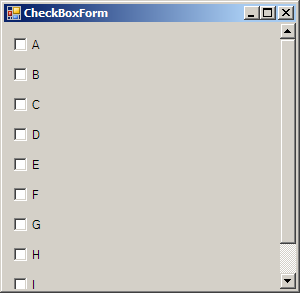
using System;
using System.Windows.Forms;
public class CheckBoxFormDemo{
[STAThread]
public static void Main(string[] args)
{
Application.Run(new CheckBoxForm());
}
}
public partial class CheckBoxForm : Form
{
public CheckBoxForm()
{
InitializeComponent();
}
protected override void OnLoad(EventArgs e)
{
base.OnLoad(e);
string[] foods = {"A", "B", "C", "D","E","F","G","H","I","J"};
this.SuspendLayout();
int topPosition = 10;
foreach (string food in foods)
{
CheckBox checkBox = new CheckBox();
checkBox.Top = topPosition;
checkBox.Left = 10;
checkBox.Text = food;
topPosition += 30;
panel1.Controls.Add(checkBox);
}
this.ResumeLayout();
}
}
partial class CheckBoxForm
{
private System.ComponentModel.IContainer components = null;
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
private void InitializeComponent()
{
this.panel1 = new System.Windows.Forms.Panel();
this.SuspendLayout();
//
// panel1
//
this.panel1.AutoScroll = true;
this.panel1.Dock = System.Windows.Forms.DockStyle.Fill;
this.panel1.Location = new System.Drawing.Point(0, 0);
this.panel1.Name = "panel1";
this.panel1.Size = new System.Drawing.Size(292, 266);
this.panel1.TabIndex = 0;
//
// CheckBoxForm
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(292, 266);
this.Controls.Add(this.panel1);
this.Name = "CheckBoxForm";
this.Text = "CheckBoxForm";
this.ResumeLayout(false);
}
#endregion
private System.Windows.Forms.Panel panel1;
}