Tree, table and detail panel (Smart GWT)
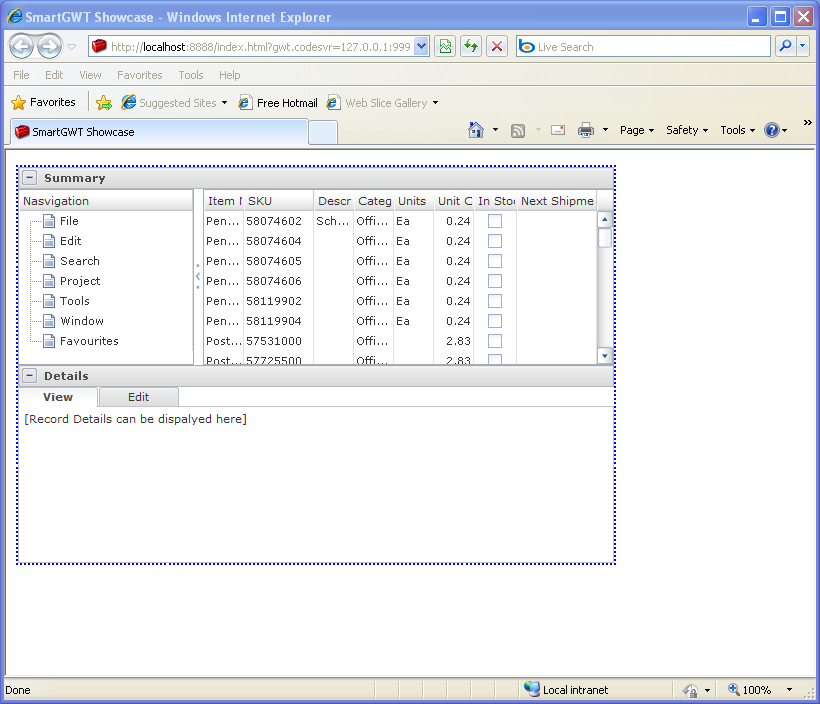
/*
* SmartGWT (GWT for SmartClient)
* Copyright 2008 and beyond, Isomorphic Software, Inc.
*
* SmartGWT is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License version 3
* as published by the Free Software Foundation. SmartGWT is also
* available under typical commercial license terms - see
* http://smartclient.com/license
* This software is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*/
package com.smartgwt.sample.showcase.client;
import com.google.gwt.core.client.EntryPoint;
import com.google.gwt.user.client.ui.RootPanel;
import com.smartgwt.client.data.DataSource;
import com.smartgwt.client.data.fields.DataSourceBooleanField;
import com.smartgwt.client.data.fields.DataSourceDateField;
import com.smartgwt.client.data.fields.DataSourceEnumField;
import com.smartgwt.client.data.fields.DataSourceFloatField;
import com.smartgwt.client.data.fields.DataSourceIntegerField;
import com.smartgwt.client.data.fields.DataSourceLinkField;
import com.smartgwt.client.data.fields.DataSourceTextField;
import com.smartgwt.client.types.Overflow;
import com.smartgwt.client.types.TreeModelType;
import com.smartgwt.client.types.VisibilityMode;
import com.smartgwt.client.widgets.Canvas;
import com.smartgwt.client.widgets.form.validator.FloatPrecisionValidator;
import com.smartgwt.client.widgets.form.validator.FloatRangeValidator;
import com.smartgwt.client.widgets.grid.CellFormatter;
import com.smartgwt.client.widgets.grid.ListGrid;
import com.smartgwt.client.widgets.grid.ListGridRecord;
import com.smartgwt.client.widgets.layout.HLayout;
import com.smartgwt.client.widgets.layout.SectionStack;
import com.smartgwt.client.widgets.layout.SectionStackSection;
import com.smartgwt.client.widgets.tab.Tab;
import com.smartgwt.client.widgets.tab.TabSet;
import com.smartgwt.client.widgets.tree.Tree;
import com.smartgwt.client.widgets.tree.TreeGrid;
import com.smartgwt.client.widgets.tree.TreeGridField;
import com.smartgwt.client.widgets.tree.TreeNode;
public class Showcase implements EntryPoint {
public void onModuleLoad() {
RootPanel.get().add(getViewPanel());
}
public Canvas getViewPanel() {
DataSource dataSource = ItemSupplyXmlDS.getInstance();
final ListGrid listGrid = new ListGrid();
listGrid.setUseAllDataSourceFields(true);
listGrid.setDataSource(dataSource);
listGrid.setAutoFetchData(true);
TabSet tabSet = new TabSet();
Tab viewTab = new Tab("View");
Canvas viewLabel = new Canvas();
viewLabel.setContents("[Record Details can be dispalyed here]");
viewTab.setPane(viewLabel);
Tab editTab = new Tab("Edit");
Canvas editLabel = new Canvas();
editLabel.setContents("[Form for edits can be dispalyed here]");
editTab.setPane(editLabel);
tabSet.setTabs(viewTab, editTab);
TreeGrid treeGrid = new TreeGrid();
treeGrid.setWidth("30%");
treeGrid.setShowConnectors(true);
treeGrid.setShowResizeBar(true);
Tree data = new Tree();
data.setModelType(TreeModelType.CHILDREN);
data.setRoot(new TreeNode("root", new TreeNode("File"), new TreeNode("Edit"), new TreeNode(
"Search"), new TreeNode("Project"), new TreeNode("Tools"), new TreeNode("Window"),
new TreeNode("Favourites")));
treeGrid.setData(data);
TreeGridField field = new TreeGridField("Nasvigation");
field.setCellFormatter(new CellFormatter() {
public String format(Object value, ListGridRecord record, int rowNum, int colNum) {
return record.getAttribute("name");
}
});
treeGrid.setFields(field);
HLayout navLayout = new HLayout();
navLayout.setMembers(treeGrid, listGrid);
SectionStack sectionStack = new SectionStack();
sectionStack.setBorder("2px dotted blue");
sectionStack.setWidth(600);
sectionStack.setHeight(400);
sectionStack.setVisibilityMode(VisibilityMode.MULTIPLE);
sectionStack.setAnimateSections(true);
sectionStack.setOverflow(Overflow.HIDDEN);
SectionStackSection summarySection = new SectionStackSection();
summarySection.setTitle("Summary");
summarySection.setExpanded(true);
summarySection.setItems(navLayout);
SectionStackSection detailsSection = new SectionStackSection();
detailsSection.setTitle("Details");
detailsSection.setExpanded(true);
detailsSection.setItems(tabSet);
sectionStack.setSections(summarySection, detailsSection);
return sectionStack;
}
}
class SupplyCategoryXmlDS extends DataSource {
private static SupplyCategoryXmlDS instance = null;
public static SupplyCategoryXmlDS getInstance() {
if (instance == null) {
instance = new SupplyCategoryXmlDS("supplyCategoryDS");
}
return instance;
}
public SupplyCategoryXmlDS(String id) {
setID(id);
setRecordXPath("/List/supplyCategory");
DataSourceTextField itemNameField = new DataSourceTextField("categoryName", "Item", 128, true);
itemNameField.setPrimaryKey(true);
DataSourceTextField parentField = new DataSourceTextField("parentID", null);
parentField.setHidden(true);
parentField.setRequired(true);
parentField.setRootValue("root");
parentField.setForeignKey("supplyCategoryDS.categoryName");
setFields(itemNameField, parentField);
setDataURL("ds/test_data/supplyCategory.data.xml");
setClientOnly(true);
}
}
class ItemSupplyXmlDS extends DataSource {
private static ItemSupplyXmlDS instance = null;
public static ItemSupplyXmlDS getInstance() {
if (instance == null) {
instance = new ItemSupplyXmlDS("supplyItemDS");
}
return instance;
}
public ItemSupplyXmlDS(String id) {
setID(id);
setRecordXPath("/List/supplyItem");
DataSourceIntegerField pkField = new DataSourceIntegerField("itemID");
pkField.setHidden(true);
pkField.setPrimaryKey(true);
DataSourceTextField itemNameField = new DataSourceTextField("itemName", "Item Name", 128, true);
DataSourceTextField skuField = new DataSourceTextField("SKU", "SKU", 10, true);
DataSourceTextField descriptionField = new DataSourceTextField("description", "Description",
2000);
DataSourceTextField categoryField = new DataSourceTextField("category", "Category", 128, true);
categoryField.setForeignKey("supplyCategoryDS.categoryName");
DataSourceEnumField unitsField = new DataSourceEnumField("units", "Units", 5);
unitsField.setValueMap("Roll", "Ea", "Pkt", "Set", "Tube", "Pad", "Ream", "Tin", "Bag", "Ctn",
"Box");
DataSourceFloatField unitCostField = new DataSourceFloatField("unitCost", "Unit Cost", 5);
FloatRangeValidator rangeValidator = new FloatRangeValidator();
rangeValidator.setMin(0);
rangeValidator.setErrorMessage("Please enter a valid (positive) cost");
FloatPrecisionValidator precisionValidator = new FloatPrecisionValidator();
precisionValidator.setPrecision(2);
precisionValidator.setErrorMessage("The maximum allowed precision is 2");
unitCostField.setValidators(rangeValidator, precisionValidator);
DataSourceBooleanField inStockField = new DataSourceBooleanField("inStock", "In Stock");
DataSourceDateField nextShipmentField = new DataSourceDateField("nextShipment", "Next Shipment");
setFields(pkField, itemNameField, skuField, descriptionField, categoryField, unitsField,
unitCostField, inStockField, nextShipmentField);
setDataURL("ds/test_data/supplyItem.data.xml");
setClientOnly(true);
}
}
class CountryXmlDS extends DataSource {
private static CountryXmlDS instance = null;
public static CountryXmlDS getInstance() {
if (instance == null) {
instance = new CountryXmlDS("countryDS");
}
return instance;
}
public CountryXmlDS(String id) {
setID(id);
setRecordXPath("/List/country");
DataSourceIntegerField pkField = new DataSourceIntegerField("pk");
pkField.setHidden(true);
pkField.setPrimaryKey(true);
DataSourceTextField countryCodeField = new DataSourceTextField("countryCode", "Code");
countryCodeField.setRequired(true);
DataSourceTextField countryNameField = new DataSourceTextField("countryName", "Country");
countryNameField.setRequired(true);
DataSourceTextField capitalField = new DataSourceTextField("capital", "Capital");
DataSourceTextField governmentField = new DataSourceTextField("government", "Government", 500);
DataSourceBooleanField memberG8Field = new DataSourceBooleanField("member_g8", "G8");
DataSourceTextField continentField = new DataSourceTextField("continent", "Continent");
continentField.setValueMap("Europe", "Asia", "North America", "Australia/Oceania",
"South America", "Africa");
DataSourceDateField independenceField = new DataSourceDateField("independence", "Nationhood");
DataSourceFloatField areaField = new DataSourceFloatField("area", "Area (km²)");
DataSourceIntegerField populationField = new DataSourceIntegerField("population", "Population");
DataSourceFloatField gdpField = new DataSourceFloatField("gdp", "GDP ($M)");
DataSourceLinkField articleField = new DataSourceLinkField("article", "Info");
setFields(pkField, countryCodeField, countryNameField, capitalField, governmentField,
memberG8Field, continentField, independenceField, areaField, populationField, gdpField,
articleField);
setDataURL("ds/test_data/country.data.xml");
setClientOnly(true);
}
}
SmartGWT.zip( 9,880 k)Related examples in the same category