Demonstrates simple record sorting and filtering
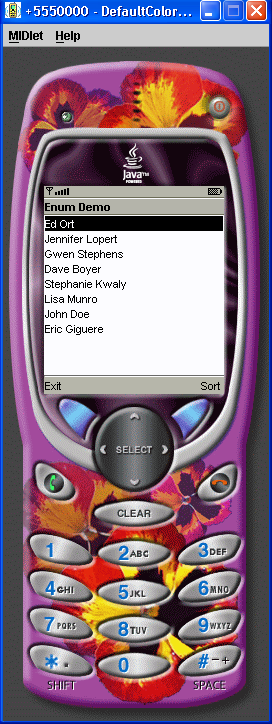
/* License
*
* Copyright 1994-2004 Sun Microsystems, Inc. All Rights Reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* * Redistribution of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
*
* * Redistribution in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
*
* Neither the name of Sun Microsystems, Inc. or the names of contributors
* may be used to endorse or promote products derived from this software
* without specific prior written permission.
*
* This software is provided "AS IS," without a warranty of any kind. ALL
* EXPRESS OR IMPLIED CONDITIONS, REPRESENTATIONS AND WARRANTIES, INCLUDING
* ANY IMPLIED WARRANTY OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE
* OR NON-INFRINGEMENT, ARE HEREBY EXCLUDED. SUN MICROSYSTEMS, INC. ("SUN")
* AND ITS LICENSORS SHALL NOT BE LIABLE FOR ANY DAMAGES SUFFERED BY LICENSEE
* AS A RESULT OF USING, MODIFYING OR DISTRIBUTING THIS SOFTWARE OR ITS
* DERIVATIVES. IN NO EVENT WILL SUN OR ITS LICENSORS BE LIABLE FOR ANY LOST
* REVENUE, PROFIT OR DATA, OR FOR DIRECT, INDIRECT, SPECIAL, CONSEQUENTIAL,
* INCIDENTAL OR PUNITIVE DAMAGES, HOWEVER CAUSED AND REGARDLESS OF THE THEORY
* OF LIABILITY, ARISING OUT OF THE USE OF OR INABILITY TO USE THIS SOFTWARE,
* EVEN IF SUN HAS BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES.
*
* You acknowledge that this software is not designed, licensed or intended
* for use in the design, construction, operation or maintenance of any
* nuclear facility.
*/
import java.io.*;
import javax.microedition.lcdui.*;
import javax.microedition.midlet.*;
import javax.microedition.rms.*;
// Demonstrates simple record sorting and filtering
public class EnumDemoMIDlet extends MIDlet
implements CommandListener {
// Data members we need
private Display display;
private RecordStore rs;
private EnumList enumListScreen;
private SortOptions sortOptionsScreen;
private String firstName;
private String lastName;
private byte[] data = new byte[200];
private ByteArrayInputStream bin =
new ByteArrayInputStream( data );
private DataInputStream din =
new DataInputStream( bin );
// Define the four commands we use
private Command exitCommand = new Command( "Exit",
Command.EXIT, 1 );
private Command sortCommand = new Command( "Sort",
Command.SCREEN, 1 );
private Command cancelCommand = new Command( "Cancel",
Command.CANCEL, 1 );
private Command okCommand = new Command( "OK",
Command.OK, 1 );
// Define the sorting modes
private static final int SORT_NONE = 0;
private static final int SORT_FIRST_LAST = 1;
private static final int SORT_LAST_FIRST = 2;
// Define the filtering modes
private static final int FILTER_NONE = 0;
private static final int FILTER_STARTSWITH = 1;
private static final int FILTER_CONTAINS = 2;
// A simple class to hold the parts of a record
private static class Record {
String firstName;
String lastName;
}
// Precanned names
private static final String[][] names = {
{ "Eric", "Giguere" },
{ "John", "Doe" },
{ "Lisa", "Munro" },
{ "Stephanie", "Kwaly" },
{ "Dave", "Boyer" },
{ "Gwen", "Stephens" },
{ "Jennifer", "Lopert" },
{ "Ed", "Ort" },
};
// Constructor
public EnumDemoMIDlet(){
}
// Clean up
protected void destroyApp( boolean unconditional )
throws MIDletStateChangeException {
exitMIDlet();
}
// Close the record store for now
protected void pauseApp(){
closeRecordStore();
}
// Initialize things and reopen record store
// if not open
protected void startApp() throws
MIDletStateChangeException {
if( display == null ){ // first time called...
initMIDlet();
}
if( rs == null ){
openRecordStore();
}
}
// Once-only initialization code
private void initMIDlet(){
display = Display.getDisplay( this );
enumListScreen = new EnumList();
sortOptionsScreen = new SortOptions();
if( openRecordStore() ){
enumListScreen.resort();
display.setCurrent( enumListScreen );
}
}
// Termination code
public void exitMIDlet(){
closeRecordStore();
notifyDestroyed();
}
// Add a first-last name pair to the record store
private void addName( String first, String last,
ByteArrayOutputStream bout,
DataOutputStream dout ){
try {
dout.writeUTF( first );
dout.writeUTF( last );
dout.flush();
byte[] data = bout.toByteArray();
rs.addRecord( data, 0, data.length );
bout.reset();
}
catch( Exception e ){
}
}
// Fill record store with some precanned names
private void fillRecordStore(){
ByteArrayOutputStream bout =
new ByteArrayOutputStream();
DataOutputStream dout =
new DataOutputStream( bout );
for( int i = 0; i < names.length; ++i ){
addName( names[i][0], names[i][1], bout,
dout );
}
}
// Open record store, if empty then fill it
private boolean openRecordStore(){
try {
if( rs != null ) closeRecordStore();
rs = RecordStore.openRecordStore(
"EnumDemo", true );
if( rs.getNumRecords() == 0 ){
fillRecordStore();
}
return true;
}
catch( RecordStoreException e ){
return false;
}
}
// Close record store
private void closeRecordStore(){
if( rs != null ){
try {
rs.closeRecordStore();
}
catch( RecordStoreException e ){
}
rs = null;
}
}
// Move to and read in a record
private boolean readRecord( int id, Record r ){
boolean ok = false;
r.firstName = null;
r.lastName = null;
if( rs != null ){
try {
rs.getRecord( id, data, 0 );
r.firstName = din.readUTF();
r.lastName = din.readUTF();
din.reset();
ok = true;
}
catch( Exception e ){
}
}
return ok;
}
// Event handling
public void commandAction( Command c, Displayable d ){
if( c == exitCommand ){
exitMIDlet();
} else if( c == sortCommand ){
display.setCurrent( sortOptionsScreen );
} else if( d == sortOptionsScreen ){
if( c == okCommand ){
enumListScreen.resort();
}
display.setCurrent( enumListScreen );
}
}
// Main screen -- a list of names
class EnumList extends List
implements RecordComparator,
RecordFilter {
private int sortBy;
private int filterBy;
private String filterText;
private Record r1 = new Record();
private Record r2 = new Record();
// Constructor
EnumList(){
super( "Enum Demo", IMPLICIT );
addCommand( exitCommand );
addCommand( sortCommand );
setCommandListener( EnumDemoMIDlet.this );
}
// Resort the data and refill the list
public void resort(){
sortBy = sortOptionsScreen.getSortType();
filterBy = sortOptionsScreen.getFilterType();
filterText = sortOptionsScreen.getFilterText();
RecordComparator comparator = null;
RecordFilter filter = null;
if( sortBy != 0 ){
comparator = this;
}
if( filterBy != 0 ){
filter = this;
}
deleteAll();
try {
RecordEnumeration e = rs.enumerateRecords(
filter, comparator, false );
Record r = new Record();
while( e.hasNextElement() ){
int id = e.nextRecordId();
if( readRecord( id, r ) ){
if( sortBy == SORT_LAST_FIRST ){
append( r.lastName + ", " +
r.firstName, null );
} else {
append( r.firstName + " " +
r.lastName, null );
}
}
}
e.destroy();
}
catch( RecordStoreException e ){
}
}
// Delete all items in the list
public void deleteAll(){
int n = size();
while( n > 0 ){
delete( --n );
}
}
// The comparator
public int compare( byte[] rec1, byte[] rec2 ){
try {
ByteArrayInputStream bin =
new ByteArrayInputStream( rec1 );
DataInputStream din =
new DataInputStream( bin );
r1.firstName = din.readUTF();
r1.lastName = din.readUTF();
bin = new ByteArrayInputStream( rec2 );
din = new DataInputStream( bin );
r2.firstName = din.readUTF();
r2.lastName = din.readUTF();
if( sortBy == SORT_FIRST_LAST ){
int cmp = r1.firstName.compareTo(
r2.firstName );
System.out.println( r1.firstName +
" compares to " + r2.firstName +
" gives " + cmp );
if( cmp != 0 ) return (
cmp < 0 ? PRECEDES : FOLLOWS );
cmp = r2.lastName.compareTo( r2.lastName );
if( cmp != 0 ) return (
cmp < 0 ? PRECEDES : FOLLOWS );
} else if( sortBy == SORT_LAST_FIRST ){
int cmp = r1.lastName.compareTo(
r2.lastName );
if( cmp != 0 ) return (
cmp < 0 ? PRECEDES : FOLLOWS );
cmp = r2.firstName.compareTo(
r2.firstName );
if( cmp != 0 ) return (
cmp < 0 ? PRECEDES :
FOLLOWS );
}
}
catch( Exception e ){
}
return EQUIVALENT;
}
// The filter
public boolean matches( byte[] rec ){
try {
ByteArrayInputStream bin =
new ByteArrayInputStream( rec );
DataInputStream din =
new DataInputStream( bin );
r1.firstName = din.readUTF();
r1.lastName = din.readUTF();
if( filterBy == FILTER_STARTSWITH ){
return( r1.firstName.startsWith(
filterText )
||
r1.lastName.startsWith(
filterText ) );
} else if( filterBy ==
FILTER_CONTAINS ){
return( r1.firstName.indexOf(
filterText )
>=0 ||
r1.lastName.indexOf(
filterText) >= 0);
}
}
catch( Exception e ){
}
return false;
}
}
// The options screen for choosing which sort and
// filter modes to use, including the filter text
class SortOptions extends Form {
// Constructor
SortOptions(){
super( "Sort Options" );
addCommand( okCommand );
addCommand( cancelCommand );
setCommandListener( EnumDemoMIDlet.this );
append( sortTypes );
append( filterTypes );
append( filterText );
}
// Return current sort type
public int getSortType(){
return sortTypes.getSelectedIndex();
}
// Return current filter type
public int getFilterType(){
return filterTypes.getSelectedIndex();
}
// Return current filter text
public String getFilterText(){
return filterText.getString();
}
// Labels and user interface components
private String[] sortLabels =
{ "None", "First Last",
"Last, First" };
private String[] filterLabels =
{ "None", "Starts With",
"Contains" };
private ChoiceGroup sortTypes =
new ChoiceGroup(
"Sort Type:",
ChoiceGroup.EXCLUSIVE,
sortLabels, null );
private ChoiceGroup filterTypes =
new ChoiceGroup(
"Filter Type:",
ChoiceGroup.EXCLUSIVE,
filterLabels, null );
private TextField filterText = new TextField(
"Filter Text:",
null, 20, 0 );
}
}
Related examples in the same category