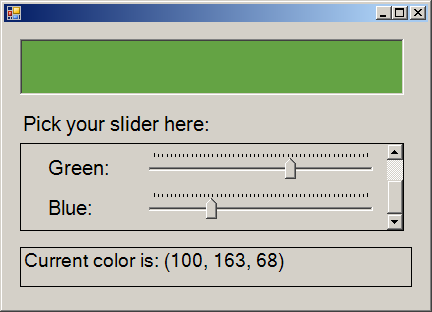
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
public class TrackColorForm : System.Windows.Forms.Form
{
private System.ComponentModel.Container components = null;
private System.Windows.Forms.Label label4;
private System.Windows.Forms.Label label2;
private System.Windows.Forms.TrackBar blueTrackBar;
private System.Windows.Forms.Label label3;
private System.Windows.Forms.TrackBar greenTrackBar;
private System.Windows.Forms.TrackBar redTrackBar;
private System.Windows.Forms.Label label1;
private System.Windows.Forms.Panel panel1;
private System.Windows.Forms.Label lblCurrColor;
private System.Windows.Forms.PictureBox colorBox;
public TrackColorForm()
{
InitializeComponent();
redTrackBar.Value = 100;
greenTrackBar.Value = 255;
blueTrackBar.Value = 0;
UpdateColor();
}
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
private void InitializeComponent()
{
this.label4 = new System.Windows.Forms.Label();
this.label1 = new System.Windows.Forms.Label();
this.label3 = new System.Windows.Forms.Label();
this.label2 = new System.Windows.Forms.Label();
this.panel1 = new System.Windows.Forms.Panel();
this.blueTrackBar = new System.Windows.Forms.TrackBar();
this.greenTrackBar = new System.Windows.Forms.TrackBar();
this.redTrackBar = new System.Windows.Forms.TrackBar();
this.colorBox = new System.Windows.Forms.PictureBox();
this.lblCurrColor = new System.Windows.Forms.Label();
this.panel1.SuspendLayout();
((System.ComponentModel.ISupportInitialize)(this.blueTrackBar)).BeginInit();
((System.ComponentModel.ISupportInitialize)(this.greenTrackBar)).BeginInit();
((System.ComponentModel.ISupportInitialize)(this.redTrackBar)).BeginInit();
this.SuspendLayout();
//
// label4
//
this.label4.Font = new System.Drawing.Font("Microsoft Sans Serif", 15F);
this.label4.Location = new System.Drawing.Point(16, 88);
this.label4.Name = "label4";
this.label4.Size = new System.Drawing.Size(240, 32);
this.label4.TabIndex = 9;
this.label4.Text = "Pick your slider here:";
//
// label1
//
this.label1.Font = new System.Drawing.Font("Arial", 15F);
this.label1.Location = new System.Drawing.Point(24, 16);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(88, 32);
this.label1.TabIndex = 4;
this.label1.Text = "Red:";
//
// label3
//
this.label3.Font = new System.Drawing.Font("Arial", 15F);
this.label3.Location = new System.Drawing.Point(24, 104);
this.label3.Name = "label3";
this.label3.Size = new System.Drawing.Size(88, 32);
this.label3.TabIndex = 6;
this.label3.Text = "Blue:";
//
// label2
//
this.label2.Font = new System.Drawing.Font("Arial", 15F);
this.label2.Location = new System.Drawing.Point(24, 64);
this.label2.Name = "label2";
this.label2.Size = new System.Drawing.Size(88, 32);
this.label2.TabIndex = 5;
this.label2.Text = "Green:";
//
// panel1
//
this.panel1.AutoScroll = true;
this.panel1.BorderStyle = System.Windows.Forms.BorderStyle.FixedSingle;
this.panel1.Controls.Add(this.label2);
this.panel1.Controls.Add(this.blueTrackBar);
this.panel1.Controls.Add(this.label3);
this.panel1.Controls.Add(this.greenTrackBar);
this.panel1.Controls.Add(this.redTrackBar);
this.panel1.Controls.Add(this.label1);
this.panel1.Location = new System.Drawing.Point(16, 120);
this.panel1.Name = "panel1";
this.panel1.Size = new System.Drawing.Size(384, 88);
this.panel1.TabIndex = 8;
//
// blueTrackBar
//
this.blueTrackBar.Location = new System.Drawing.Point(120, 96);
this.blueTrackBar.Maximum = 255;
this.blueTrackBar.Name = "blueTrackBar";
this.blueTrackBar.Size = new System.Drawing.Size(240, 45);
this.blueTrackBar.TabIndex = 1;
this.blueTrackBar.TickFrequency = 5;
this.blueTrackBar.TickStyle = System.Windows.Forms.TickStyle.TopLeft;
this.blueTrackBar.Scroll += new System.EventHandler(this.blueTrackBar_Scroll);
//
// greenTrackBar
//
this.greenTrackBar.Location = new System.Drawing.Point(120, 56);
this.greenTrackBar.Maximum = 255;
this.greenTrackBar.Name = "greenTrackBar";
this.greenTrackBar.Size = new System.Drawing.Size(240, 45);
this.greenTrackBar.TabIndex = 3;
this.greenTrackBar.TickFrequency = 5;
this.greenTrackBar.TickStyle = System.Windows.Forms.TickStyle.TopLeft;
this.greenTrackBar.Scroll += new System.EventHandler(this.greenTrackBar_Scroll);
//
// redTrackBar
//
this.redTrackBar.Location = new System.Drawing.Point(120, 16);
this.redTrackBar.Maximum = 255;
this.redTrackBar.Name = "redTrackBar";
this.redTrackBar.Size = new System.Drawing.Size(232, 45);
this.redTrackBar.TabIndex = 2;
this.redTrackBar.TickFrequency = 5;
this.redTrackBar.TickStyle = System.Windows.Forms.TickStyle.TopLeft;
this.redTrackBar.Scroll += new System.EventHandler(this.redTrackBar_Scroll);
//
// colorBox
//
this.colorBox.BackColor = System.Drawing.Color.Blue;
this.colorBox.BorderStyle = System.Windows.Forms.BorderStyle.Fixed3D;
this.colorBox.Location = new System.Drawing.Point(16, 16);
this.colorBox.Name = "colorBox";
this.colorBox.Size = new System.Drawing.Size(384, 56);
this.colorBox.TabIndex = 0;
this.colorBox.TabStop = false;
//
// lblCurrColor
//
this.lblCurrColor.BorderStyle = System.Windows.Forms.BorderStyle.FixedSingle;
this.lblCurrColor.Font = new System.Drawing.Font("Microsoft Sans Serif", 14F);
this.lblCurrColor.Location = new System.Drawing.Point(16, 224);
this.lblCurrColor.Name = "lblCurrColor";
this.lblCurrColor.Size = new System.Drawing.Size(392, 40);
this.lblCurrColor.TabIndex = 7;
this.lblCurrColor.Text = "label4";
//
// TrackColorForm
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(424, 285);
this.Controls.Add(this.label4);
this.Controls.Add(this.panel1);
this.Controls.Add(this.lblCurrColor);
this.Controls.Add(this.colorBox);
this.panel1.ResumeLayout(false);
((System.ComponentModel.ISupportInitialize)(this.blueTrackBar)).EndInit();
((System.ComponentModel.ISupportInitialize)(this.greenTrackBar)).EndInit();
((System.ComponentModel.ISupportInitialize)(this.redTrackBar)).EndInit();
this.ResumeLayout(false);
}
[STAThread]
static void Main()
{
Application.Run(new TrackColorForm());
}
protected void greenTrackBar_Scroll (object sender, System.EventArgs e)
{
UpdateColor();
}
protected void redTrackBar_Scroll (object sender, System.EventArgs e)
{
UpdateColor();
}
protected void blueTrackBar_Scroll (object sender, System.EventArgs e)
{
UpdateColor();
}
private void UpdateColor()
{
Color c = Color.FromArgb(redTrackBar.Value, greenTrackBar.Value, blueTrackBar.Value);
colorBox.BackColor = c;
lblCurrColor.Text = string.Format("Current color is: ({0}, {1}, {2})", redTrackBar.Value, greenTrackBar.Value,blueTrackBar.Value);
}
}