Static Member Functions: its strictions
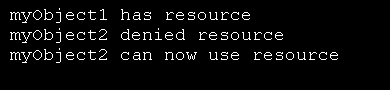
//A static member function does not have a this pointer.
//cannot be a static and a non-static version of the same function.
//A static member function may not be virtual.
//static functions cannot be declared as const or volatile.
#include <iostream>
using namespace std;
class MyClass {
static int resource;
public:
static int getResource();
void freeResource() {
resource = 0;
}
};
int MyClass::resource; // define resource
int MyClass::getResource()
{
if(resource) return 0; // resource already in use
else {
resource = 1;
return 1; // resource allocated to this object
}
}
int main()
{
MyClass myObject1, myObject2;
if(MyClass::getResource())
cout << "myObject1 has resource\n";
if(!MyClass::getResource())
cout << "myObject2 denied resource\n";
myObject1.freeResource();
if(myObject2.getResource())
cout << "myObject2 can now use resource\n";
return 0;
}
Related examples in the same category