Timer Template
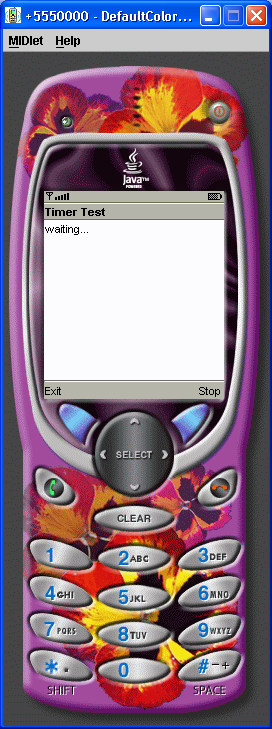
/*--------------------------------------------------
* TimerTemplate.java
*
* Test all six Timer scheduling options
*
* Example from the book: Core J2ME Technology
* Copyright John W. Muchow http://www.CoreJ2ME.com
* You may use/modify for any non-commercial purpose
*-------------------------------------------------*/
import java.util.*;
import javax.microedition.midlet.*;
import javax.microedition.lcdui.*;
public class TimerTemplate extends MIDlet implements CommandListener
{
private Display display; // Our display
private Form fmMain; // Main form
private Command cmExit; // Exit midlet
private Command cmStop; // Stop the timer
private Timer tm; // Timer
private TestTimerTask tt; // Task
private int count = 0; // How many times has task run
public TimerTemplate()
{
display = Display.getDisplay(this);
// Create main form
fmMain = new Form("Timer Test");
fmMain.append("waiting...\n");
// Create commands and add to form
cmExit = new Command("Exit", Command.EXIT, 1);
cmStop= new Command("Stop", Command.STOP, 2);
fmMain.addCommand(cmExit);
fmMain.addCommand(cmStop);
fmMain.setCommandListener(this);
//------------------------------------------------------
// Option #1 - One time task with delayed start
// Create a timer that will go off in 5 seconds
//------------------------------------------------------
tm = new Timer();
tt = new TestTimerTask();
tm.schedule(tt,5000);
//------------------------------------------------------
// Option #2 - Fixed-delay repeating task with delayed start
// Create a timer that will go off in 5 seconds
// Repeating every 3 seconds
//------------------------------------------------------
// tm = new Timer();
// tt = new TestTimerTask();
// tm.schedule(tt,5000, 3000);
//------------------------------------------------------
// Option #3 - Fixed-rate repeating task with delayed start
// Create a timer that will go off in 5 seconds
// Repeating every 3 seconds
//------------------------------------------------------
// timer = new Timer();
// tt = new TestTimerTask();
// timer.scheduleAtFixedRate(tt,5000, 3000);
//------------------------------------------------------
// Option #4 - One time task at specified date
// Create timer that starts at current date
//------------------------------------------------------
// timer = new Timer();
// tt = new TestTimerTask();
// timer.schedule(tt, new Date());
//------------------------------------------------------
// Option #5 - Fixed-delay repeating task starting
// at a specified date
// Create timer that starts at current date
// Repeating every 3 seconds
//------------------------------------------------------
// timer = new Timer();
// tt = new TestTimerTask();
// timer.schedule(tt, new Date(), 3000);
//------------------------------------------------------
// Option #6 - Fixed-rate repeating task starting
// at a specified date
// Create timer that starts at current date
// Repeating every 3 seconds
//------------------------------------------------------
// timer = new Timer();
// tt = new TestTimerTask();
// timer.scheduleAtFixedRate(tt, new Date(), 3000);
}
/*--------------------------------------------------
* Show the main Form
*-------------------------------------------------*/
public void startApp ()
{
display.setCurrent(fmMain);
}
/*--------------------------------------------------
* Shutting down. Cleanup all we created
*-------------------------------------------------*/
public void destroyApp (boolean unconditional)
{
}
/*--------------------------------------------------
* No pause code necessary
*-------------------------------------------------*/
public void pauseApp ()
{ }
/*--------------------------------------------------
* Process events for the main form
*-------------------------------------------------*/
public void commandAction(Command c, Displayable d)
{
if (c == cmStop)
{
tm.cancel();
}
else if (c == cmExit)
{
destroyApp(false);
notifyDestroyed();
}
}
/*--------------------------------------------------
* TestTimerTask Class - Run the task
*-------------------------------------------------*/
private class TestTimerTask extends TimerTask
{
public final void run()
{
fmMain.append("run count: " + ++count + "\n");
}
}
}
Related examples in the same category