Link the table and form fields (Smart GWT)
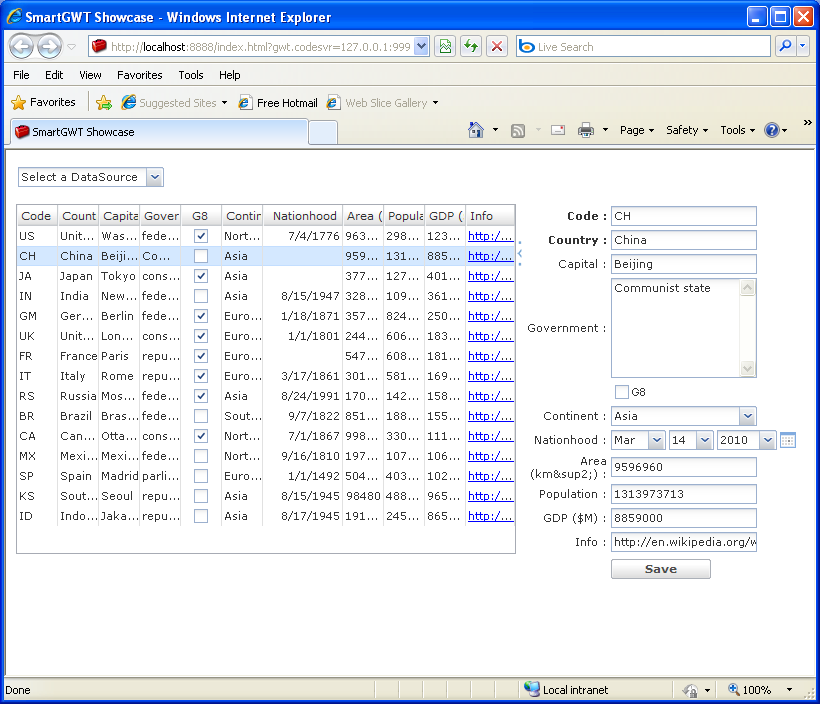
/*
* SmartGWT (GWT for SmartClient)
* Copyright 2008 and beyond, Isomorphic Software, Inc.
*
* SmartGWT is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License version 3
* as published by the Free Software Foundation. SmartGWT is also
* available under typical commercial license terms - see
* http://smartclient.com/license
* This software is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*/
package com.smartgwt.sample.showcase.client;
import com.google.gwt.core.client.EntryPoint;
import com.google.gwt.user.client.ui.Composite;
import com.google.gwt.user.client.ui.RootPanel;
import com.smartgwt.client.data.DataSource;
import com.smartgwt.client.data.fields.DataSourceBooleanField;
import com.smartgwt.client.data.fields.DataSourceDateField;
import com.smartgwt.client.data.fields.DataSourceFloatField;
import com.smartgwt.client.data.fields.DataSourceIntegerField;
import com.smartgwt.client.data.fields.DataSourceLinkField;
import com.smartgwt.client.data.fields.DataSourceTextField;
import com.smartgwt.client.types.Alignment;
import com.smartgwt.client.widgets.Canvas;
import com.smartgwt.client.widgets.IButton;
import com.smartgwt.client.widgets.events.ClickEvent;
import com.smartgwt.client.widgets.events.ClickHandler;
import com.smartgwt.client.widgets.form.DynamicForm;
import com.smartgwt.client.widgets.form.fields.SelectItem;
import com.smartgwt.client.widgets.form.fields.events.ChangedEvent;
import com.smartgwt.client.widgets.form.fields.events.ChangedHandler;
import com.smartgwt.client.widgets.grid.ListGrid;
import com.smartgwt.client.widgets.grid.events.RecordClickEvent;
import com.smartgwt.client.widgets.grid.events.RecordClickHandler;
import com.smartgwt.client.widgets.layout.HLayout;
import com.smartgwt.client.widgets.layout.VLayout;
public class Showcase implements EntryPoint {
public void onModuleLoad() {
RootPanel.get().add(getViewPanel());
}
public Canvas getViewPanel() {
final DataSource countryDS = CountryXmlDS.getInstance();
final DataSource supplyItemDS = SupplyCategoryXmlDS.getInstance();
final CompoundEditor cEditor = new CompoundEditor(countryDS);
SelectItem dsSelect = new SelectItem();
dsSelect.setName("datasource2");
dsSelect.setShowTitle(false);
dsSelect.setValueMap("country", "supply");
dsSelect.addChangedHandler(new ChangedHandler() {
public void onChanged(ChangedEvent event) {
String ds = (String) event.getValue();
if (ds.equalsIgnoreCase("country")) {
cEditor.setDatasource(countryDS);
} else {
cEditor.setDatasource(supplyItemDS);
}
}
});
DynamicForm form = new DynamicForm();
form.setValue("datasource2", "Select a DataSource");
form.setItems(dsSelect);
VLayout layout = new VLayout(15);
layout.addMember(form);
layout.addMember(cEditor);
return layout;
}
private static class CompoundEditor extends Composite {
private DataSource datasource;
private DynamicForm form;
private ListGrid grid;
private IButton saveButton;
public CompoundEditor(DataSource datasource) {
super();
this.datasource = datasource;
this.form = new DynamicForm();
form.setDataSource(datasource);
saveButton = new IButton("Save");
saveButton.setLayoutAlign(Alignment.CENTER);
saveButton.addClickHandler(new ClickHandler() {
public void onClick(ClickEvent event) {
form.saveData();
}
});
VLayout editorLayout = new VLayout(5);
editorLayout.addMember(form);
editorLayout.addMember(saveButton);
grid = new ListGrid();
grid.setWidth(500);
grid.setHeight(350);
grid.setDataSource(datasource);
grid.setShowResizeBar(true);
grid.setAutoFetchData(true);
grid.addRecordClickHandler(new RecordClickHandler() {
public void onRecordClick(RecordClickEvent event) {
form.clearErrors(true);
form.editRecord(event.getRecord());
saveButton.enable();
}
});
HLayout hLayout = new HLayout();
hLayout.setAutoWidth();
hLayout.addMember(grid);
hLayout.addMember(editorLayout);
// All composites must call initWidget() in their constructors.
initWidget(hLayout);
}
public DataSource getDatasource() {
return datasource;
}
public void setDatasource(DataSource datasource) {
this.datasource = datasource;
grid.setDataSource(datasource);
form.setDataSource(datasource);
saveButton.disable();
grid.fetchData();
}
}
}
class SupplyCategoryXmlDS extends DataSource {
private static SupplyCategoryXmlDS instance = null;
public static SupplyCategoryXmlDS getInstance() {
if (instance == null) {
instance = new SupplyCategoryXmlDS("supplyCategoryDS");
}
return instance;
}
public SupplyCategoryXmlDS(String id) {
setID(id);
setRecordXPath("/List/supplyCategory");
DataSourceTextField itemNameField = new DataSourceTextField("categoryName", "Item", 128, true);
itemNameField.setPrimaryKey(true);
DataSourceTextField parentField = new DataSourceTextField("parentID", null);
parentField.setHidden(true);
parentField.setRequired(true);
parentField.setRootValue("root");
parentField.setForeignKey("supplyCategoryDS.categoryName");
setFields(itemNameField, parentField);
setDataURL("ds/test_data/supplyCategory.data.xml");
setClientOnly(true);
}
}
class CountryXmlDS extends DataSource {
private static CountryXmlDS instance = null;
public static CountryXmlDS getInstance() {
if (instance == null) {
instance = new CountryXmlDS("countryDS");
}
return instance;
}
public CountryXmlDS(String id) {
setID(id);
setRecordXPath("/List/country");
DataSourceIntegerField pkField = new DataSourceIntegerField("pk");
pkField.setHidden(true);
pkField.setPrimaryKey(true);
DataSourceTextField countryCodeField = new DataSourceTextField("countryCode", "Code");
countryCodeField.setRequired(true);
DataSourceTextField countryNameField = new DataSourceTextField("countryName", "Country");
countryNameField.setRequired(true);
DataSourceTextField capitalField = new DataSourceTextField("capital", "Capital");
DataSourceTextField governmentField = new DataSourceTextField("government", "Government", 500);
DataSourceBooleanField memberG8Field = new DataSourceBooleanField("member_g8", "G8");
DataSourceTextField continentField = new DataSourceTextField("continent", "Continent");
continentField.setValueMap("Europe", "Asia", "North America", "Australia/Oceania",
"South America", "Africa");
DataSourceDateField independenceField = new DataSourceDateField("independence", "Nationhood");
DataSourceFloatField areaField = new DataSourceFloatField("area", "Area (km²)");
DataSourceIntegerField populationField = new DataSourceIntegerField("population", "Population");
DataSourceFloatField gdpField = new DataSourceFloatField("gdp", "GDP ($M)");
DataSourceLinkField articleField = new DataSourceLinkField("article", "Info");
setFields(pkField, countryCodeField, countryNameField, capitalField, governmentField,
memberG8Field, continentField, independenceField, areaField, populationField, gdpField,
articleField);
setDataURL("ds/test_data/country.data.xml");
setClientOnly(true);
}
}
SmartGWT.zip( 9,880 k)Related examples in the same category