Decimal Format with different Symbols
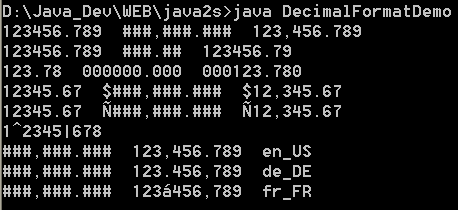
/*
* Copyright (c) 1995 - 2008 Sun Microsystems, Inc. All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* - Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
*
* - Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
*
* - Neither the name of Sun Microsystems nor the names of its
* contributors may be used to endorse or promote products derived
* from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS
* IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO,
* THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
* PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR
* CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL,
* EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO,
* PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR
* PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF
* LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING
* NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
* SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
import java.text.DecimalFormat;
import java.text.DecimalFormatSymbols;
import java.text.NumberFormat;
import java.util.Locale;
public class DecimalFormatDemo {
static public void customFormat(String pattern, double value) {
DecimalFormat myFormatter = new DecimalFormat(pattern);
String output = myFormatter.format(value);
System.out.println(value + " " + pattern + " " + output);
}
static public void localizedFormat(String pattern, double value, Locale loc) {
NumberFormat nf = NumberFormat.getNumberInstance(loc);
DecimalFormat df = (DecimalFormat) nf;
df.applyPattern(pattern);
String output = df.format(value);
System.out.println(pattern + " " + output + " " + loc.toString());
}
static public void main(String[] args) {
customFormat("###,###.###", 123456.789);
customFormat("###.##", 123456.789);
customFormat("000000.000", 123.78);
customFormat("$###,###.###", 12345.67);
customFormat("\u00a5###,###.###", 12345.67);
Locale currentLocale = new Locale("en", "US");
DecimalFormatSymbols unusualSymbols = new DecimalFormatSymbols(
currentLocale);
unusualSymbols.setDecimalSeparator('|');
unusualSymbols.setGroupingSeparator('^');
String strange = "#,##0.###";
DecimalFormat weirdFormatter = new DecimalFormat(strange, unusualSymbols);
weirdFormatter.setGroupingSize(4);
String bizarre = weirdFormatter.format(12345.678);
System.out.println(bizarre);
Locale[] locales = { new Locale("en", "US"), new Locale("de", "DE"),
new Locale("fr", "FR") };
for (int i = 0; i < locales.length; i++) {
localizedFormat("###,###.###", 123456.789, locales[i]);
}
}
}
Related examples in the same category
1. | Date Era change | |  |
2. | Date Format | | |
3. | The Time and Date Format Suffixes | | |
4. | Display standard 12-hour time format | | |
5. | Display complete time and date information | | |
6. | Display just hour and minute | | |
7. | Display month by name and number | | |
8. | DateFormat.getDateInstance(DateFormat.SHORT) | | |
9. | Use relative indexes to simplify the creation of a custom time and date format. | | |
10. | Date Format with Locale | |  |
11. | Date Format Symbols | | 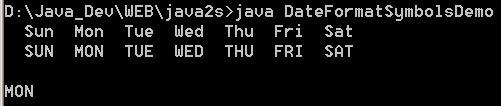 |
12. | Date format: "dd.MM.yy", "yyyy.MM.dd G 'at' hh:mm:ss z","EEE, MMM d, ''yy", "h:mm a", "H:mm", "H:mm:ss:SSS", "K:mm a,z","yyyy.MMMMM.dd GGG hh:mm aaa" | | 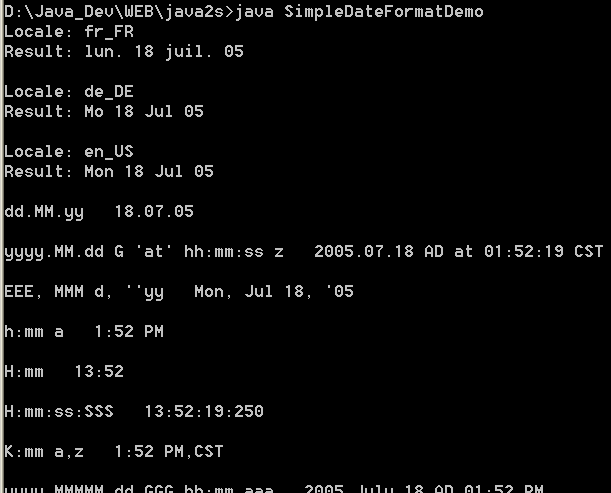 |
13. | SimpleDateFormat.getAvailableLocales | | 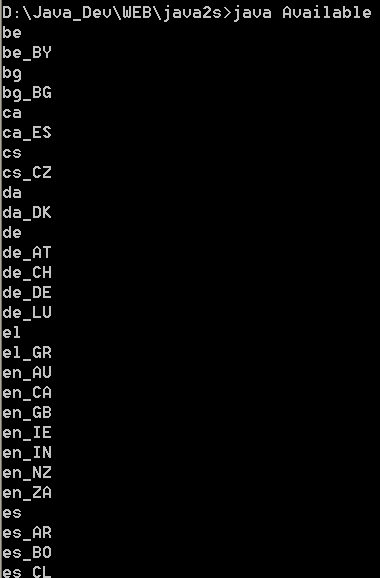 |
14. | DateFormat.SHORT | | |
15. | This is same as MEDIUM: DateFormat.getDateInstance().format(new Date()) | | |
16. | This is same as MEDIUM: DateFormat.getDateInstance(DateFormat.DEFAULT).format(new Date()) | | |
17. | DateFormat.getTimeInstance(DateFormat.MEDIUM, Locale.CANADA).format(new Date()) | | |
18. | DateFormat.getTimeInstance(DateFormat.LONG, Locale.CANADA).format(new Date()) | | |
19. | DateFormat.getTimeInstance(DateFormat.FULL, Locale.CANADA).format(new Date()) | | |
20. | DateFormat.getTimeInstance(DateFormat.DEFAULT, Locale.CANADA).format(new Date()) | | |
21. | DateFormat.getDateInstance(DateFormat.LONG) | | |
22. | DateFormat.getTimeInstance(DateFormat.SHORT) | | |
23. | DateFormat.getTimeInstance(DateFormat.LONG) | | |
24. | Parse date string input with DateFormat.getTimeInstance(DateFormat.DEFAULT, Locale.CANADA) | | |
25. | Simple Date Format Demo | | 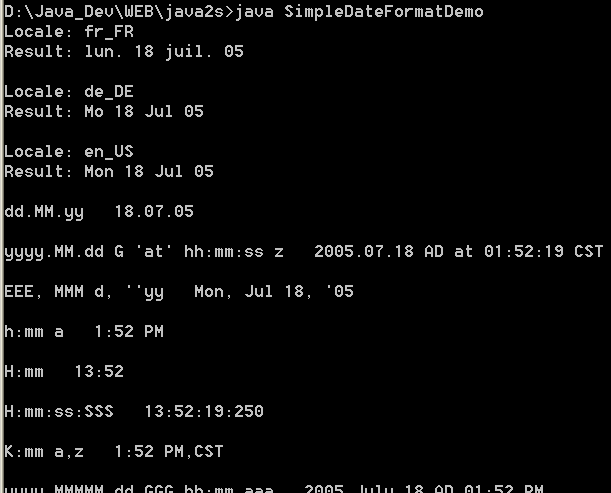 |
26. | Format date in Medium format | | |
27. | Format date in Long format | | |
28. | Format date in Full format | | |
29. | Format date in Default format | | |
30. | Formatting day of week using SimpleDateFormat | | |
31. | Formatting day of week in EEEE format like Sunday, Monday etc. | | |
32. | Formatting day in d format like 1,2 etc | | |
33. | Formatting day in dd format like 01, 02 etc. | | |
34. | Format hour in h (1-12 in AM/PM) format like 1, 2..12. | | |
35. | Format hour in hh (01-12 in AM/PM) format like 01, 02..12. | | |
36. | Format hour in H (0-23) format like 0, 1...23. | | |
37. | Format hour in HH (00-23) format like 00, 01..23. | | |
38. | Format hour in k (1-24) format like 1, 2..24. | | |
39. | Format hour in kk (01-24) format like 01, 02..24. | | |
40. | Format hour in K (0-11 in AM/PM) format like 0, 1..11. | | |
41. | Format hour in KK (00-11) format like 00, 01,..11. | | |
42. | Formatting minute in m format like 1,2 etc. | | |
43. | Format minutes in mm format like 01, 02 etc. | | |
44. | Format month in M format like 1,2 etc | | |
45. | Format Month in MM format like 01, 02 etc. | | |
46. | Format Month in MMM format like Jan, Feb etc. | | |
47. | Format Month in MMMM format like January, February etc. | | |
48. | Format seconds in s format like 1,2 etc. | | |
49. | Format seconds in ss format like 01, 02 etc. | | |
50. | Format date in dd/mm/yyyy format | | |
51. | Format date in mm-dd-yyyy hh:mm:ss format | | |
52. | Format year in yy format like 07, 08 etc | | |
53. | Format year in yyyy format like 2007, 2008 etc. | | |
54. | new SimpleDateFormat("hh") | | |
55. | new SimpleDateFormat("H") // The hour (0-23) | | |
56. | new SimpleDateFormat("m"): The minutes | | |
57. | new SimpleDateFormat("mm") | | |
58. | SimpleDateFormat("MM"): number based month value | | |
59. | new SimpleDateFormat("s"): The seconds | | |
60. | new SimpleDateFormat("ss") | | |
61. | new SimpleDateFormat("a"): The am/pm marker | | |
62. | new SimpleDateFormat("z"): The time zone | | |
63. | new SimpleDateFormat("zzzz") | | |
64. | new SimpleDateFormat("Z") | | |
65. | new SimpleDateFormat("hh:mm:ss a") | | |
66. | new SimpleDateFormat("HH.mm.ss") | | |
67. | new SimpleDateFormat("HH:mm:ss Z") | | |
68. | SimpleDateFormat("MM/dd/yy") | | |
69. | SimpleDateFormat("dd-MMM-yy") | | |
70. | SimpleDateFormat("E, dd MMM yyyy HH:mm:ss Z") | | |
71. | SimpleDateFormat("yyyy") | | |
72. | The month: SimpleDateFormat("M") | | |
73. | Three letter-month value: SimpleDateFormat("MMM") | | |
74. | Full length of month name: SimpleDateFormat("MMMM") | | |
75. | The day number: SimpleDateFormat("d") | | |
76. | Two digits day number: SimpleDateFormat("dd") | | |
77. | The day in week: SimpleDateFormat("E") | | |
78. | Full day name: SimpleDateFormat("EEEE") | | |
79. | Add AM PM to time using SimpleDateFormat | | |
80. | Simply format a date as "YYYYMMDD" | | |
81. | Java SimpleDateFormat Class Example("MM/dd/yyyy") | | |
82. | The format used is EEE, dd MMM yyyy HH:mm:ss Z in US locale. | | |
83. | Date Formatting and Localization | | |
84. | Get a List of Short Month Names | | |
85. | Get a List of Weekday Names | | |
86. | Get a List of Short Weekday Names | | |
87. | Change date formatting symbols | | |
88. | An alternate way to get week days symbols | | |
89. | ISO8601 formatter for date-time without time zone.The format used is yyyy-MM-dd'T'HH:mm:ss. | | |
90. | ISO8601 formatter for date-time with time zone. The format used is yyyy-MM-dd'T'HH:mm:ssZZ. | | |
91. | Parsing custom formatted date string into Date object using SimpleDateFormat | | |
92. | Parse with a custom format | | |
93. | Parsing the Time Using a Custom Format | | |
94. | Parse with a default format | | |
95. | Parse a date and time | | |
96. | Parse string date value input with SimpleDateFormat("E, dd MMM yyyy HH:mm:ss Z") | | |
97. | Parse string date value input with SimpleDateFormat("dd-MMM-yy") | | |
98. | Parse string date value with default format: DateFormat.getDateInstance(DateFormat.DEFAULT) | | |
99. | Find the current date format | | |
100. | Time format viewer | | |
101. | Date format viewer | | |
102. | Returns a String in the format Xhrs, Ymins, Z sec, for the time difference between two times | | |
103. | format Duration | | |
104. | Get Date Suffix | | |
105. | Date Format Cache | | |
106. | ISO8601 Date Format | | |
107. | Explode a date in 8 digit format into the three components. | | |
108. | Date To Iso Date Time | | |
109. | Iso Date Time To Date | | |
110. | Gets formatted time | | |
111. | Format Time To 2 Digits | | |
112. | Time formatting utility. | | |
113. | ISO 8601 BASIC date format | | |
114. | Format As MySQL Datetime | | |
115. | new SimpleDateFormat( "EEE MMM d HH:mm:ss z yyyy", Locale.UK ) | | |
116. | Date parser for the ISO 8601 format. | | |
117. | Parse W3C Date format | | |
118. | Pack/Unpacks date stored in kdb format | | |
119. | Provides preset formatting for Dates. All dates are returned as GMT | | |
120. | Parse RSS date format to Date object. | | |
121. | Date format for face book | | |
122. | FastDateFormat is a fast and thread-safe version of java.text.SimpleDateFormat. | | |
123. | Date format and parse Util | | |
124. | XSD Date Time | | |
125. | Return a String value of Now() in a specify format | | |
126. | Format data to string with specified style. | | |
127. | extends Formatter | | |