Use a static member variable independent of any object.
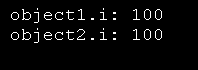
#include <iostream>
using namespace std;
class myclass {
public:
static int i;
void setInt(int n) {
i = n;
}
int getInt() {
return i;
}
};
int myclass::i;
int main()
{
myclass object1, object2;
myclass::i = 100; // set i directly, no object is referenced.
cout << "object1.i: " << object1.getInt() << '\n'; // displays 100
cout << "object2.i: " << object2.getInt() << '\n'; // also displays 100
return 0;
}
Related examples in the same category