Keep the UI from becoming non-responsive in single threaded application which performs a long operation.
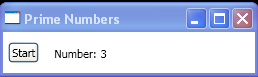
<Window x:Class="WpfApplication1.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Prime Numbers" Width="260" Height="75">
<StackPanel Orientation="Horizontal" VerticalAlignment="Center" >
<Button Content="Start" Click="StartOrStop" Name="startStopButton" Margin="5,0,5,0"/>
<TextBlock Margin="10,5,0,0">Number:</TextBlock>
<TextBlock Name="numberTextBlock" Margin="4,5,0,0">3</TextBlock>
</StackPanel>
</Window>
//File:Window.xaml.vb
Imports System
Imports System.Windows
Imports System.Windows.Controls
Imports System.Windows.Threading
Imports System.Threading
Namespace WpfApplication1
Public Partial Class Window1
Inherits Window
Public Delegate Sub NextPrimeDelegate()
Private num As Long = 1
Private continueCalculating As Boolean = False
Public Sub New()
MyBase.New()
InitializeComponent()
End Sub
Public Sub StartOrStop(sender As Object, e As EventArgs)
If continueCalculating Then
continueCalculating = False
startStopButton.Content = "Resume"
Else
continueCalculating = True
startStopButton.Content = "Stop"
startStopButton.Dispatcher.BeginInvoke(DispatcherPriority.Normal, New NextPrimeDelegate(AddressOf CheckNextNumber))
End If
End Sub
Public Sub CheckNextNumber()
numberTextBlock.Text = num.ToString()
num += 2
If continueCalculating Then
startStopButton.Dispatcher.BeginInvoke(System.Windows.Threading.DispatcherPriority.SystemIdle, New NextPrimeDelegate(AddressOf Me.CheckNextNumber))
End If
End Sub
End Class
End Namespace
Related examples in the same category