Font Properties in Bigger Font size
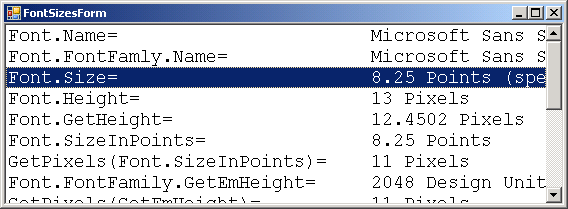
Imports System
Imports System.ComponentModel
Imports System.Windows.Forms
Imports System.Data
Imports System.Configuration
Imports System.Drawing
Imports System.Drawing.Drawing2D
Imports System.Drawing.Text
Imports System.Globalization
Imports System.Text
Imports System.Collections
Public Class MainClass
Shared Sub Main()
Dim myform As Form = New FontSizesForm()
Application.Run(myform)
End Sub
End Class
Public Class FontSizesForm
Inherits System.Windows.Forms.Form
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
Friend WithEvents listBox1 As System.Windows.Forms.ListBox
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.listBox1 = New System.Windows.Forms.ListBox()
Me.SuspendLayout()
'
'listBox1
'
Me.listBox1.Dock = System.Windows.Forms.DockStyle.Fill
Me.listBox1.Font = New System.Drawing.Font("Courier New", 14.0!)
Me.listBox1.IntegralHeight = False
Me.listBox1.ItemHeight = 21
Me.listBox1.Name = "listBox1"
Me.listBox1.Size = New System.Drawing.Size(560, 182)
Me.listBox1.TabIndex = 1
'
'FontSizesForm
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.ClientSize = New System.Drawing.Size(560, 182)
Me.Controls.AddRange(New System.Windows.Forms.Control() {Me.listBox1})
Me.Name = "FontSizesForm"
Me.Text = "FontSizesForm"
Me.ResumeLayout(False)
End Sub
#End Region
Private Function GetPixelsFromPoints(ByVal points As Single, ByVal dpi As Single) As Single
Return (dpi * points) / 72
End Function
Private Function GetInchesFromPoints(ByVal points As Single, ByVal dpi As Single) As Single
Return GetPixelsFromPoints(points, dpi) / dpi
End Function
Private Function GetPixelsFromDesignUnits(ByVal designUnits As Single, ByVal font As Font, ByVal dpi As Single) As Single
Dim scale As Single = GetPixelsFromPoints(font.Size, dpi) / font.FontFamily.GetEmHeight(font.Style)
Return designUnits * scale
End Function
Private Function GetPointsFromPixels(ByVal pixels As Single, ByVal dpi As Single) As Single
Return (pixels * 72) / dpi
End Function
Private Sub FontSizesForm_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles MyBase.Load
Dim g As Graphics = Me.CreateGraphics()
listBox1.Items.Add(String.Format("Font.Name= {0}", Me.Font.Name))
listBox1.Items.Add(String.Format("Font.FontFamly.Name= {0}", Me.Font.FontFamily.Name))
listBox1.Items.Add(String.Format("Font.Size= {0} {1}s (specified Unit)", Me.Font.Size, Me.Font.Unit.ToString()))
listBox1.Items.Add(String.Format("Font.Height= {0} Pixels", Me.Font.Height))
listBox1.Items.Add(String.Format("Font.GetHeight= {0} Pixels", Me.Font.GetHeight(g)))
listBox1.Items.Add(String.Format("Font.SizeInPoints= {0} Points", Me.Font.SizeInPoints.ToString()))
listBox1.Items.Add(String.Format("GetPixels(Font.SizeInPoints)= {0} Pixels", GetPixelsFromPoints(Me.Font.SizeInPoints, g.DpiY)))
'listBox1.Items.Add(string.Format("GetInches(Font.SizeInPoints)= {0} Inches", GetInchesFromPoints(Me.Font.SizeInPoints, g.DpiY)))
listBox1.Items.Add(String.Format("Font.FontFamily.GetEmHeight= {0} Design Units", Me.Font.FontFamily.GetEmHeight(FontStyle.Regular)))
listBox1.Items.Add(String.Format("GetPixels(GetEmHeight)= {0} Pixels", GetPixelsFromDesignUnits(Me.Font.FontFamily.GetEmHeight(Me.Font.Style), Me.Font, g.DpiY)))
listBox1.Items.Add(String.Format("Font.FontFamily.GetLineSpacing= {0} Design Units", Me.Font.FontFamily.GetLineSpacing(FontStyle.Regular)))
listBox1.Items.Add(String.Format("GetPixels(GetLineSpacing)= {0} Pixels", GetPixelsFromDesignUnits(Me.Font.FontFamily.GetLineSpacing(Me.Font.Style), Me.Font, g.DpiY)))
listBox1.Items.Add(String.Format("Font.FontFamily.GetCellAscent= {0} Design Units", Me.Font.FontFamily.GetCellAscent(FontStyle.Regular)))
listBox1.Items.Add(String.Format("GetPixels(GetCellAscent)= {0} Pixels", GetPixelsFromDesignUnits(Me.Font.FontFamily.GetCellAscent(Me.Font.Style), Me.Font, g.DpiY)))
listBox1.Items.Add(String.Format("Font.FontFamily.GetCellDescent= {0} Design Units", Me.Font.FontFamily.GetCellDescent(FontStyle.Regular)))
listBox1.Items.Add(String.Format("GetPixels(GetCellDescent)= {0} Pixels", GetPixelsFromDesignUnits(Me.Font.FontFamily.GetCellDescent(Me.Font.Style), Me.Font, g.DpiY)))
listBox1.Items.Add(String.Format("Padding= {0} Design Units", Me.Font.FontFamily.GetLineSpacing(FontStyle.Regular) - (Me.Font.FontFamily.GetCellAscent(FontStyle.Regular) + Me.Font.FontFamily.GetCellDescent(FontStyle.Regular))))
listBox1.Items.Add(String.Format("GetPixels(Padding)= {0} Pixels", GetPixelsFromDesignUnits(Me.Font.FontFamily.GetLineSpacing(FontStyle.Regular) - (Me.Font.FontFamily.GetCellAscent(FontStyle.Regular) + Me.Font.FontFamily.GetCellDescent(FontStyle.Regular)), Me.Font, g.DpiY)))
End Sub
End Class
Related examples in the same category