Find a file: search directory recursively
Imports System
Imports System.Windows.Forms
Imports System.IO
Imports System.Text.RegularExpressions
Imports System.Collections.Specialized
Public Class MainClass
Shared Sub Main()
Dim myform As Form = New FrmFileSearch()
Application.Run(myform)
End Sub ' Main
End Class
Public Class FrmFileSearch
Inherits Form
' label that displays current directory
Friend WithEvents lblDirectory As Label
' label that displays directions to user
Friend WithEvents lblDirections As Label
' button that activates search
Friend WithEvents cmdSearch As Button
' text boxes for inputting and outputting data
Friend WithEvents txtInput As TextBox
Friend WithEvents txtOutput As TextBox
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.Container
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.txtOutput = New System.Windows.Forms.TextBox()
Me.lblDirections = New System.Windows.Forms.Label()
Me.lblDirectory = New System.Windows.Forms.Label()
Me.txtInput = New System.Windows.Forms.TextBox()
Me.cmdSearch = New System.Windows.Forms.Button()
Me.SuspendLayout()
'
'txtOutput
'
Me.txtOutput.AutoSize = False
Me.txtOutput.BackColor = System.Drawing.SystemColors.Control
Me.txtOutput.Location = New System.Drawing.Point(20, 276)
Me.txtOutput.Multiline = True
Me.txtOutput.Name = "txtOutput"
Me.txtOutput.ReadOnly = True
Me.txtOutput.ScrollBars = System.Windows.Forms.ScrollBars.Vertical
Me.txtOutput.Size = New System.Drawing.Size(461, 208)
Me.txtOutput.TabIndex = 4
Me.txtOutput.Text = ""
'
'lblDirections
'
Me.lblDirections.Font = New System.Drawing.Font("Microsoft Sans Serif", 10.0!, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, CType(0, Byte))
Me.lblDirections.Location = New System.Drawing.Point(20, 138)
Me.lblDirections.Name = "lblDirections"
Me.lblDirections.Size = New System.Drawing.Size(451, 20)
Me.lblDirections.TabIndex = 1
Me.lblDirections.Text = "Enter Path to Search:"
'
'lblDirectory
'
Me.lblDirectory.Font = New System.Drawing.Font("Microsoft Sans Serif", 10.0!, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, CType(0, Byte))
Me.lblDirectory.Location = New System.Drawing.Point(20, 20)
Me.lblDirectory.Name = "lblDirectory"
Me.lblDirectory.Size = New System.Drawing.Size(451, 98)
Me.lblDirectory.TabIndex = 0
Me.lblDirectory.Text = "Current Directory:"
'
'txtInput
'
Me.txtInput.Location = New System.Drawing.Point(20, 168)
Me.txtInput.Name = "txtInput"
Me.txtInput.Size = New System.Drawing.Size(461, 22)
Me.txtInput.TabIndex = 3
Me.txtInput.Text = ""
'
'cmdSearch
'
Me.cmdSearch.Font = New System.Drawing.Font("Microsoft Sans Serif", 9.75!, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, CType(0, Byte))
Me.cmdSearch.Location = New System.Drawing.Point(20, 217)
Me.cmdSearch.Name = "cmdSearch"
Me.cmdSearch.Size = New System.Drawing.Size(461, 40)
Me.cmdSearch.TabIndex = 2
Me.cmdSearch.Text = "Search Directory"
'
'FrmFileSearch
'
Me.AutoScaleBaseSize = New System.Drawing.Size(6, 15)
Me.ClientSize = New System.Drawing.Size(501, 499)
Me.Controls.AddRange(New System.Windows.Forms.Control() {Me.txtOutput, Me.txtInput, Me.cmdSearch, Me.lblDirections, Me.lblDirectory})
Me.Name = "FrmFileSearch"
Me.Text = "Using Regular Expressions"
Me.ResumeLayout(False)
End Sub
#End Region
Dim currentDirectory As String = Directory.GetCurrentDirectory
Dim directoryList As String()
Dim fileArray As String()
Dim found As NameValueCollection = New NameValueCollection()
Private Sub txtInput_KeyDown(ByVal sender As System.Object, _
ByVal e As System.Windows.Forms.KeyEventArgs) _
Handles txtInput.KeyDown
If (e.KeyCode = Keys.Enter) Then
cmdSearch_Click(sender, e)
End If
End Sub
Private Sub cmdSearch_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles cmdSearch.Click
Dim current As String
If txtInput.Text <> "" Then
' verify that user input is a valid directory name
If Directory.Exists(txtInput.Text) Then
currentDirectory = txtInput.Text
' reset input text box and update display
lblDirectory.Text = "Current Directory:" & vbCrLf & _
currentDirectory
' show error if user does not specify valid directory
Else
MessageBox.Show("Invalid Directory", "Error", _
MessageBoxButtons.OK, MessageBoxIcon.Error)
Return
End If
End If
' clear text boxes
txtInput.Text = ""
txtOutput.Text = ""
' search directory
SearchDirectory(currentDirectory)
' summarize and print results
For Each current In found
txtOutput.Text &= "* Found " & found(current) & " " _
& current & " files." & vbCrLf
Next
' clear output for new search
found.Clear()
End Sub ' cmdSearch_Click
' search directory using regular expression
Private Sub SearchDirectory(ByVal currentDirectory As String)
' for file name without directory path
Try
Dim fileName As String = ""
Dim myFile As String
Dim myDirectory As String
' regular expression for extensions matching pattern
Dim regularExpression As Regex = _
New Regex("([a-zA-Z0-9]+\.(?<extension>\w+))")
' stores regular-expression-match result
Dim matchResult As Match
Dim fileExtension As String ' holds file extensions
' number of files with given extension in directory
Dim extensionCount As Integer
' get directories
directoryList = _
Directory.GetDirectories(currentDirectory)
' get list of files in current directory
fileArray = Directory.GetFiles(currentDirectory)
' iterate through list of files
For Each myFile In fileArray
fileName = myFile.Substring( _
myFile.LastIndexOf("\") + 1)
matchResult = regularExpression.Match(fileName)
If (matchResult.Success) Then
fileExtension = matchResult.Result("${extension}")
Else
fileExtension = "[no extension]"
End If
If (found(fileExtension) = Nothing) Then
found.Add(fileExtension, "1")
Else
extensionCount = _
Convert.ToInt32(found(fileExtension)) + 1
found(fileExtension) = extensionCount.ToString()
End If
Next
For Each myDirectory In directoryList
SearchDirectory(myDirectory)
Next
Catch unauthorizedAccess As UnauthorizedAccessException
MessageBox.Show("Some files may not be visible due to" _
& " permission settings", "Warning", _
MessageBoxButtons.OK, MessageBoxIcon.Information)
End Try
End Sub
End Class
Related examples in the same category
1. | Get all directories | | |
2. | Recursive Directory Info | | 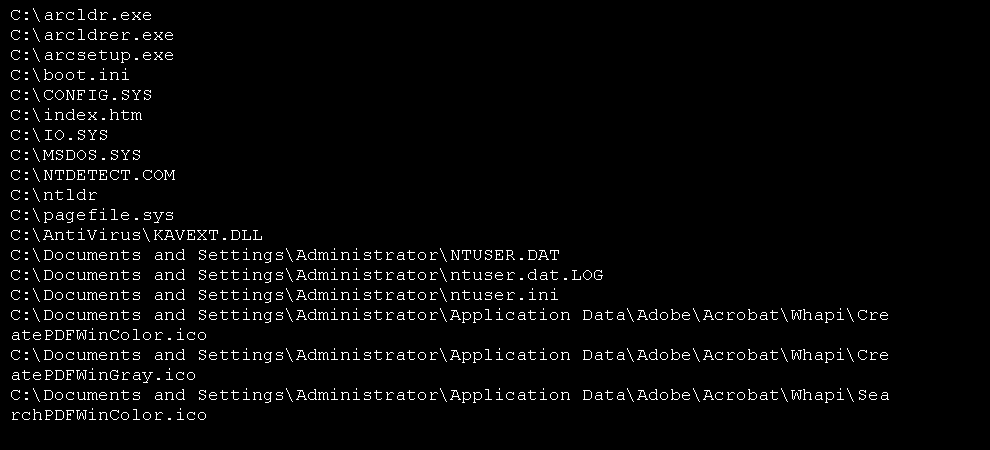 |
3. | Display Directory Tree | | 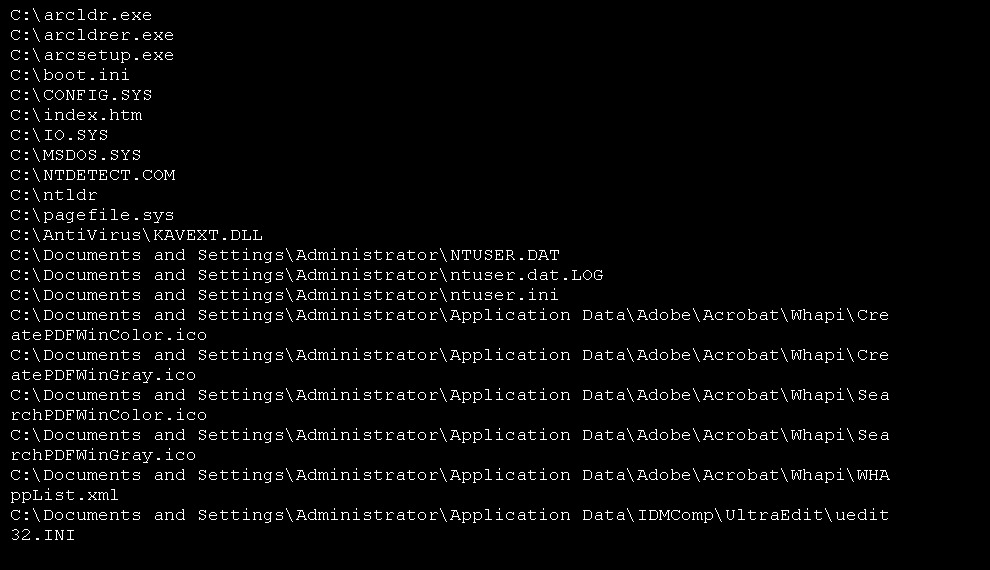 |
4. | List files and directories in root | | 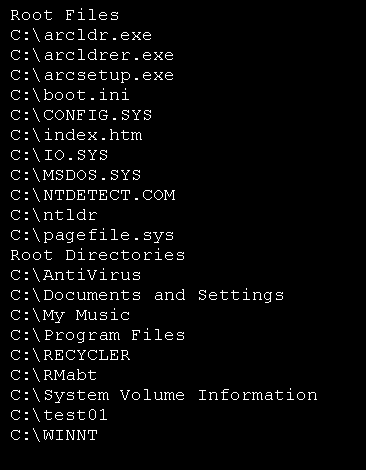 |
5. | Directory Information: name, last update and create time | | 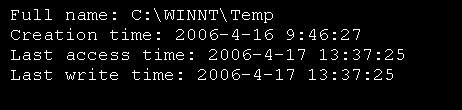 |
6. | Update Directory: create time, last access time and last write time | |  |
7. | Get Root directory and current directory | |  |
8. | Directory Separator | |  |
9. | Get Current Directory | |  |
10. | Get file in the parent folder | |  |
11. | Display all file under a Directory | |  |
12. | Directory Class Exposes static methods for creating, moving, and enumerating through directories and subdirectories | | |
13. | Move the directory | | |
14. | Count the files in a directory | | |
15. | Calculate the size of a directory and its subdirectories and displays the total size in bytes. | | |
16. | Gets or sets the attributes for the current file or directory. | | |
17. | FileSystemInfo.LastAccessTime Gets or sets the time the current file or directory was last accessed. | | |
18. | Path Class performs operations on file or directory path | | |
19. | Directory.CreateDirectory | | |
20. | Directory.Delete deletes an empty directory from a specified path. | | |
21. | Deletes the specified directory and subdirectories and files in the directory. | | |
22. | Directory.EnumerateDirectories returns an enumerable collection of directory names in a specified path. | | |
23. | Returns an enumerable collection of directory names that match a search pattern in a specified path. | | |
24. | Returns directory names that match a search pattern in a specified path, and optionally searches subdirectories. | | |
25. | Determines whether the given path refers to an existing directory on disk. | | |
26. | Gets the creation date and time of a directory. | | |
27. | Gets the creation date and time, in Coordinated Universal Time (UTC) format, of a directory. | | |
28. | Gets the current working directory of the application. | | |
29. | Gets the names of subdirectories in the specified directory. | | |
30. | Gets an array of directories matching the specified search pattern from the current directory. | | |
31. | Returns the names of files (including their paths) that match the specified search pattern in the specified directory. | | |
32. | Returns the date and time the specified file or directory was last accessed. | | |
33. | Returns the date and time the specified file or directory was last written to. | | |
34. | Sets the date and time the specified file or directory was last accessed. | | |
35. | Sets the date and time a directory was last written to. | | |
36. | Returns an enumerable collection of file names in a specified path. | | |
37. | Returns file names that match a search pattern in a specified path. | | |
38. | Returns file names that match a search pattern in a specified path, and optionally searches subdirectories. | | |
39. | Returns the volume information, root information, or both for the specified path. | | |