Color Dialog: Changing the background and text colors of a form
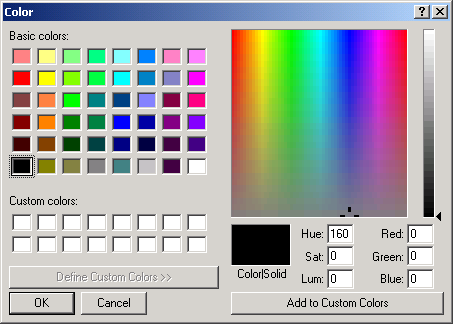
Imports System
Imports System.Drawing
Imports System.Windows.Forms
Public Class MainClass
Shared Sub Main()
Dim myform As Form = New FrmColorDialogTest()
Application.Run(myform)
End Sub ' Main
End Class
Public Class FrmColorDialogTest
Inherits System.Windows.Forms.Form
Friend WithEvents cmdBackgroundButton As Button
Friend WithEvents cmdTextButton As Button
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.Container
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.cmdBackgroundButton = New System.Windows.Forms.Button()
Me.cmdTextButton = New System.Windows.Forms.Button()
Me.SuspendLayout()
'
'cmdBackgroundButton
'
Me.cmdBackgroundButton.Location = New System.Drawing.Point(16, 16)
Me.cmdBackgroundButton.Name = "cmdBackgroundButton"
Me.cmdBackgroundButton.Size = New System.Drawing.Size(160, 24)
Me.cmdBackgroundButton.TabIndex = 0
Me.cmdBackgroundButton.Text = "Change Background Color"
'
'cmdTextButton
'
Me.cmdTextButton.Location = New System.Drawing.Point(16, 56)
Me.cmdTextButton.Name = "cmdTextButton"
Me.cmdTextButton.Size = New System.Drawing.Size(160, 24)
Me.cmdTextButton.TabIndex = 1
Me.cmdTextButton.Text = "Change Text Color"
'
'FrmColorDialogTest
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.ClientSize = New System.Drawing.Size(192, 93)
Me.Controls.AddRange(New System.Windows.Forms.Control() {Me.cmdTextButton, Me.cmdBackgroundButton})
Me.Name = "FrmColorDialogTest"
Me.Text = "Using Color Dialogs"
Me.ResumeLayout(False)
End Sub
#End Region
Private Sub cmdTextButton_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles cmdTextButton.Click
Dim colorBox As ColorDialog = New ColorDialog()
Dim result As DialogResult
result = colorBox.ShowDialog()
If result = DialogResult.Cancel Then
Return
End If
cmdBackgroundButton.ForeColor = colorBox.Color
cmdTextButton.ForeColor = colorBox.Color
End Sub
Private Sub cmdBackgroundButton_Click( _
ByVal sender As System.Object, _
ByVal e As System.EventArgs) _
Handles cmdBackgroundButton.Click
Dim colorBox As ColorDialog = New ColorDialog()
Dim result As DialogResult
colorBox.FullOpen = True
result = colorBox.ShowDialog()
If result = DialogResult.Cancel Then
Return
End If
Me.BackColor = colorBox.Color
End Sub
End Class
Related examples in the same category