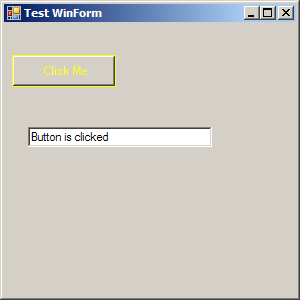
using System;
using System.Windows.Forms;
using System.Drawing;
public class ButtonClickEvent : System.Windows.Forms.Form
{
private System.Windows.Forms.Button button1;
private System.Windows.Forms.TextBox textBox1;
public ButtonClickEvent()
{
Text = "Test WinForm";
ForeColor = System.Drawing.Color.Yellow;
button1 = new System.Windows.Forms.Button();
textBox1 = new System.Windows.Forms.TextBox();
// button control and its properties
button1.Location = new System.Drawing.Point(8, 32);
button1.Name = "button1";
button1.Size = new System.Drawing.Size(104, 32);
button1.TabIndex = 0;
button1.Text = "Click Me";
// text box control and its properties
textBox1.Location = new System.Drawing.Point(24, 104);
textBox1.Name = "textBox1";
textBox1.Size = new System.Drawing.Size(184, 20);
textBox1.TabIndex = 1;
textBox1.Text = "textBox1";
// Adding controls to the fomr
Controls.AddRange(new System.Windows.Forms.Control[]{textBox1, button1} );
button1.Click += new System.EventHandler(button1_Click);
}
private void button1_Click(object sender,System.EventArgs e)
{
textBox1.Text = "Button is clicked";
MessageBox.Show("Button is clicked");
}
public static int Main()
{
Application.Run(new ButtonClickEvent());
return 0;
}
}