Performs continuous matching of a pattern in a given string.
// Useful when looping for the records within the search results.
/*
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. The ASF licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
*/
//package org.opentides.util;
import java.util.ArrayList;
import java.util.List;
//import org.apache.log4j.Logger;
/**
* This class performs continuous matching of a pattern in a given string.
* Useful when looping for the records within the search results.
*
* @author allanctan
*/
public class BlockSplitter {
//private static Logger _log = Logger.getLogger(BlockSplitter.class);
private String prePattern;
private String endPattern;
/**
* @param prePattern - starting pattern
* @param endPattern - ending pattern
*/
public BlockSplitter(String prePattern, String endPattern) {
super();
this.prePattern = prePattern;
this.endPattern = endPattern;
}
/**
* Performs matching for the declared prePattern and endPattern
* @return - the next matching string. null if not found.
*/
public List<String> split(String code) {
int startIndex = 0;
List<String> ret = new ArrayList<String>();
//if (StringUtil.isEmpty(code))
//return ret;
while (startIndex < code.length()) {
int preIndex = code.indexOf(prePattern, startIndex);
if ( preIndex != -1 ) {
ret.add(code.substring(startIndex, preIndex));
int endIndex = code.indexOf(endPattern, preIndex);
if (endIndex != -1) {
startIndex = endIndex+endPattern.length();
ret.add(code.substring(preIndex+prePattern.length(), endIndex));
} else {
// _log.error("No matching end string ["+prePattern+"] found for ["+endPattern+"]");
ret.remove(ret.size()-1);
ret.add(code.substring(startIndex));
break;
}
} else {
// no more match found
ret.add(code.substring(startIndex));
break;
}
}
return ret;
}
}
----------------
package org.opentides.util;
import org.junit.Assert;
import org.junit.Test;
public class BlockSplitterTest {
@Test
public void testSplitComment() {
BlockSplitter split = new BlockSplitter("/*","*/");
String test1 = "Hello /* Comment */ ko to";
String[] expected1 = {"Hello ", " Comment ", " ko to"};
Assert.assertArrayEquals(expected1, split.split(test1).toArray());
String test2 = "Hello /* Comment */";
String[] expected2 = {"Hello ", " Comment "};
Assert.assertArrayEquals(expected2, split.split(test2).toArray());
String test3 = "/* Comment */ ko to";
String[] expected3 = {""," Comment ", " ko to"};
Assert.assertArrayEquals(expected3, split.split(test3).toArray());
}
@Test
public void testNoSplit() {
BlockSplitter split = new BlockSplitter("/*","*/");
String test1 = "Hello /* Comment";
String[] expected1 = {"Hello /* Comment"};
Assert.assertArrayEquals(expected1, split.split(test1).toArray());
String test2 = "";
String[] expected2 = {};
Assert.assertArrayEquals(expected2, split.split(test2).toArray());
}
}
Related examples in the same category
1. | String Region Match Demo | |  |
2. | Palindrome | | 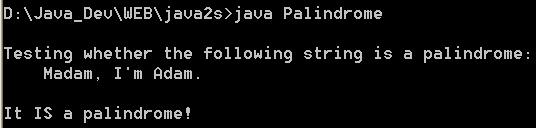 |
3. | Look for particular sequences in sentences | | 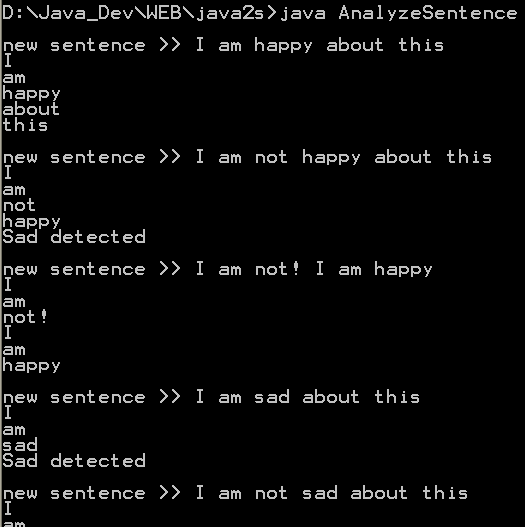 |
4. | Strings -- extract printable strings from binary file | | |
5. | Java Search String | | |
6. | Java String endsWith | | |
7. | Java String startsWith | | |
8. | Search a substring Anywhere | | |
9. | Starts with, ignore case( regular expressions ) | | |
10. | Ends with, ignore case( regular expressions ) | | |
11. | Anywhere, ignore case( regular expressions ) | | |
12. | Searching a String for a Character or a Substring | | |
13. | Not found returns -1 | | |
14. | If a string contains a specific word | | |
15. | Not found | | |
16. | if a String starts with a digit or uppercase letter | | |
17. | Search a String to find the first index of any character in the given set of characters. | | |
18. | Search a String to find the first index of any character not in the given set of characters. | | |
19. | Searches a String for substrings delimited by a start and end tag, returning all matching substrings in an array. | | |
20. | Helper functions to query a strings end portion. The comparison is case insensitive. | | |
21. | Helper functions to query a strings start portion. The comparison is case insensitive. | | |
22. | Wrapper for arrays of ordered strings. This verifies the arrays and supports efficient lookups. | | |
23. | Returns an index into arra (or -1) where the character is not in the charset byte array. | | |
24. | Returns an int[] array of length segments containing the distribution count of the elements in unsorted int[] array with values between min and max (range). | | |
25. | Returns the next index of a character from the chars string | | |
26. | Finds the first index within a String, handling null. | | |
27. | Finds the last index within a String from a start position, handling null. | | |
28. | Finds the n-th index within a String, handling null. | | |
29. | Case insensitive check if a String ends with a specified suffix. | | |
30. | Case insensitive check if a String starts with a specified prefix. | | |
31. | Case insensitive removal of a substring if it is at the begining of a source string, otherwise returns the source string. | | |
32. | Case insensitive removal of a substring if it is at the end of a source string, otherwise returns the source string. | | |
33. | Check if a String ends with a specified suffix. | | |
34. | Check if a String starts with a specified prefix. | | |
35. | Determine if a String is contained in a String Collection | | |
36. | Determine if a String is contained in a String Collection, ignoring case | | |
37. | Determine if a String is contained in a String [], ignoring case | | |
38. | Determine if a String is contained in a String [], ignoring case or not as specified | | |
39. | Determine if a String is contained in a String[] | | |
40. | Determines if the specified string contains only Unicode letters or digits as defined by Character#isLetterOrDigit(char) | | |
41. | Determining the validity of various XML names | | |
42. | Return the nth index of the given token occurring in the given string | | |
43. | Find the earliest index of any of a set of potential substrings. | | |
44. | Find the latest index of any of a set of potential substrings. | | |
45. | Fast String Search | | |
46. | Count match | | |