Define and use your own Time Class
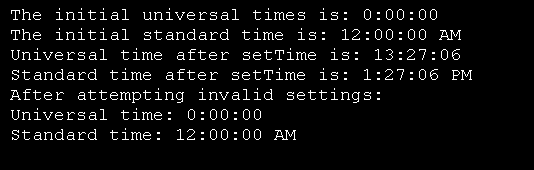
Imports System
Public Class MainClass
Shared Sub Main(ByVal args As String())
Dim time As New CTime() ' call CTime constructor
Console.WriteLine( "The initial universal times is: " & _
time.ToUniversalString() & vbCrLf & _
"The initial standard time is: " & _
time.ToStandardString() )
time.SetTime(13, 27, 6) ' set time with valid settings
Console.WriteLine( "Universal time after setTime is: " & _
time.ToUniversalString() & vbCrLf & _
"Standard time after setTime is: " & _
time.ToStandardString() )
time.SetTime(99, 99, 99) ' set time with invalid settings
Console.WriteLine( "After attempting invalid settings: " & vbCrLf & _
"Universal time: " & time.ToUniversalString() & _
vbCrLf & "Standard time: " & time.ToStandardString() )
End Sub
End Class
Class CTime
Inherits Object
Private mHour As Integer ' 0 - 23
Private mMinute As Integer ' 0 - 59
Private mSecond As Integer ' 0 - 59
Public Sub New()
SetTime(0, 0, 0)
End Sub ' New
Public Sub SetTime(ByVal hourValue As Integer, _
ByVal minuteValue As Integer, ByVal secondValue As Integer)
If (hourValue >= 0 AndAlso hourValue < 24) Then
mHour = hourValue
Else
mHour = 0
End If
If (minuteValue >= 0 AndAlso minuteValue < 60) Then
mMinute = minuteValue
Else
mMinute = 0
End If
If (secondValue >= 0 AndAlso secondValue < 60) Then
mSecond = secondValue
Else
mSecond = 0
End If
End Sub ' SetTime
' convert String to universal-time format
Public Function ToUniversalString() As String
Return String.Format("{0}:{1:D2}:{2:D2}", _
mHour, mMinute, mSecond)
End Function ' ToUniversalString
' convert to String in standard-time format
Public Function ToStandardString() As String
Dim suffix As String = " PM"
Dim format As String = "{0}:{1:D2}:{2:D2}"
Dim standardHour As Integer
' determine whether time is AM or PM
If mHour < 12 Then
suffix = " AM"
End If
' convert from universal-time format to standard-time format
If (mHour = 12 OrElse mHour = 0) Then
standardHour = 12
Else
standardHour = mHour Mod 12
End If
Return String.Format(format, standardHour, mMinute, _
mSecond) & suffix
End Function ' ToStandardString
End Class ' CTime
Related examples in the same category