Get Installed Font and display one by one
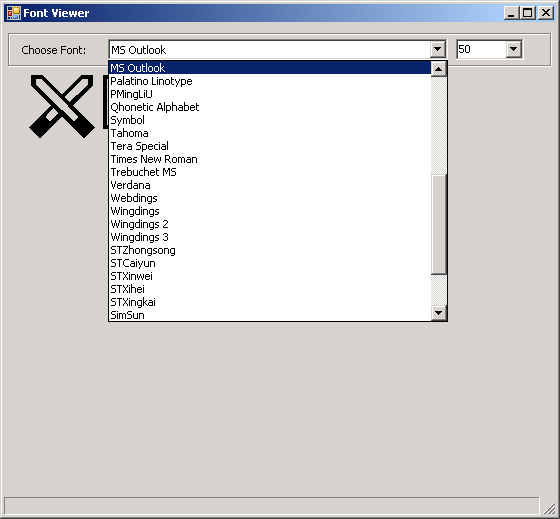
Imports System
Imports System.Drawing
Imports System.Windows.Forms
Imports System.IO
public class MainClass
Shared Sub Main()
Dim form1 As Form = New FontViewer
Application.Run(form1)
End Sub
End Class
Public Class FontViewer
Inherits System.Windows.Forms.Form
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
Friend WithEvents StatusBar As System.Windows.Forms.StatusBar
Friend WithEvents GroupBox1 As System.Windows.Forms.GroupBox
Friend WithEvents lstFonts As System.Windows.Forms.ComboBox
Friend WithEvents label1 As System.Windows.Forms.Label
Friend WithEvents Panel As System.Windows.Forms.StatusBarPanel
Friend WithEvents lstSize As System.Windows.Forms.ComboBox
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.StatusBar = New System.Windows.Forms.StatusBar()
Me.Panel = New System.Windows.Forms.StatusBarPanel()
Me.GroupBox1 = New System.Windows.Forms.GroupBox()
Me.lstSize = New System.Windows.Forms.ComboBox()
Me.lstFonts = New System.Windows.Forms.ComboBox()
Me.label1 = New System.Windows.Forms.Label()
CType(Me.Panel, System.ComponentModel.ISupportInitialize).BeginInit()
Me.GroupBox1.SuspendLayout()
Me.SuspendLayout()
'
'StatusBar
'
Me.StatusBar.Location = New System.Drawing.Point(0, 150)
Me.StatusBar.Name = "StatusBar"
Me.StatusBar.Panels.AddRange(New System.Windows.Forms.StatusBarPanel() {Me.Panel})
Me.StatusBar.ShowPanels = True
Me.StatusBar.Size = New System.Drawing.Size(552, 20)
Me.StatusBar.TabIndex = 3
'
'Panel
'
Me.Panel.AutoSize = System.Windows.Forms.StatusBarPanelAutoSize.Spring
Me.Panel.Width = 536
'
'GroupBox1
'
Me.GroupBox1.Anchor = ((System.Windows.Forms.AnchorStyles.Top Or System.Windows.Forms.AnchorStyles.Left) _
Or System.Windows.Forms.AnchorStyles.Right)
Me.GroupBox1.Controls.AddRange(New System.Windows.Forms.Control() {Me.lstSize, Me.lstFonts, Me.label1})
Me.GroupBox1.FlatStyle = System.Windows.Forms.FlatStyle.System
Me.GroupBox1.Font = New System.Drawing.Font("Tahoma", 8.25!, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, CType(0, Byte))
Me.GroupBox1.Location = New System.Drawing.Point(4, 4)
Me.GroupBox1.Name = "GroupBox1"
Me.GroupBox1.Size = New System.Drawing.Size(544, 40)
Me.GroupBox1.TabIndex = 2
Me.GroupBox1.TabStop = False
'
'lstSize
'
Me.lstSize.DropDownWidth = 40
Me.lstSize.Location = New System.Drawing.Point(448, 12)
Me.lstSize.MaxDropDownItems = 20
Me.lstSize.Name = "lstSize"
Me.lstSize.Size = New System.Drawing.Size(68, 21)
Me.lstSize.TabIndex = 2
'
'lstFonts
'
Me.lstFonts.DropDownStyle = System.Windows.Forms.ComboBoxStyle.DropDownList
Me.lstFonts.DropDownWidth = 340
Me.lstFonts.Location = New System.Drawing.Point(100, 12)
Me.lstFonts.MaxDropDownItems = 20
Me.lstFonts.Name = "lstFonts"
Me.lstFonts.Size = New System.Drawing.Size(340, 21)
Me.lstFonts.TabIndex = 1
'
'label1
'
Me.label1.Location = New System.Drawing.Point(12, 16)
Me.label1.Name = "label1"
Me.label1.Size = New System.Drawing.Size(80, 12)
Me.label1.TabIndex = 0
Me.label1.Text = "Choose Font:"
'
'FontViewer
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.ClientSize = New System.Drawing.Size(552, 170)
Me.Controls.AddRange(New System.Windows.Forms.Control() {Me.StatusBar, Me.GroupBox1})
Me.Name = "FontViewer"
Me.Text = "Font Viewer"
CType(Me.Panel, System.ComponentModel.ISupportInitialize).EndInit()
Me.GroupBox1.ResumeLayout(False)
Me.ResumeLayout(False)
End Sub
#End Region
Private Sub lstFonts_SelectedIndexChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles lstFonts.SelectedIndexChanged
' Trigger a refresh and repaint.
If lstFonts.SelectedIndex <> -1 Then Me.Invalidate()
End Sub
Private Sub FontViewer_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
Dim Fonts As New System.Drawing.Text.InstalledFontCollection()
Dim Family As FontFamily
For Each Family In Fonts.Families
lstFonts.Items.Add(Family.Name)
Next
Dim i As Integer
For i = 10 To 100
lstSize.Items.Add(i)
Next
lstSize.Text = "50"
End Sub
Private Sub FontViewer_Paint(ByVal sender As Object, ByVal e As System.Windows.Forms.PaintEventArgs) Handles MyBase.Paint
If lstFonts.SelectedIndex <> -1 Then
Try
e.Graphics.DrawString(lstFonts.Text, New Font(lstFonts.Text, Val(lstSize.Text)), Brushes.Black, 10, 50)
StatusBar.Panels(0).Text = ""
Catch err As Exception
StatusBar.Panels(0).Text = err.Message
End Try
End If
End Sub
Private Sub lstSize_SelectedIndexChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles lstSize.SelectedIndexChanged
If Val(lstSize.Text) = 0 Then
lstSize.Text = "50"
End If
Me.Invalidate()
End Sub
End Class
Related examples in the same category