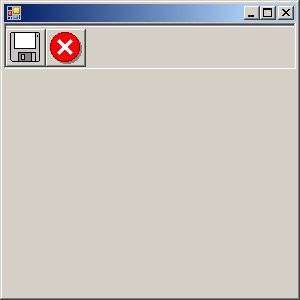
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
public class ImageListCreateAndAddToToolBar : System.Windows.Forms.Form
{
private ImageList toolBarIcons;
private ToolBarButton tbExitButton;
private ToolBarButton tbSaveButton;
private ToolBar toolBar;
private System.ComponentModel.Container components = null;
public ImageListCreateAndAddToToolBar()
{
this.components = new System.ComponentModel.Container();
this.Size = new System.Drawing.Size(300,300);
toolBar = new ToolBar();
toolBarIcons = new ImageList();
tbSaveButton = new ToolBarButton();
tbExitButton = new ToolBarButton();
tbSaveButton.ImageIndex = 0;
tbExitButton.ImageIndex = 1;
toolBar.ImageList = toolBarIcons;
toolBar.Size = new System.Drawing.Size(272, 28);
toolBar.BorderStyle = System.Windows.Forms.BorderStyle.Fixed3D;
toolBar.ShowToolTips = true;
toolBar.Buttons.AddRange(new ToolBarButton[] {tbSaveButton, tbExitButton});
toolBar.ButtonClick += new ToolBarButtonClickEventHandler(ToolBar_Clicked);
toolBarIcons.ImageSize = new System.Drawing.Size(32, 32);
toolBarIcons.Images.Add(new Icon("filesave.ico"));
toolBarIcons.Images.Add(new Icon("fileexit.ico"));
toolBarIcons.ColorDepth = ColorDepth.Depth16Bit;
toolBarIcons.TransparentColor = System.Drawing.Color.Transparent;
this.Controls.Add(toolBar);
}
[STAThread]
static void Main()
{
Application.Run(new ImageListCreateAndAddToToolBar());
}
private void ToolBar_Clicked(object sender, ToolBarButtonClickEventArgs e)
{
MessageBox.Show(e.Button.ToolTipText);
}
}