Communicating with the System Clipboard
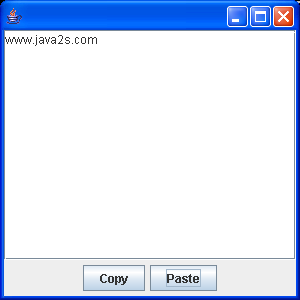
import java.awt.BorderLayout;
import java.awt.Container;
import java.awt.datatransfer.Clipboard;
import java.awt.datatransfer.DataFlavor;
import java.awt.datatransfer.StringSelection;
import java.awt.datatransfer.Transferable;
import java.awt.datatransfer.UnsupportedFlavorException;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.IOException;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
public class ClipText {
public static void main(String args[]) {
JFrame frame = new JFrame();
Container contentPane = frame.getContentPane();
final Clipboard clipboard = frame.getToolkit().getSystemClipboard();
final JTextArea jt = new JTextArea();
JScrollPane pane = new JScrollPane(jt);
contentPane.add(pane, BorderLayout.CENTER);
JButton copy = new JButton("Copy");
copy.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String selection = jt.getSelectedText();
StringSelection data = new StringSelection(selection);
clipboard.setContents(data, data);
}
});
JButton paste = new JButton("Paste");
paste.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent actionEvent) {
Transferable clipData = clipboard.getContents(clipboard);
if (clipData != null) {
try {
if (clipData
.isDataFlavorSupported(DataFlavor.stringFlavor)) {
String s = (String) (clipData
.getTransferData(DataFlavor.stringFlavor));
jt.replaceSelection(s);
}
} catch (UnsupportedFlavorException ufe) {
System.err.println("Unsupported flavor: " + ufe);
} catch (IOException ufe) {
System.err.println("Unable to get data: " + ufe);
}
}
}
});
JPanel p = new JPanel();
p.add(copy);
p.add(paste);
contentPane.add(p, BorderLayout.SOUTH);
frame.setSize(300, 300);
frame.show();
}
}
Related examples in the same category