Pmw.PanedWidget (Split pane): add, move and delete
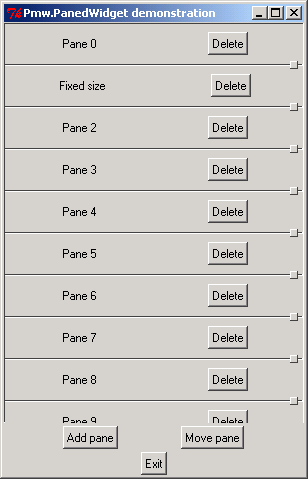
#Pmw copyright
#Copyright 1997-1999 Telstra Corporation Limited, Australia
#Copyright 2000-2002 Really Good Software Pty Ltd, Australia
#Permission is hereby granted, free of charge, to any person obtaining a copy
#of this software and associated documentation files (the "Software"), to deal
#in the Software without restriction, including without limitation the rights
#to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
#copies of the Software, and to permit persons to whom the Software is furnished
#to do so, subject to the following conditions:
#The above copyright notice and this permission notice shall be included in all
#copies or substantial portions of the Software.
#THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
#INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A
#PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
#HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
#OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
#SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
title = 'Pmw.PanedWidget demonstration'
# Import Pmw from this directory tree.
import sys
sys.path[:0] = ['../../..']
import Tkinter
import Pmw
class Demo:
def __init__(self, parent):
# Create a main PanedWidget with a few panes.
self.pw = Pmw.PanedWidget(parent,
orient='vertical',
hull_borderwidth = 1,
hull_relief = 'sunken',
hull_width=300,
hull_height=400)
for self.numPanes in range(4):
if self.numPanes == 1:
name = 'Fixed size'
pane = self.pw.add(name, min = .1, max = .1)
else:
name = 'Pane ' + str(self.numPanes)
pane = self.pw.add(name, min = .1, size = .25)
label = Tkinter.Label(pane, text = name)
label.pack(side = 'left', expand = 1)
button = Tkinter.Button(pane, text = 'Delete',
command = lambda s=self, n=name: s.deletePane(n))
button.pack(side = 'left', expand = 1)
# TODO: add buttons to invoke self.moveOneUp and self.moveOneUp.
self.pw.pack(expand = 1, fill='both')
buttonBox = Pmw.ButtonBox(parent)
buttonBox.pack(fill = 'x')
buttonBox.add('Add pane', command = self.addPane)
buttonBox.add('Move pane', command = self.move)
self.moveSrc = 0
self.moveNewPos = 1
self.moveBack = 0
def move(self):
numPanes = len(self.pw.panes())
if numPanes == 0:
print 'No panes to move!'
return
if self.moveSrc >= numPanes:
self.moveSrc = numPanes - 1
if self.moveNewPos >= numPanes:
self.moveNewPos = numPanes - 1
print 'Moving pane', self.moveSrc, 'to new position', self.moveNewPos
self.pw.move(self.moveSrc, self.moveNewPos)
self.moveSrc, self.moveNewPos = self.moveNewPos, self.moveSrc
if self.moveBack:
if self.moveNewPos == numPanes - 1:
self.moveNewPos = 0
if self.moveSrc == numPanes - 1:
self.moveSrc = 0
else:
self.moveSrc = self.moveSrc + 1
else:
self.moveNewPos = self.moveNewPos + 1
self.moveBack = not self.moveBack
def addPane(self):
self.numPanes = self.numPanes + 1
name = 'Pane ' + str(self.numPanes)
print 'Adding', name
pane = self.pw.add(name, min = .1, size = .25)
label = Tkinter.Label(pane, text = name)
label.pack(side = 'left', expand = 1)
button = Tkinter.Button(pane, text = 'Delete',
command = lambda s=self, n=name: s.deletePane(n))
button.pack(side = 'left', expand = 1)
self.pw.updatelayout()
def deletePane(self, name):
print 'Deleting', name
self.pw.delete(name)
self.pw.updatelayout()
def moveOneUp(self, name):
self.pw.move(name, name, -1)
def moveOneDown(self, name):
self.pw.move(name, name, 1)
######################################################################
# Create demo in root window for testing.
if __name__ == '__main__':
root = Tkinter.Tk()
Pmw.initialise(root)
root.title(title)
exitButton = Tkinter.Button(root, text = 'Exit', command = root.destroy)
exitButton.pack(side = 'bottom')
widget = Demo(root)
root.mainloop()
Related examples in the same category