Gets an array of directories matching the specified search pattern from the current directory.
Imports System
Imports System.IO
Public Class Test
Public Shared Sub Main()
Try
Dim dirs As String() = Directory.GetDirectories("c:\", "p*")
Console.WriteLine("The number of directories starting with p is {0}.", dirs.Length)
Dim dir As String
For Each dir In dirs
Console.WriteLine(dir)
Next
Catch e As Exception
Console.WriteLine("The process failed: {0}", e.ToString())
End Try
End Sub
End Class
Related examples in the same category
1. | Get all directories | | |
2. | Recursive Directory Info | | 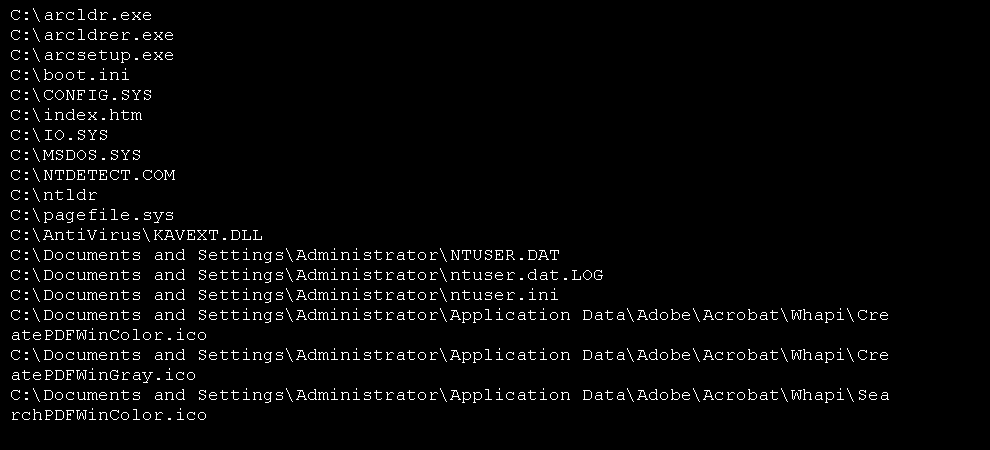 |
3. | Display Directory Tree | | 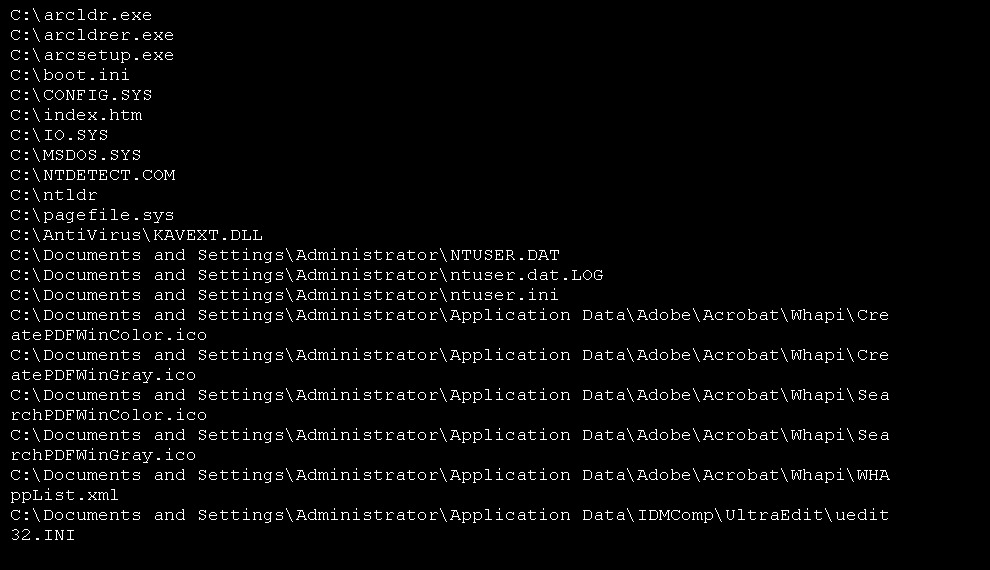 |
4. | List files and directories in root | | 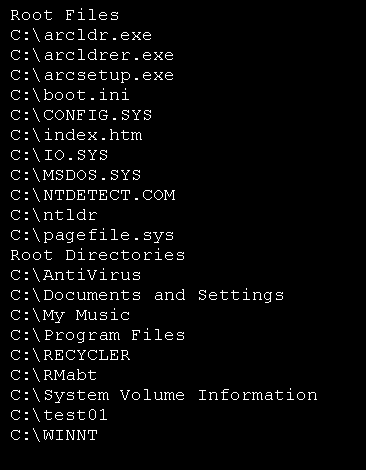 |
5. | Directory Information: name, last update and create time | | 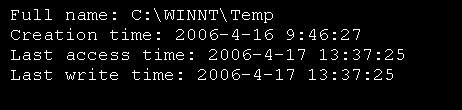 |
6. | Update Directory: create time, last access time and last write time | |  |
7. | Get Root directory and current directory | |  |
8. | Directory Separator | |  |
9. | Get Current Directory | |  |
10. | Get file in the parent folder | |  |
11. | Display all file under a Directory | |  |
12. | Find a file: search directory recursively | | |
13. | Directory Class Exposes static methods for creating, moving, and enumerating through directories and subdirectories | | |
14. | Move the directory | | |
15. | Count the files in a directory | | |
16. | Calculate the size of a directory and its subdirectories and displays the total size in bytes. | | |
17. | Gets or sets the attributes for the current file or directory. | | |
18. | FileSystemInfo.LastAccessTime Gets or sets the time the current file or directory was last accessed. | | |
19. | Path Class performs operations on file or directory path | | |
20. | Directory.CreateDirectory | | |
21. | Directory.Delete deletes an empty directory from a specified path. | | |
22. | Deletes the specified directory and subdirectories and files in the directory. | | |
23. | Directory.EnumerateDirectories returns an enumerable collection of directory names in a specified path. | | |
24. | Returns an enumerable collection of directory names that match a search pattern in a specified path. | | |
25. | Returns directory names that match a search pattern in a specified path, and optionally searches subdirectories. | | |
26. | Determines whether the given path refers to an existing directory on disk. | | |
27. | Gets the creation date and time of a directory. | | |
28. | Gets the creation date and time, in Coordinated Universal Time (UTC) format, of a directory. | | |
29. | Gets the current working directory of the application. | | |
30. | Gets the names of subdirectories in the specified directory. | | |
31. | Returns the names of files (including their paths) that match the specified search pattern in the specified directory. | | |
32. | Returns the date and time the specified file or directory was last accessed. | | |
33. | Returns the date and time the specified file or directory was last written to. | | |
34. | Sets the date and time the specified file or directory was last accessed. | | |
35. | Sets the date and time a directory was last written to. | | |
36. | Returns an enumerable collection of file names in a specified path. | | |
37. | Returns file names that match a search pattern in a specified path. | | |
38. | Returns file names that match a search pattern in a specified path, and optionally searches subdirectories. | | |
39. | Returns the volume information, root information, or both for the specified path. | | |