Taken from the Sun documentation on Clipboard API
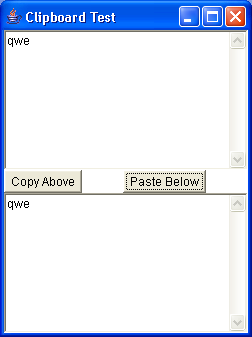
/*
* Copyright (c) Ian F. Darwin, http://www.darwinsys.com/, 1996-2002.
* All rights reserved. Software written by Ian F. Darwin and others.
* $Id: LICENSE,v 1.8 2004/02/09 03:33:38 ian Exp $
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
* 1. Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
*
* THIS SOFTWARE IS PROVIDED BY THE AUTHOR AND CONTRIBUTORS ``AS IS''
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED
* TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
* PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE AUTHOR OR CONTRIBUTORS
* BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* Java, the Duke mascot, and all variants of Sun's Java "steaming coffee
* cup" logo are trademarks of Sun Microsystems. Sun's, and James Gosling's,
* pioneering role in inventing and promulgating (and standardizing) the Java
* language and environment is gratefully acknowledged.
*
* The pioneering role of Dennis Ritchie and Bjarne Stroustrup, of AT&T, for
* inventing predecessor languages C and C++ is also gratefully acknowledged.
*/
import java.awt.Button;
import java.awt.Frame;
import java.awt.GridBagConstraints;
import java.awt.GridBagLayout;
import java.awt.TextArea;
import java.awt.datatransfer.Clipboard;
import java.awt.datatransfer.ClipboardOwner;
import java.awt.datatransfer.DataFlavor;
import java.awt.datatransfer.StringSelection;
import java.awt.datatransfer.Transferable;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
/** Taken from the Sun documentation on Clipboard API */
public class ClipboardTest extends Frame implements ClipboardOwner,
ActionListener {
TextArea srcText, dstText;
Button copyButton, pasteButton;
Clipboard clipboard = getToolkit().getSystemClipboard();
public ClipboardTest() {
super("Clipboard Test");
GridBagLayout gridbag = new GridBagLayout();
GridBagConstraints c = new GridBagConstraints();
setLayout(gridbag);
srcText = new TextArea(8, 32);
c.gridwidth = 2;
c.anchor = GridBagConstraints.CENTER;
gridbag.setConstraints(srcText, c);
add(srcText);
copyButton = new Button("Copy Above");
copyButton.setActionCommand("copy");
copyButton.addActionListener(this);
c.gridy = 1;
c.gridwidth = 1;
gridbag.setConstraints(copyButton, c);
add(copyButton);
pasteButton = new Button("Paste Below");
pasteButton.setActionCommand("paste");
pasteButton.addActionListener(this);
pasteButton.setEnabled(false);
c.gridx = 1;
gridbag.setConstraints(pasteButton, c);
add(pasteButton);
dstText = new TextArea(8, 32);
c.gridx = 0;
c.gridy = 2;
c.gridwidth = 2;
gridbag.setConstraints(dstText, c);
add(dstText);
pack();
}
public void actionPerformed(ActionEvent evt) {
String cmd = evt.getActionCommand();
if (cmd.equals("copy")) {
// Implement Copy operation
String srcData = srcText.getText();
if (srcData != null) {
StringSelection contents = new StringSelection(srcData);
clipboard.setContents(contents, this);
pasteButton.setEnabled(true);
}
} else if (cmd.equals("paste")) {
// Implement Paste operation
Transferable content = clipboard.getContents(this);
if (content != null) {
try {
String dstData = (String) content
.getTransferData(DataFlavor.stringFlavor);
dstText.append(dstData);
} catch (Exception e) {
System.out
.println("Couldn't get contents in format: "
+ DataFlavor.stringFlavor
.getHumanPresentableName());
}
}
}
}
public void lostOwnership(Clipboard clipboard, Transferable contents) {
System.out.println("Clipboard contents replaced");
}
public static void main(String[] args) {
ClipboardTest test = new ClipboardTest();
test.setVisible(true);
}
}
Related examples in the same category