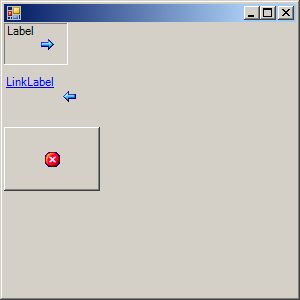
using System;
using System.Drawing;
using System.Windows.Forms;
using System.Collections;
public class ImageLists : Form
{
ImageList imgList;
Label lbl;
LinkLabel lnk;
Button btn;
public ImageLists()
{
Size = new Size(300,300);
imgList = new ImageList();
Image img;
String[] arFiles = {"1.ico","2.ico","3.ico"};
for (int i = 0; i < arFiles.Length; i++)
{
img = Image.FromFile(arFiles[i]);
imgList.Images.Add(img);
}
imgList.ImageSize = new Size(32, 32);
lbl = new Label();
lbl.Parent = this;
lbl.Text = "Label";
lbl.Location = new Point(0,0);
lbl.Size = new Size(lbl.PreferredWidth + imgList.ImageSize.Width,
imgList.ImageSize.Height + 10);
lbl.BorderStyle = BorderStyle.Fixed3D;
lbl.ImageList = imgList;
lbl.ImageIndex = 0;
lbl.ImageAlign = ContentAlignment.MiddleRight;
int yDelta = lbl.Height + 10;
lnk = new LinkLabel();
lnk.Parent = this;
lnk.Text = "LinkLabel";
lnk.Size = new Size(lnk.PreferredWidth + imgList.ImageSize.Width,
imgList.ImageSize.Height + 10);
lnk.Location = new Point(0, yDelta);
lnk.ImageList = imgList;
lnk.ImageIndex = 0;
lnk.ImageAlign = ContentAlignment.MiddleRight;
btn = new Button();
btn.Parent = this;
btn.ImageList = imgList;
btn.ImageIndex = imgList.Images.Count - 1;
btn.Location = new Point(0, 2 * yDelta);
btn.Size = new Size(3 * imgList.ImageSize.Width,
2 * imgList.ImageSize.Height);
lbl.ImageIndex = 1;
lnk.ImageIndex = 0;
btn.ImageIndex = 2;
}
static void Main()
{
Application.Run(new ImageLists());
}
}