Tree with Multiple Columns Sample (Smart GWT)
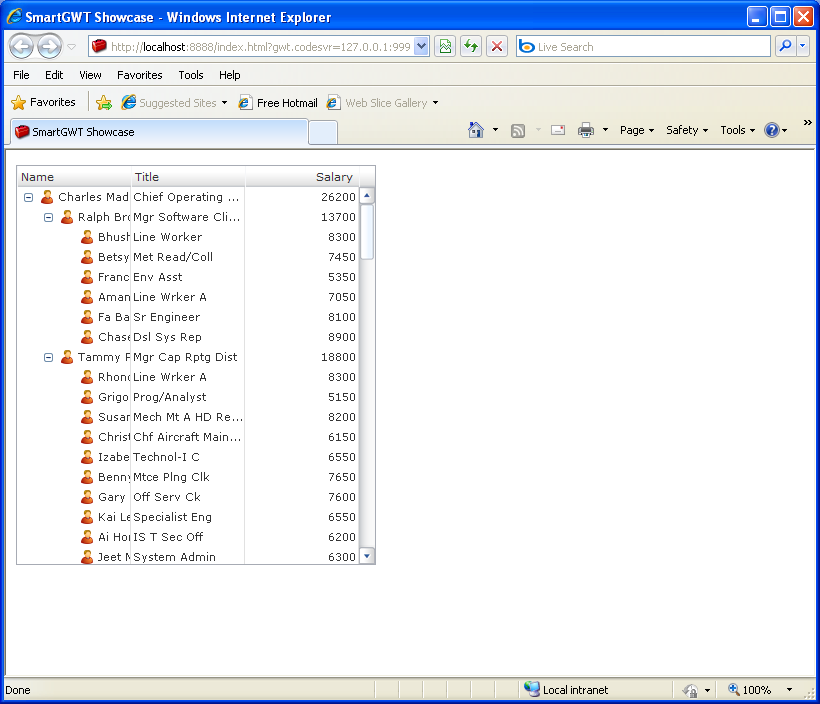
/*
* SmartGWT (GWT for SmartClient)
* Copyright 2008 and beyond, Isomorphic Software, Inc.
*
* SmartGWT is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License version 3
* as published by the Free Software Foundation. SmartGWT is also
* available under typical commercial license terms - see
* http://smartclient.com/license
* This software is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*/
package com.smartgwt.sample.showcase.client;
import com.google.gwt.core.client.EntryPoint;
import com.google.gwt.user.client.ui.RootPanel;
import com.smartgwt.client.data.DataSource;
import com.smartgwt.client.data.fields.DataSourceFloatField;
import com.smartgwt.client.data.fields.DataSourceIntegerField;
import com.smartgwt.client.data.fields.DataSourceTextField;
import com.smartgwt.client.widgets.Canvas;
import com.smartgwt.client.widgets.tree.TreeGrid;
import com.smartgwt.client.widgets.tree.TreeGridField;
import com.smartgwt.client.widgets.tree.events.DataArrivedEvent;
import com.smartgwt.client.widgets.tree.events.DataArrivedHandler;
public class Showcase implements EntryPoint {
public void onModuleLoad() {
RootPanel.get().add(getViewPanel());
}
public Canvas getViewPanel() {
EmployeeXmlDS employeesDS = EmployeeXmlDS.getInstance();
final TreeGrid treeGrid = new TreeGrid();
treeGrid.setCanEdit(true);
treeGrid.setLoadDataOnDemand(false);
treeGrid.setWidth(360);
treeGrid.setHeight(400);
treeGrid.setDataSource(employeesDS);
treeGrid.setNodeIcon("icons/16/person.png");
treeGrid.setFolderIcon("icons/16/person.png");
treeGrid.setShowOpenIcons(false);
treeGrid.setShowDropIcons(false);
treeGrid.setClosedIconSuffix("");
treeGrid.setAutoFetchData(true);
TreeGridField nameField = new TreeGridField("Name");
TreeGridField jobField = new TreeGridField("Job");
TreeGridField salaryField = new TreeGridField("Salary");
treeGrid.setFields(nameField, jobField, salaryField);
treeGrid.addDataArrivedHandler(new DataArrivedHandler() {
public void onDataArrived(DataArrivedEvent event) {
treeGrid.getData().openAll();
}
});
return treeGrid;
}
}
class EmployeeXmlDS extends DataSource {
private static EmployeeXmlDS instance = null;
public static EmployeeXmlDS getInstance() {
if (instance == null) {
instance = new EmployeeXmlDS("employeesDS");
}
return instance;
}
public EmployeeXmlDS(String id) {
setID(id);
setTitleField("Name");
setRecordXPath("/List/employee");
DataSourceTextField nameField = new DataSourceTextField("Name", "Name", 128);
DataSourceIntegerField employeeIdField = new DataSourceIntegerField("EmployeeId", "Employee ID");
employeeIdField.setPrimaryKey(true);
employeeIdField.setRequired(true);
DataSourceIntegerField reportsToField = new DataSourceIntegerField("ReportsTo", "Manager");
reportsToField.setRequired(true);
reportsToField.setForeignKey(id + ".EmployeeId");
reportsToField.setRootValue("1");
DataSourceTextField jobField = new DataSourceTextField("Job", "Title", 128);
DataSourceTextField emailField = new DataSourceTextField("Email", "Email", 128);
DataSourceTextField statusField = new DataSourceTextField("EmployeeStatus", "Status", 40);
DataSourceFloatField salaryField = new DataSourceFloatField("Salary", "Salary");
DataSourceTextField orgField = new DataSourceTextField("OrgUnit", "Org Unit", 128);
DataSourceTextField genderField = new DataSourceTextField("Gender", "Gender", 7);
genderField.setValueMap("male", "female");
DataSourceTextField maritalStatusField = new DataSourceTextField("MaritalStatus",
"Marital Status", 10);
setFields(nameField, employeeIdField, reportsToField, jobField, emailField, statusField,
salaryField, orgField, genderField, maritalStatusField);
setDataURL("ds/test_data/employees.data.xml");
setClientOnly(true);
}
}
SmartGWT.zip( 9,880 k)Related examples in the same category