Place an icon with a popup menu on the system tray
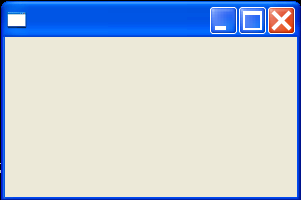
/*
* Tray example snippet: place an icon with a popup menu on the system tray
*
* For a list of all SWT example snippets see
* http://dev.eclipse.org/viewcvs/index.cgi/%7Echeckout%7E/platform-swt-home/dev.html#snippets
*/
import org.eclipse.swt.SWT;
import org.eclipse.swt.graphics.Image;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Event;
import org.eclipse.swt.widgets.Listener;
import org.eclipse.swt.widgets.Menu;
import org.eclipse.swt.widgets.MenuItem;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.Tray;
import org.eclipse.swt.widgets.TrayItem;
public class Snippet143 {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
Image image = new Image(display, 16, 16);
final Tray tray = display.getSystemTray();
if (tray == null) {
System.out.println("The system tray is not available");
} else {
final TrayItem item = new TrayItem(tray, SWT.NONE);
item.setToolTipText("SWT TrayItem");
item.addListener(SWT.Show, new Listener() {
public void handleEvent(Event event) {
System.out.println("show");
}
});
item.addListener(SWT.Hide, new Listener() {
public void handleEvent(Event event) {
System.out.println("hide");
}
});
item.addListener(SWT.Selection, new Listener() {
public void handleEvent(Event event) {
System.out.println("selection");
}
});
item.addListener(SWT.DefaultSelection, new Listener() {
public void handleEvent(Event event) {
System.out.println("default selection");
}
});
final Menu menu = new Menu(shell, SWT.POP_UP);
for (int i = 0; i < 8; i++) {
MenuItem mi = new MenuItem(menu, SWT.PUSH);
mi.setText("Item" + i);
}
item.addListener(SWT.MenuDetect, new Listener() {
public void handleEvent(Event event) {
menu.setVisible(true);
}
});
item.setImage(image);
}
shell.setBounds(50, 50, 300, 200);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
image.dispose();
display.dispose();
}
}
Related examples in the same category