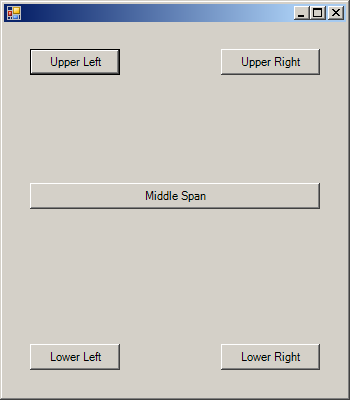
using System;
using System.Drawing;
using System.Windows.Forms;
public class ControlAnchor : Form
{
public ControlAnchor()
{
Size = new Size(350,400);
int xButtonSize, yButtonSize = Font.Height * 2;
int xMargin, yMargin;
xMargin = yMargin = Font.Height * 2;
Button btn = new Button();
btn.Parent = this;
btn.Text = "Upper Left";
xButtonSize = (int)(Font.Height * .75) * btn.Text.Length;
btn.Size = new Size(xButtonSize, yButtonSize);
btn.Location = new Point(xMargin, yMargin);
btn = new Button();
btn.Parent = this;
btn.Text = "Lower Left";
xButtonSize = (int)(Font.Height * .75) * btn.Text.Length;
btn.Size = new Size(xButtonSize, yButtonSize);
btn.Location = new Point(xMargin, this.ClientSize.Height - yMargin - yButtonSize);
btn.Anchor = AnchorStyles.Bottom | AnchorStyles.Left;
btn = new Button();
btn.Parent = this;
btn.Text = "Upper Right";
xButtonSize = (int)(Font.Height * .75) * btn.Text.Length;
btn.Size = new Size(xButtonSize, yButtonSize);
btn.Location = new Point(this.ClientSize.Width - xMargin - xButtonSize, yMargin);
btn.Anchor = AnchorStyles.Top | AnchorStyles.Right;
btn = new Button();
btn.Parent = this;
btn.Text = "Lower Right";
xButtonSize = (int)(Font.Height * .75) * btn.Text.Length;
btn.Size = new Size(xButtonSize, yButtonSize);
btn.Location = new Point(this.ClientSize.Width - xMargin - xButtonSize,
this.ClientSize.Height - yMargin - yButtonSize);
btn.Anchor = AnchorStyles.Bottom | AnchorStyles.Right;
btn = new Button();
btn.Parent = this;
btn.Text = "Middle Span";
xButtonSize = this.ClientSize.Width - (2 * xMargin);
btn.Size = new Size(xButtonSize, yButtonSize);
btn.Location = new Point(xMargin,
(int)(this.ClientSize.Height / 2) - yButtonSize);
btn.Anchor = AnchorStyles.Left | AnchorStyles.Right;
}
static void Main()
{
Application.Run(new ControlAnchor());
}
}