Search a String to find the first index of any character in the given set of characters.
/**
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
/**
* <p>Operations on {@link java.lang.String} that are
* <code>null</code> safe.</p>
*
* @see java.lang.String
* @author <a href="http://jakarta.apache.org/turbine/">Apache Jakarta Turbine</a>
* @author <a href="mailto:jon@latchkey.com">Jon S. Stevens</a>
* @author Daniel L. Rall
* @author <a href="mailto:gcoladonato@yahoo.com">Greg Coladonato</a>
* @author <a href="mailto:ed@apache.org">Ed Korthof</a>
* @author <a href="mailto:rand_mcneely@yahoo.com">Rand McNeely</a>
* @author Stephen Colebourne
* @author <a href="mailto:fredrik@westermarck.com">Fredrik Westermarck</a>
* @author Holger Krauth
* @author <a href="mailto:alex@purpletech.com">Alexander Day Chaffee</a>
* @author <a href="mailto:hps@intermeta.de">Henning P. Schmiedehausen</a>
* @author Arun Mammen Thomas
* @author Gary Gregory
* @author Phil Steitz
* @author Al Chou
* @author Michael Davey
* @author Reuben Sivan
* @author Chris Hyzer
* @author Scott Johnson
* @since 1.0
* @version $Id: StringUtils.java 635447 2008-03-10 06:27:09Z bayard $
*/
public class Main {
// IndexOfAny chars
//-----------------------------------------------------------------------
/**
* <p>Search a String to find the first index of any
* character in the given set of characters.</p>
*
* <p>A <code>null</code> String will return <code>-1</code>.
* A <code>null</code> or zero length search array will return <code>-1</code>.</p>
*
* <pre>
* StringUtils.indexOfAny(null, *) = -1
* StringUtils.indexOfAny("", *) = -1
* StringUtils.indexOfAny(*, null) = -1
* StringUtils.indexOfAny(*, []) = -1
* StringUtils.indexOfAny("zzabyycdxx",['z','a']) = 0
* StringUtils.indexOfAny("zzabyycdxx",['b','y']) = 3
* StringUtils.indexOfAny("aba", ['z']) = -1
* </pre>
*
* @param str the String to check, may be null
* @param searchChars the chars to search for, may be null
* @return the index of any of the chars, -1 if no match or null input
* @since 2.0
*/
public static int indexOfAny(String str, char[] searchChars) {
if (isEmpty(str) || isEmpty(searchChars)) {
return -1;
}
for (int i = 0; i < str.length(); i++) {
char ch = str.charAt(i);
for (int j = 0; j < searchChars.length; j++) {
if (searchChars[j] == ch) {
return i;
}
}
}
return -1;
}
// ----------------------------------------------------------------------
/**
* <p>Checks if an array of Objects is empty or <code>null</code>.</p>
*
* @param array the array to test
* @return <code>true</code> if the array is empty or <code>null</code>
* @since 2.1
*/
public static boolean isEmpty(char[] array) {
if (array == null || array.length == 0) {
return true;
}
return false;
}
// Empty checks
//-----------------------------------------------------------------------
/**
* <p>Checks if a String is empty ("") or null.</p>
*
* <pre>
* StringUtils.isEmpty(null) = true
* StringUtils.isEmpty("") = true
* StringUtils.isEmpty(" ") = false
* StringUtils.isEmpty("bob") = false
* StringUtils.isEmpty(" bob ") = false
* </pre>
*
* <p>NOTE: This method changed in Lang version 2.0.
* It no longer trims the String.
* That functionality is available in isBlank().</p>
*
* @param str the String to check, may be null
* @return <code>true</code> if the String is empty or null
*/
public static boolean isEmpty(String str) {
return str == null || str.length() == 0;
}
}
Related examples in the same category
1. | String Region Match Demo | |  |
2. | Palindrome | | 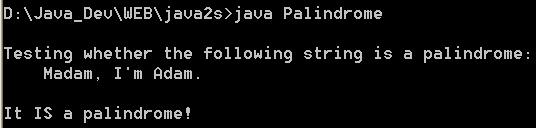 |
3. | Look for particular sequences in sentences | | 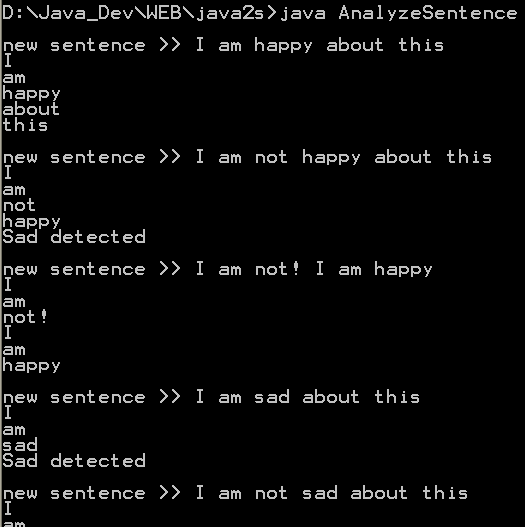 |
4. | Strings -- extract printable strings from binary file | | |
5. | Java Search String | | |
6. | Java String endsWith | | |
7. | Java String startsWith | | |
8. | Search a substring Anywhere | | |
9. | Starts with, ignore case( regular expressions ) | | |
10. | Ends with, ignore case( regular expressions ) | | |
11. | Anywhere, ignore case( regular expressions ) | | |
12. | Searching a String for a Character or a Substring | | |
13. | Not found returns -1 | | |
14. | If a string contains a specific word | | |
15. | Not found | | |
16. | if a String starts with a digit or uppercase letter | | |
17. | Search a String to find the first index of any character not in the given set of characters. | | |
18. | Searches a String for substrings delimited by a start and end tag, returning all matching substrings in an array. | | |
19. | Helper functions to query a strings end portion. The comparison is case insensitive. | | |
20. | Helper functions to query a strings start portion. The comparison is case insensitive. | | |
21. | Wrapper for arrays of ordered strings. This verifies the arrays and supports efficient lookups. | | |
22. | Returns an index into arra (or -1) where the character is not in the charset byte array. | | |
23. | Returns an int[] array of length segments containing the distribution count of the elements in unsorted int[] array with values between min and max (range). | | |
24. | Returns the next index of a character from the chars string | | |
25. | Finds the first index within a String, handling null. | | |
26. | Finds the last index within a String from a start position, handling null. | | |
27. | Finds the n-th index within a String, handling null. | | |
28. | Case insensitive check if a String ends with a specified suffix. | | |
29. | Case insensitive check if a String starts with a specified prefix. | | |
30. | Case insensitive removal of a substring if it is at the begining of a source string, otherwise returns the source string. | | |
31. | Case insensitive removal of a substring if it is at the end of a source string, otherwise returns the source string. | | |
32. | Check if a String ends with a specified suffix. | | |
33. | Check if a String starts with a specified prefix. | | |
34. | Determine if a String is contained in a String Collection | | |
35. | Determine if a String is contained in a String Collection, ignoring case | | |
36. | Determine if a String is contained in a String [], ignoring case | | |
37. | Determine if a String is contained in a String [], ignoring case or not as specified | | |
38. | Determine if a String is contained in a String[] | | |
39. | Determines if the specified string contains only Unicode letters or digits as defined by Character#isLetterOrDigit(char) | | |
40. | Determining the validity of various XML names | | |
41. | Return the nth index of the given token occurring in the given string | | |
42. | Find the earliest index of any of a set of potential substrings. | | |
43. | Find the latest index of any of a set of potential substrings. | | |
44. | Fast String Search | | |
45. | Performs continuous matching of a pattern in a given string. | | |
46. | Count match | | |