wrap" Width="800" Height="1000" Name="txt1"/>
</ScrollViewer>
</Border>
</DockPanel>
</Window>
//File:Window.xaml.cs
using System;
using System.Windows;
using System.Windows.Automation.Provider;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Navigation;
using System.Text;
namespace ScrollViewer_Methods
{
public partial class Window1 : Window
{
private void onLoad(object sender, System.EventArgs e)
{
StringBuilder myStringBuilder = new StringBuilder(400);
for (int i = 0; i < 100; i++)
{
myStringBuilder.Append("this is a test ");
}
txt1.Text = myStringBuilder.ToString();
}
private void svLineUp(object sender, RoutedEventArgs e)
{
sv1.LineUp();
}
private void svLineDown(object sender, RoutedEventArgs e)
{
sv1.LineDown();
}
private void svLineRight(object sender, RoutedEventArgs e)
{
sv1.LineRight();
}
private void svLineLeft(object sender, RoutedEventArgs e)
{
sv1.LineLeft();
}
private void svPageUp(object sender, RoutedEventArgs e)
{
sv1.PageUp();
}
private void svPageDown(object sender, RoutedEventArgs e)
{
sv1.PageDown();
}
private void svPageRight(object sender, RoutedEventArgs e)
{
sv1.PageRight();
}
private void svPageLeft(object sender, RoutedEventArgs e)
{
sv1.PageLeft();
}
}
}
Related examples in the same category