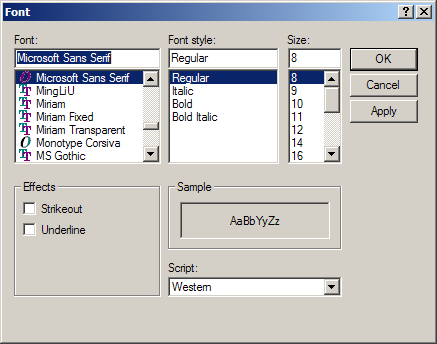
using System;
using System.Drawing;
using System.Windows.Forms;
public class FontProperties : Form
{
private Button btnChange;
private Label lbl;
private FontDialog fd;
public FontProperties()
{
Size = new Size(350,200);
btnChange = new Button();
btnChange.Location = new Point(200,50);
btnChange.Size = new Size(100,23);
btnChange.Text = "Change";
btnChange.Click += new System.EventHandler(btnChange_Click);
btnChange.Parent = this;
lbl = new Label();
lbl.Text = "test";
lbl.AutoSize = true;
lbl.Parent = this;
}
static void Main()
{
Application.Run(new FontProperties());
}
private void btnChange_Click(object sender, EventArgs e)
{
fd = new FontDialog();
fd.ShowHelp = false;
fd.ShowApply = true;
fd.Apply += new System.EventHandler(this.fd_Apply);
if (fd.ShowDialog() == DialogResult.OK)
lbl.Font = fd.Font;
MessageBox.Show("Label Font:\t" + lbl.Font.ToString() + "\n" +
"Label Font Family:\t" + lbl.Font.FontFamily.ToString() + "\n" +
"Label Font Style:\t" + lbl.Font.Style.ToString() + "\n" +
"Label Font Unit:\t" + lbl.Font.Unit.ToString() + "\n" +
"Label Font Height:\t" + lbl.Font.Height.ToString() + "\n",
"Font Properties");
}
private void fd_Apply(object sender, System.EventArgs e)
{
lbl.Font = fd.Font;
}
}