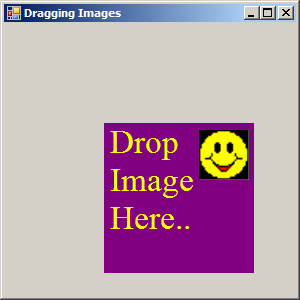
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
public class ImageDragDrop : System.Windows.Forms.Form
{
private System.ComponentModel.Container components = null;
private bool isDragging = false;
private int oldX, oldY;
Rectangle dropRect = new Rectangle(100, 100, 150, 150);
private PictureBox picBox = new PictureBox();
public ImageDragDrop()
{
InitializeComponent();
CenterToScreen();
picBox.SizeMode = PictureBoxSizeMode.StretchImage;
picBox.Location = new System.Drawing.Point(64, 32);
picBox.Size = new System.Drawing.Size(50, 50);
picBox.Image = new Bitmap("YourFile.bmp");
picBox.MouseDown += new MouseEventHandler(picBox_MouseDown);
picBox.MouseUp += new MouseEventHandler(picBox_MouseUp);
picBox.MouseMove += new MouseEventHandler(picBox_MouseMove);
picBox.Cursor = Cursors.Hand;
Controls.Add(picBox);
}
protected override void Dispose( bool disposing )
{
picBox.Dispose();
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
private void InitializeComponent()
{
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(292, 273);
this.Name = "ImageDragDrop";
this.Text = "Dragging Images";
this.Paint += new System.Windows.Forms.PaintEventHandler(this.ImageDragDrop_Paint);
}
[STAThread]
static void Main()
{
Application.Run(new ImageDragDrop());
}
private void picBox_MouseDown(object sender, MouseEventArgs e)
{
isDragging = true;
oldX = e.X;
oldY = e.Y;
}
private void picBox_MouseMove(object sender, MouseEventArgs e)
{
if (isDragging)
{
picBox.Top = picBox.Top + (e.Y - oldY);
picBox.Left = picBox.Left + (e.X - oldX);
}
}
private void picBox_MouseUp(object sender, MouseEventArgs e)
{
isDragging = false;
if(dropRect.Contains(picBox.Bounds))
{
MessageBox.Show("Message", "In Rectangle");
}
}
private void ImageDragDrop_Paint(object sender, System.Windows.Forms.PaintEventArgs e)
{
Graphics g = e.Graphics;
g.FillRectangle(Brushes.Purple, dropRect);
g.DrawString("Drop Image Here..", new Font("Times New Roman", 25),Brushes.Yellow, dropRect);
}
}
23.60.Drag Move |
| 23.60.1. | Drag and Drop Image | 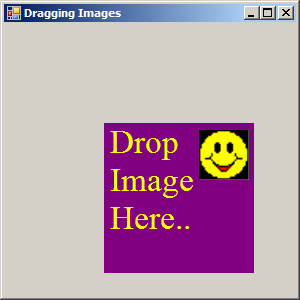 |