Drag and drop serialized Object base on XML
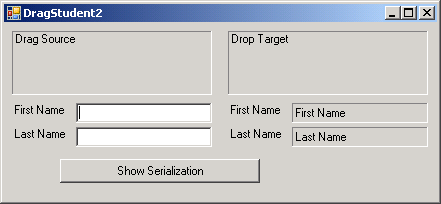
Imports System
Imports System.Collections
Imports System.Data
Imports System.Xml.Serialization
Imports System.Drawing
Imports System.Windows.Forms
Imports System.ComponentModel
Imports System.Drawing.Drawing2D
Imports System.IO
Public Class MainClass
Shared Sub Main()
Dim form1 As Form = New Form1
Application.Run(form1)
End Sub
End Class
Public Class Form1
<Serializable()> _
Public Class Student
Public FirstName As String
Public LastName As String
Public Sub New()
End Sub
Public Sub New(ByVal first_name As String, ByVal last_name As String)
FirstName = first_name
LastName = last_name
End Sub
End Class
Private Sub lblDragSource_MouseDown(ByVal sender As Object, ByVal e As System.Windows.Forms.MouseEventArgs) Handles lblDragSource.MouseDown
Dim emp As New Student(txtFirstName.Text, txtLastName.Text)
Dim data_object As New DataObject()
data_object.SetData("Student", emp)
If lblDragSource.DoDragDrop(data_object, _
DragDropEffects.Copy Or DragDropEffects.Move) = DragDropEffects.Move _
Then
txtFirstName.Text = ""
txtLastName.Text = ""
End If
End Sub
Private Sub lblDropTarget_DragOver(ByVal sender As Object, ByVal e As System.Windows.Forms.DragEventArgs) Handles lblDropTarget.DragOver
If e.Data.GetDataPresent("Student") Then
Const KEY_CTRL As Integer = 8
If (e.KeyState And KEY_CTRL) <> 0 Then
e.Effect = DragDropEffects.Copy
Else
e.Effect = DragDropEffects.Move
End If
End If
End Sub
Private Sub lblDropTarget_DragDrop(ByVal sender As Object, ByVal e As System.Windows.Forms.DragEventArgs) Handles lblDropTarget.DragDrop
Dim emp As Student = DirectCast(e.Data.GetData("Student"), Student)
lblFirstName.Text = emp.FirstName
lblLastName.Text = emp.LastName
End Sub
Private Sub btnShowSerialization_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnShowSerialization.Click
Dim emp As New Student(txtFirstName.Text, txtLastName.Text)
Dim string_writer As New StringWriter()
Dim serializer As New XmlSerializer(GetType(Student))
serializer.Serialize(string_writer, emp)
MessageBox.Show(string_writer.ToString, _
"Serialization", MessageBoxButtons.OK, _
MessageBoxIcon.Information)
End Sub
End Class
<Global.Microsoft.VisualBasic.CompilerServices.DesignerGenerated()> _
Partial Public Class Form1
Inherits System.Windows.Forms.Form
'Form overrides dispose to clean up the component list.
<System.Diagnostics.DebuggerNonUserCode()> _
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing AndAlso components IsNot Nothing Then
components.Dispose()
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> _
Private Sub InitializeComponent()
Me.lblLastName = New System.Windows.Forms.Label
Me.lblFirstName = New System.Windows.Forms.Label
Me.Label3 = New System.Windows.Forms.Label
Me.Label4 = New System.Windows.Forms.Label
Me.btnShowSerialization = New System.Windows.Forms.Button
Me.txtLastName = New System.Windows.Forms.TextBox
Me.txtFirstName = New System.Windows.Forms.TextBox
Me.Label2 = New System.Windows.Forms.Label
Me.Label1 = New System.Windows.Forms.Label
Me.lblDropTarget = New System.Windows.Forms.Label
Me.lblDragSource = New System.Windows.Forms.Label
Me.SuspendLayout()
'
'lblLastName
'
Me.lblLastName.BorderStyle = System.Windows.Forms.BorderStyle.Fixed3D
Me.lblLastName.Location = New System.Drawing.Point(288, 104)
Me.lblLastName.Name = "lblLastName"
Me.lblLastName.Size = New System.Drawing.Size(136, 20)
Me.lblLastName.TabIndex = 28
Me.lblLastName.TextAlign = System.Drawing.ContentAlignment.MiddleLeft
'
'lblFirstName
'
Me.lblFirstName.BorderStyle = System.Windows.Forms.BorderStyle.Fixed3D
Me.lblFirstName.Location = New System.Drawing.Point(288, 80)
Me.lblFirstName.Name = "lblFirstName"
Me.lblFirstName.Size = New System.Drawing.Size(136, 20)
Me.lblFirstName.TabIndex = 27
Me.lblFirstName.TextAlign = System.Drawing.ContentAlignment.MiddleLeft
'
'Label3
'
Me.Label3.AutoSize = True
Me.Label3.Location = New System.Drawing.Point(224, 104)
Me.Label3.Name = "Label3"
Me.Label3.Size = New System.Drawing.Size(54, 13)
Me.Label3.TabIndex = 26
Me.Label3.Text = "Last Name"
'
'Label4
'
Me.Label4.AutoSize = True
Me.Label4.Location = New System.Drawing.Point(224, 80)
Me.Label4.Name = "Label4"
Me.Label4.Size = New System.Drawing.Size(53, 13)
Me.Label4.TabIndex = 25
Me.Label4.Text = "First Name"
'
'btnShowSerialization
'
Me.btnShowSerialization.Location = New System.Drawing.Point(56, 136)
Me.btnShowSerialization.Name = "btnShowSerialization"
Me.btnShowSerialization.Size = New System.Drawing.Size(200, 24)
Me.btnShowSerialization.TabIndex = 24
Me.btnShowSerialization.Text = "Show Serialization"
'
'txtLastName
'
Me.txtLastName.Location = New System.Drawing.Point(72, 104)
Me.txtLastName.Name = "txtLastName"
Me.txtLastName.Size = New System.Drawing.Size(136, 20)
Me.txtLastName.TabIndex = 23
Me.txtLastName.Text = "Last Name"
'
'txtFirstName
'
Me.txtFirstName.Location = New System.Drawing.Point(72, 80)
Me.txtFirstName.Name = "txtFirstName"
Me.txtFirstName.Size = New System.Drawing.Size(136, 20)
Me.txtFirstName.TabIndex = 22
Me.txtFirstName.Text = "First Name"
'
'Label2
'
Me.Label2.AutoSize = True
Me.Label2.Location = New System.Drawing.Point(8, 104)
Me.Label2.Name = "Label2"
Me.Label2.Size = New System.Drawing.Size(54, 13)
Me.Label2.TabIndex = 21
Me.Label2.Text = "Last Name"
'
'Label1
'
Me.Label1.AutoSize = True
Me.Label1.Location = New System.Drawing.Point(8, 80)
Me.Label1.Name = "Label1"
Me.Label1.Size = New System.Drawing.Size(53, 13)
Me.Label1.TabIndex = 20
Me.Label1.Text = "First Name"
'
'lblDropTarget
'
Me.lblDropTarget.AllowDrop = True
Me.lblDropTarget.BorderStyle = System.Windows.Forms.BorderStyle.Fixed3D
Me.lblDropTarget.Location = New System.Drawing.Point(224, 8)
Me.lblDropTarget.Name = "lblDropTarget"
Me.lblDropTarget.Size = New System.Drawing.Size(200, 64)
Me.lblDropTarget.TabIndex = 19
Me.lblDropTarget.Text = "Drop Target"
'
'lblDragSource
'
Me.lblDragSource.BorderStyle = System.Windows.Forms.BorderStyle.Fixed3D
Me.lblDragSource.Location = New System.Drawing.Point(8, 8)
Me.lblDragSource.Name = "lblDragSource"
Me.lblDragSource.Size = New System.Drawing.Size(200, 64)
Me.lblDragSource.TabIndex = 18
Me.lblDragSource.Text = "Drag Source"
'
'Form1
'
Me.AutoScaleDimensions = New System.Drawing.SizeF(6.0!, 13.0!)
Me.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font
Me.ClientSize = New System.Drawing.Size(433, 177)
Me.Controls.Add(Me.lblLastName)
Me.Controls.Add(Me.lblFirstName)
Me.Controls.Add(Me.Label3)
Me.Controls.Add(Me.Label4)
Me.Controls.Add(Me.btnShowSerialization)
Me.Controls.Add(Me.txtLastName)
Me.Controls.Add(Me.txtFirstName)
Me.Controls.Add(Me.Label2)
Me.Controls.Add(Me.Label1)
Me.Controls.Add(Me.lblDropTarget)
Me.Controls.Add(Me.lblDragSource)
Me.Name = "Form1"
Me.Text = "DragStudent2"
Me.ResumeLayout(False)
Me.PerformLayout()
End Sub
Friend WithEvents lblLastName As System.Windows.Forms.Label
Friend WithEvents lblFirstName As System.Windows.Forms.Label
Friend WithEvents Label3 As System.Windows.Forms.Label
Friend WithEvents Label4 As System.Windows.Forms.Label
Friend WithEvents btnShowSerialization As System.Windows.Forms.Button
Friend WithEvents txtLastName As System.Windows.Forms.TextBox
Friend WithEvents txtFirstName As System.Windows.Forms.TextBox
Friend WithEvents Label2 As System.Windows.Forms.Label
Friend WithEvents Label1 As System.Windows.Forms.Label
Friend WithEvents lblDropTarget As System.Windows.Forms.Label
Friend WithEvents lblDragSource As System.Windows.Forms.Label
End Class
Related examples in the same category