Pmw.PanedWidget: add components
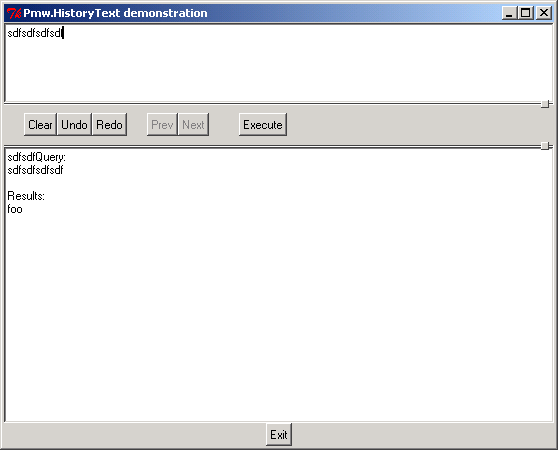
#Pmw copyright
#Copyright 1997-1999 Telstra Corporation Limited, Australia
#Copyright 2000-2002 Really Good Software Pty Ltd, Australia
#Permission is hereby granted, free of charge, to any person obtaining a copy
#of this software and associated documentation files (the "Software"), to deal
#in the Software without restriction, including without limitation the rights
#to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
#copies of the Software, and to permit persons to whom the Software is furnished
#to do so, subject to the following conditions:
#The above copyright notice and this permission notice shall be included in all
#copies or substantial portions of the Software.
#THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
#INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A
#PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
#HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
#OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
#SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
title = 'Pmw.HistoryText demonstration'
# Import Pmw from this directory tree.
import sys
sys.path[:0] = ['../../..']
import Tkinter
import Pmw
class Demo:
def __init__(self, parent):
# Create and pack the PanedWidget to hold the query and result
# windows.
# !! panedwidget should automatically size to requested size
panedWidget = Pmw.PanedWidget(parent,
orient = 'vertical',
hull_height = 400,
hull_width = 550)
panedWidget.add('query', min = 0.05, size = 0.2)
panedWidget.add('buttons', min = 0.1, max = 0.1)
panedWidget.add('results', min = 0.05)
panedWidget.pack(fill = 'both', expand = 1)
# Create and pack the HistoryText.
self.historyText = Pmw.HistoryText(panedWidget.pane('query'),
text_wrap = 'none',
text_width = 60,
text_height = 10,
historycommand = self.statechange,
)
self.historyText.pack(fill = 'both', expand = 1)
self.historyText.component('text').focus()
buttonList = (
[20, None],
['Clear', self.clear],
['Undo', self.historyText.undo],
['Redo', self.historyText.redo],
[20, None],
['Prev', self.historyText.prev],
['Next', self.historyText.next],
[30, None],
['Execute', Pmw.busycallback(self.executeQuery)],
)
self.buttonDict = {}
buttonFrame = panedWidget.pane('buttons')
for text, cmd in buttonList:
if type(text) == type(69):
frame = Tkinter.Frame(buttonFrame, width = text)
frame.pack(side = 'left')
else:
button = Tkinter.Button(buttonFrame, text = text, command = cmd)
button.pack(side = 'left')
self.buttonDict[text] = button
for text in ('Prev', 'Next'):
self.buttonDict[text].configure(state = 'disabled')
self.results = Pmw.ScrolledText(panedWidget.pane('results'), text_wrap = 'none')
self.results.pack(fill = 'both', expand = 1)
def statechange(self, prevstate, nextstate):
self.buttonDict['Prev'].configure(state = prevstate)
self.buttonDict['Next'].configure(state = nextstate)
def clear(self):
self.historyText.delete('1.0', 'end')
def addnewlines(self, text):
if len(text) == 1:
text = text + '\n'
if text[-1] != '\n':
text = text + '\n'
if text[-2] != '\n':
text = text + '\n'
return text
def executeQuery(self):
sql = self.historyText.get()
self.results.insert('end', 'Query:\n' + self.addnewlines(sql))
self.results.see('end')
self.results.update_idletasks()
self.historyText.addhistory()
results = 'Results:\nfoo'
if len(results) > 0:
self.results.insert('end', self.addnewlines(results))
self.results.see('end')
######################################################################
# Create demo in root window for testing.
if __name__ == '__main__':
root = Tkinter.Tk()
Pmw.initialise(root)
root.title(title)
exitButton = Tkinter.Button(root, text = 'Exit', command = root.destroy)
exitButton.pack(side = 'bottom')
widget = Demo(root)
root.mainloop()
Related examples in the same category