Define and use ReadOnly Class Property
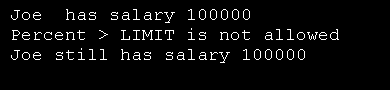
Imports System
Imports System.IO
Public Class MainClass
Shared Sub Main()
Dim e As New Employee("Joe", 100000)
Console.WriteLine(e.Name & " has salary " & e.Salary)
e.RaiseSalary(0.2D) 'D necessary for decimal
Console.WriteLine(e.Name & " still has salary " & e.Salary)
Console.WriteLine()
End Sub
End Class
Public Class Employee
Private m_Name As String
Private m_Salary As Decimal
Private Const LIMIT As Decimal = 0.1D
Public Sub New(ByVal theName As String, ByVal curSalary As Decimal)
m_Name = theName
m_Salary = curSalary
End Sub
ReadOnly Property Name() As String
Get
Return m_Name
End Get
End Property
ReadOnly Property Salary() As Decimal
Get
Return m_Salary
End Get
End Property
Public Overloads Sub RaiseSalary(ByVal Percent As Decimal)
If Percent > LIMIT Then
'not allowed
Console.WriteLine("Percent > LIMIT is not allowed")
Else
m_Salary = (1 + Percent) * m_Salary
End If
End Sub
Public Overloads Sub RaiseSalary(ByVal Percent As Decimal, _
ByVal Password As String)
If Password = "special" Then
m_Salary = (1 + Percent) * m_Salary
End If
End Sub
End Class
Related examples in the same category