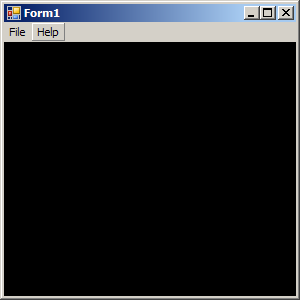
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
public class FormWithMenu : System.Windows.Forms.Form
{
private MainMenu mainMenu;
private System.ComponentModel.Container components = null;
public FormWithMenu()
{
InitializeComponent();
mainMenu = new MainMenu();
MenuItem miFile = mainMenu.MenuItems.Add("&File");
miFile.MenuItems.Add(new MenuItem("E&xit", new EventHandler(this.FileExit_Clicked), Shortcut.CtrlX));
MenuItem miHelp = mainMenu.MenuItems.Add("Help");
miHelp.MenuItems.Add(new MenuItem("&About", new EventHandler(this.HelpAbout_Clicked),Shortcut.CtrlA));
this.Menu = mainMenu;
mainMenu.GetForm().BackColor = Color.Black;
}
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
private void InitializeComponent()
{
this.components = new System.ComponentModel.Container();
this.Size = new System.Drawing.Size(300,300);
this.Text = "Form1";
}
private void FileExit_Clicked(object sender, EventArgs e)
{
this.Close();
}
private void HelpAbout_Clicked(object sender, EventArgs e)
{
MessageBox.Show("Help");
}
[STAThread]
static void Main()
{
Application.Run(new FormWithMenu());
}
}