Timer and sticker
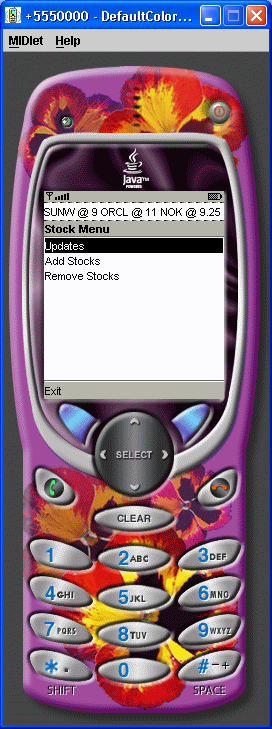
/*
* TimerMIDlet.java Copyright (c) 2000 Sun Microsystems, Inc. All Rights
* Reserved.
*
* Author: Srikanth Raju
*
* This software is the confidential and proprietary information of Sun
* Microsystems, Inc. ("Confidential Information"). You shall not disclose such
* Confidential Information and shall use it only in accordance with the terms
* of the license agreement you entered into with Sun.
*
* SUN MAKES NO REPRESENTATIONS OR WARRANTIES ABOUT THE SUITABILITY OF THE
* SOFTWARE, EITHER EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE IMPLIED
* WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE, OR
* NON-INFRINGEMENT. SUN SHALL NOT BE LIABLE FOR ANY DAMAGES SUFFERED BY
* LICENSEE AS A RESULT OF USING, MODIFYING OR DISTRIBUTING THIS SOFTWARE OR ITS
* DERIVATIVES.
*/
import java.util.Timer;
import java.util.TimerTask;
import javax.microedition.lcdui.Choice;
import javax.microedition.lcdui.ChoiceGroup;
import javax.microedition.lcdui.Command;
import javax.microedition.lcdui.CommandListener;
import javax.microedition.lcdui.Display;
import javax.microedition.lcdui.Displayable;
import javax.microedition.lcdui.Form;
import javax.microedition.lcdui.List;
import javax.microedition.lcdui.Ticker;
import javax.microedition.midlet.MIDlet;
import javax.microedition.midlet.MIDletStateChangeException;
public class TimerMIDlet extends MIDlet implements CommandListener {
private Display display = null;
private Ticker stockTicker = null;
private String[] _stocks = { "SUNW", "ORCL", "NOK", "MOT" };
private String[] _prices = { "9", "11", "9.25", "14.5" };
private static final Command exitCommand = new Command("Exit",
Command.STOP, 0);
private static final Command backCommand = new Command("Back",
Command.BACK, 0);
private static final Command doneCommand = new Command("Done", Command.OK,
0);
private Timer stockRefresh = null;
private StockRefreshTask stockRefreshTask = null;
private int refresh_interval = 10000; // 1000 = 1 second
private List menu = null;
private ChoiceGroup _updatesChoices = null;
private Form _updatesForm = null;
private String _currentMenu = null;
public TimerMIDlet() {
}
public void startApp() throws MIDletStateChangeException {
display = Display.getDisplay(this);
menu = new List("Stock Menu", Choice.IMPLICIT);
menu.append("Updates", null);
menu.append("Add Stocks", null);
menu.append("Remove Stocks", null);
menu.addCommand(exitCommand);
menu.setCommandListener(this);
// Make the ticker
stockTicker = new Ticker(makeTickerString());
menu.setTicker(stockTicker);
display.setCurrent(menu);
_currentMenu = "Stock Menu";
_updatesForm = new Form("Updates");
_updatesChoices = new ChoiceGroup("Update Interval:", Choice.EXCLUSIVE);
_updatesChoices.append("Continuous", null); // will be 10 seconds
_updatesChoices.append("15 minutes", null);
_updatesChoices.append("30 minutes", null);
_updatesChoices.append("1 hour", null);
_updatesChoices.append("3 hours", null);
_updatesForm.setTicker(stockTicker);
_updatesForm.append(_updatesChoices);
_updatesForm.addCommand(backCommand);
_updatesForm.addCommand(doneCommand);
_updatesForm.setCommandListener(this);
//Set up and start the timer to refresh the stock quotes
stockRefreshTask = new StockRefreshTask();
stockRefresh = new Timer();
stockRefresh.schedule(stockRefreshTask, 0, refresh_interval);
}
public String makeTickerString() {
String retString = new String();
for (int i = 0; i < _stocks.length; i++) {
retString += _stocks[i];
retString += " @ ";
retString += _prices[i];
retString += " ";
}
return retString;
}
public void pauseApp() {
// free memory used by these objects
display = null;
stockRefresh = null;
stockRefreshTask = null;
}
public void destroyApp(boolean unconditional)
throws MIDletStateChangeException {
notifyDestroyed();
}
public void commandAction(Command c, Displayable d) {
if (c == exitCommand) {
try {
destroyApp(false);
} catch (MIDletStateChangeException msce) {
System.out.println("Error in detroyApp(false) ");
msce.printStackTrace();
}
notifyDestroyed();
} else if (c == backCommand) {
_currentMenu = "Stock Menu";
display.setCurrent(menu);
} else if (c == doneCommand) {
switch (_updatesChoices.getSelectedIndex()) {
case 0:
refresh_interval = 10000;
break;
case 1:
refresh_interval = 900000;
break;
case 2:
refresh_interval = 1800000;
break;
case 3:
refresh_interval = 3600000;
break;
case 4:
refresh_interval = 10800000;
break;
default:
break;
}
stockRefreshTask.cancel();
stockRefreshTask = new StockRefreshTask();
stockRefresh.schedule(stockRefreshTask, 0, refresh_interval);
display.setCurrent(menu);
_currentMenu = "Stock Menu";
} else {
List shown = (List) display.getCurrent();
switch (shown.getSelectedIndex()) {
case 0: // Updates
display.setCurrent(_updatesForm);
_currentMenu = "Updates";
break;
case 1: // Add Stock
System.out.println("Add Stock... ");
_currentMenu = "Add Stock";
break;
case 2: // Remove Stock
System.out.println("Remove Stock... ");
_currentMenu = "Remove Stock";
break;
}
}
}
/**
* This is an extention of the TimerTask class which runs when called by
* Timer. It refreshes the stock info for each stock from the quote server
* and checks to see if any of the alerts should be fired.
*/
class StockRefreshTask extends TimerTask {
/**
* Execute the Timer's Task
*/
public void run() {
try {
// Just return if the database is empty
if (_stocks.length == 0)
return;
else {
for (int i = 0; i < _stocks.length; i++) {
System.out.println("Stock price for symbol: "
+ _stocks[i] + " is: " + _prices[i]);
}
}
} catch (Exception e) {
System.out.println("error('UPDATE FAILED', 2000)");
}
}
}
}
Related examples in the same category