Change Look and feel
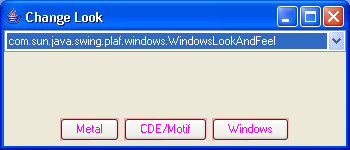
/*
Definitive Guide to Swing for Java 2, Second Edition
By John Zukowski
ISBN: 1-893115-78-X
Publisher: APress
*/
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Container;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.DefaultComboBoxModel;
import javax.swing.JButton;
import javax.swing.JComboBox;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.SwingUtilities;
import javax.swing.UIDefaults;
import javax.swing.UIManager;
public class ChangeLook {
public static void main(String args[]) {
final JFrame frame = new JFrame("Change Look");
ActionListener actionListener = new ActionListener() {
public void actionPerformed(ActionEvent actionEvent) {
Object source = actionEvent.getSource();
String lafClassName = null;
if (source instanceof JComboBox) {
JComboBox comboBox = (JComboBox) source;
lafClassName = (String) comboBox.getSelectedItem();
} else if (source instanceof JButton) {
lafClassName = actionEvent.getActionCommand();
}
if (lafClassName != null) {
final String finalLafClassName = lafClassName;
Runnable runnable = new Runnable() {
public void run() {
try {
UIManager.setLookAndFeel(finalLafClassName);
SwingUtilities.updateComponentTreeUI(frame);
} catch (Exception exception) {
JOptionPane.showMessageDialog(frame,
"Can't change look and feel",
"Invalid PLAF",
JOptionPane.ERROR_MESSAGE);
}
}
};
SwingUtilities.invokeLater(runnable);
}
}
};
Object newSettings[] = { "Button.background", Color.pink,
"Button.foreground", Color.magenta };
UIDefaults defaults = UIManager.getDefaults();
defaults.putDefaults(newSettings);
UIManager.LookAndFeelInfo looks[] = UIManager
.getInstalledLookAndFeels();
DefaultComboBoxModel model = new DefaultComboBoxModel();
JComboBox comboBox = new JComboBox(model);
JPanel panel = new JPanel();
for (int i = 0, n = looks.length; i < n; i++) {
JButton button = new JButton(looks[i].getName());
model.addElement(looks[i].getClassName());
button.setActionCommand(looks[i].getClassName());
button.addActionListener(actionListener);
panel.add(button);
}
comboBox.addActionListener(actionListener);
Container contentPane = frame.getContentPane();
contentPane.add(comboBox, BorderLayout.NORTH);
contentPane.add(panel, BorderLayout.SOUTH);
frame.setSize(350, 150);
frame.setVisible(true);
}
}
Related examples in the same category