Illustrates how to display a ball lit by a red light
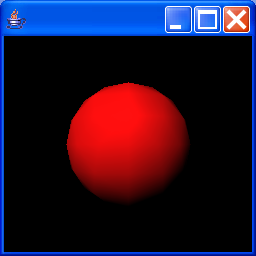
/*
The Joy of Java 3D
by Greg Hopkins
Copyright Copyright 2001
*/
/*
Lighting up the World. The way the light falls on an object provides us with the
shading that helps us see shapes in three dimensions
The next example illustrates how to display a ball lit by a red light:
The sphere we created is white (the default), it appears red because of the colored
light. Since it is a DirectionalLight, we also have to specify how far the light
shines and in what direction. In the example, the light shines for 100 meters from
the origin and the direction is to the right, down and into the screen (this is defined
by the vector: 4.0 right, -7.0 down, and -12.0 into the screen).
You can also create an AmbientLight which will produce a directionless light, or a
SpotLight if you want to focus on a particular part of your scene. A combination of a
strong directional light and a weaker ambient light gives a natural-looking appearance to
your scene. Java 3D lights do not produce shadows.
*/
import javax.media.j3d.BoundingSphere;
import javax.media.j3d.BranchGroup;
import javax.media.j3d.DirectionalLight;
import javax.vecmath.Color3f;
import javax.vecmath.Point3d;
import javax.vecmath.Vector3f;
import com.sun.j3d.utils.geometry.Sphere;
import com.sun.j3d.utils.universe.SimpleUniverse;
public class Ball {
public Ball() {
// Create the universe
SimpleUniverse universe = new SimpleUniverse();
// Create a structure to contain objects
BranchGroup group = new BranchGroup();
// Create a ball and add it to the group of objects
Sphere sphere = new Sphere(0.5f);
group.addChild(sphere);
// Create a red light that shines for 100m from the origin
Color3f light1Color = new Color3f(1.8f, 0.1f, 0.1f);
BoundingSphere bounds = new BoundingSphere(new Point3d(0.0, 0.0, 0.0),
100.0);
Vector3f light1Direction = new Vector3f(4.0f, -7.0f, -12.0f);
DirectionalLight light1 = new DirectionalLight(light1Color,
light1Direction);
light1.setInfluencingBounds(bounds);
group.addChild(light1);
// look towards the ball
universe.getViewingPlatform().setNominalViewingTransform();
// add the group of objects to the Universe
universe.addBranchGraph(group);
}
public static void main(String[] args) {
new Ball();
}
}
Related examples in the same category