Get all Known color and calcuate its Brightness and Saturation
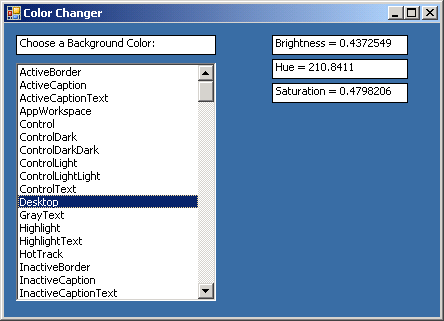
Imports System
Imports System.Drawing
Imports System.Windows.Forms
Imports System.IO
public class MainClass
Shared Sub Main()
Dim form1 As Form = New Form1
Application.Run(form1)
End Sub
End Class
Public Class Form1
Inherits System.Windows.Forms.Form
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
Friend WithEvents lstColors As System.Windows.Forms.ListBox
Friend WithEvents Label1 As System.Windows.Forms.Label
Friend WithEvents lblBrightness As System.Windows.Forms.Label
Friend WithEvents lblHue As System.Windows.Forms.Label
Friend WithEvents lblSaturation As System.Windows.Forms.Label
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.lstColors = New System.Windows.Forms.ListBox()
Me.Label1 = New System.Windows.Forms.Label()
Me.lblBrightness = New System.Windows.Forms.Label()
Me.lblHue = New System.Windows.Forms.Label()
Me.lblSaturation = New System.Windows.Forms.Label()
Me.SuspendLayout()
'
'lstColors
'
Me.lstColors.Location = New System.Drawing.Point(12, 40)
Me.lstColors.Name = "lstColors"
Me.lstColors.Size = New System.Drawing.Size(200, 238)
Me.lstColors.TabIndex = 0
'
'Label1
'
Me.Label1.BackColor = System.Drawing.SystemColors.ActiveCaptionText
Me.Label1.BorderStyle = System.Windows.Forms.BorderStyle.FixedSingle
Me.Label1.FlatStyle = System.Windows.Forms.FlatStyle.System
Me.Label1.Location = New System.Drawing.Point(12, 12)
Me.Label1.Name = "Label1"
Me.Label1.Size = New System.Drawing.Size(200, 20)
Me.Label1.TabIndex = 1
Me.Label1.Text = " Choose a Background Color:"
'
'lblBrightness
'
Me.lblBrightness.BackColor = System.Drawing.SystemColors.ActiveCaptionText
Me.lblBrightness.BorderStyle = System.Windows.Forms.BorderStyle.FixedSingle
Me.lblBrightness.FlatStyle = System.Windows.Forms.FlatStyle.System
Me.lblBrightness.Location = New System.Drawing.Point(268, 12)
Me.lblBrightness.Name = "lblBrightness"
Me.lblBrightness.Size = New System.Drawing.Size(136, 20)
Me.lblBrightness.TabIndex = 2
Me.lblBrightness.Text = " Brightness"
'
'lblHue
'
Me.lblHue.BackColor = System.Drawing.SystemColors.ActiveCaptionText
Me.lblHue.BorderStyle = System.Windows.Forms.BorderStyle.FixedSingle
Me.lblHue.FlatStyle = System.Windows.Forms.FlatStyle.System
Me.lblHue.Location = New System.Drawing.Point(268, 36)
Me.lblHue.Name = "lblHue"
Me.lblHue.Size = New System.Drawing.Size(136, 20)
Me.lblHue.TabIndex = 3
Me.lblHue.Text = " Hue"
'
'lblSaturation
'
Me.lblSaturation.BackColor = System.Drawing.SystemColors.ActiveCaptionText
Me.lblSaturation.BorderStyle = System.Windows.Forms.BorderStyle.FixedSingle
Me.lblSaturation.FlatStyle = System.Windows.Forms.FlatStyle.System
Me.lblSaturation.Location = New System.Drawing.Point(268, 60)
Me.lblSaturation.Name = "lblSaturation"
Me.lblSaturation.Size = New System.Drawing.Size(136, 20)
Me.lblSaturation.TabIndex = 4
Me.lblSaturation.Text = " Saturation"
'
'Form1
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 14)
Me.ClientSize = New System.Drawing.Size(436, 294)
Me.Controls.AddRange(New System.Windows.Forms.Control() {Me.lblSaturation, Me.lblHue, Me.lblBrightness, Me.Label1, Me.lstColors})
Me.Font = New System.Drawing.Font("Tahoma", 8.25!, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, CType(0, Byte))
Me.Name = "Form1"
Me.Text = "Color Changer"
Me.ResumeLayout(False)
End Sub
#End Region
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
Dim ColorNames() As String
ColorNames = System.Enum.GetNames(GetType(KnownColor))
lstColors.Items.AddRange(ColorNames)
End Sub
Private Sub lstColors_SelectedIndexChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles lstColors.SelectedIndexChanged
Dim SelectedColor As KnownColor
SelectedColor = System.Enum.Parse(GetType(KnownColor), lstColors.Text)
Me.BackColor = System.Drawing.Color.FromKnownColor(SelectedColor)
lblBrightness.Text = " Brightness = " & Me.BackColor.GetBrightness.ToString()
lblHue.Text = " Hue = " & Me.BackColor.GetHue.ToString()
lblSaturation.Text = " Saturation = " & Me.BackColor.GetSaturation.ToString()
End Sub
End Class
Related examples in the same category