A frame with a toolbar and menu for color changes
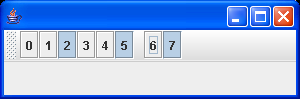
/*
This program is a part of the companion code for Core Java 8th ed.
(http://horstmann.com/corejava)
This program is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program. If not, see <http://www.gnu.org/licenses/>.
*/
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.EventQueue;
import java.awt.event.ActionEvent;
import javax.swing.AbstractAction;
import javax.swing.Action;
import javax.swing.Icon;
import javax.swing.ImageIcon;
import javax.swing.JFrame;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JPanel;
import javax.swing.JToolBar;
/**
* @version 1.13 2007-06-12
* @author Cay Horstmann
*/
public class ToolBarTest
{
public static void main(String[] args)
{
EventQueue.invokeLater(new Runnable()
{
public void run()
{
ToolBarFrame frame = new ToolBarFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
});
}
}
/**
* A frame with a toolbar and menu for color changes.
*/
class ToolBarFrame extends JFrame
{
public ToolBarFrame()
{
setTitle("ToolBarTest");
setSize(DEFAULT_WIDTH, DEFAULT_HEIGHT);
// add a panel for color change
panel = new JPanel();
add(panel, BorderLayout.CENTER);
// set up actions
Action blueAction = new ColorAction("Blue", new ImageIcon("blue-ball.gif"), Color.BLUE);
Action yellowAction = new ColorAction("Yellow", new ImageIcon("yellow-ball.gif"),
Color.YELLOW);
Action redAction = new ColorAction("Red", new ImageIcon("red-ball.gif"), Color.RED);
Action exitAction = new AbstractAction("Exit", new ImageIcon("exit.gif"))
{
public void actionPerformed(ActionEvent event)
{
System.exit(0);
}
};
exitAction.putValue(Action.SHORT_DESCRIPTION, "Exit");
// populate tool bar
JToolBar bar = new JToolBar();
bar.add(blueAction);
bar.add(yellowAction);
bar.add(redAction);
bar.addSeparator();
bar.add(exitAction);
add(bar, BorderLayout.NORTH);
// populate menu
JMenu menu = new JMenu("Color");
menu.add(yellowAction);
menu.add(blueAction);
menu.add(redAction);
menu.add(exitAction);
JMenuBar menuBar = new JMenuBar();
menuBar.add(menu);
setJMenuBar(menuBar);
}
public static final int DEFAULT_WIDTH = 300;
public static final int DEFAULT_HEIGHT = 200;
private JPanel panel;
/**
* The color action sets the background of the frame to a given color.
*/
class ColorAction extends AbstractAction
{
public ColorAction(String name, Icon icon, Color c)
{
putValue(Action.NAME, name);
putValue(Action.SMALL_ICON, icon);
putValue(Action.SHORT_DESCRIPTION, name + " background");
putValue("Color", c);
}
public void actionPerformed(ActionEvent event)
{
Color c = (Color) getValue("Color");
panel.setBackground(c);
}
}
}
Related examples in the same category