Indexed Set : Set « Collections Data Structure « Java
- Java
- Collections Data Structure
- Set
Indexed Set
//package gr.forth.ics.util;
import java.util.AbstractCollection;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Set;
/**
*
* @author Andreou Dimitris, email: jim.andreou (at) gmail (dot) com
*/
public class IndexedSet<T> extends AbstractCollection<T> implements Set<T> {
private final List<T> list = new ArrayList<T>();
private final Set<T> set = new HashSet<T>();
public int size() {
return list.size();
}
@Override
public boolean isEmpty() {
return list.isEmpty();
}
@Override
public boolean contains(Object o) {
return set.contains(o);
}
public Iterator<T> iterator() {
return new Iterator<T>() {
Iterator<T> realIterator = list.iterator();
T current = null;
public boolean hasNext() {
return realIterator.hasNext();
}
public T next() {
T next = realIterator.next();
current = next;
return next;
}
public void remove() {
realIterator.remove();
set.remove(current);
current = null;
}
};
}
@Override
public Object[] toArray() {
return list.toArray();
}
@Override
public <T> T[] toArray(T[] a) {
return list.toArray(a);
}
@Override
public boolean add(T e) {
boolean added = set.add(e);
if (added) {
list.add(e);
}
return added;
}
@Override
public boolean remove(Object o) {
boolean removed = set.remove(o);
if (removed) {
list.remove(o);
}
return removed;
}
@Override
public boolean containsAll(Collection<?> c) {
return set.containsAll(c);
}
@Override
public boolean retainAll(Collection<?> c) {
boolean removed = false;
Iterator<T> it = list.iterator();
while (it.hasNext()) {
T next = it.next();
if (!c.contains(next)) {
it.remove();
set.remove(it);
removed = true;
}
}
return removed;
}
public void clear() {
set.clear();
list.clear();
}
public T get(int index) {
return list.get(index);
}
public T remove(int index) {
T t = list.get(index);
remove(t);
return t;
}
}
Related examples in the same category
1. | Set, HashSet and TreeSet | | |
2. | Things you can do with Sets | |  |
3. | Set operations: union, intersection, difference, symmetric difference, is subset, is superset | | |
4. | Set implementation that use == instead of equals() | | |
5. | Set that compares object by identity rather than equality | | |
6. | Set union and intersection | | |
7. | Set with values iterated in insertion order. | | |
8. | Putting your own type in a Set | | 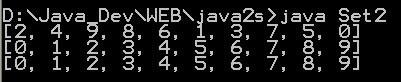 |
9. | Use set | |  |
10. | Another Set demo | | |
11. | Set subtraction | |  |
12. | Working with HashSet and TreeSet | | 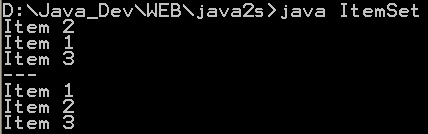 |
13. | TreeSet Demo | | 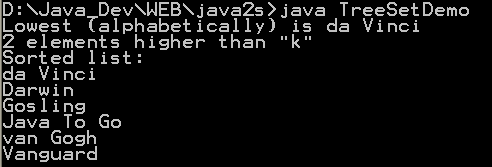 |
14. | Show the union and intersection of two sets | | |
15. | Demonstrate the Set interface | | |
16. | Array Set extends AbstractSet | | 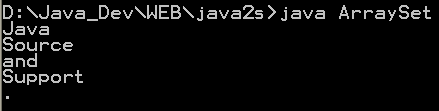 |
17. | Sync Test | | |
18. | Set Copy | | |
19. | Set and TreeSet | | |
20. | Tail | | |
21. | What you can do with a TreeSet | | 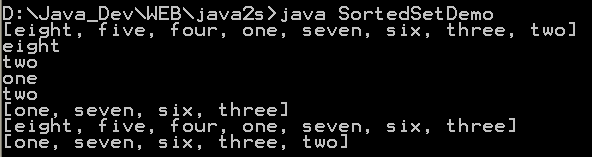 |
22. | Remove all elements from a set | | |
23. | Copy all the elements from set2 to set1 (set1 += set2), set1 becomes the union of set1 and set2 | | |
24. | Remove all the elements in set1 from set2 (set1 -= set2), set1 becomes the asymmetric difference of set1 and set2 | | |
25. | Get the intersection of set1 and set2, set1 becomes the intersection of set1 and set2 | | |
26. | Extend AbstractSet to Create Simple Set | | |
27. | Int Set | | |
28. | One Item Set | | |
29. | Small sets whose elements are known to be unique by construction | | |
30. | List Set implements Set | | |
31. | Converts a char array to a Set | | |
32. | Converts a string to a Set | | |
33. | Implements the Set interface, backed by a ConcurrentHashMap instance | | |
34. | An IdentitySet that uses reference-equality instead of object-equality | | |
35. | An implementation of the java.util.Stack based on an ArrayList instead of a Vector, so it is not synchronized to protect against multi-threaded access. | | |
36. | A thin wrapper around a List transforming it into a modifiable Set. | | |
37. | A thread-safe Set that manages canonical objects | | |
38. | This program uses a set to print all unique words in System.in | | |
39. | Indexed Set | | |
40. | An ObjectToSet provides a java.util.Map from arbitrary objects to objects of class java.util.Set. | | |
41. | Sorted Multi Set | | |
42. | Fixed Size Sorted Set | | |
43. | Set operations | | |
44. | A NumberedSet is a generic container of Objects where each element is identified by an integer id. | | |
45. | Set which counts the number of times a values are added to it. | | |
46. | Set which counts the number of times a values are added to it and assigns them a unique positive index. | | |
47. | A set acts like array. | | |
48. | Implements a Bloom filter. Which, as you may not know, is a space-efficient structure for storing a set. | | |
49. | Implementation of disjoint-set data structure | | |
50. | Call it an unordered list or a multiset, this collection is defined by oxymorons | | |