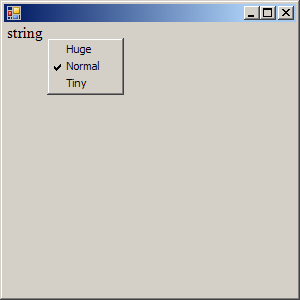
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
public class FormPopupMenu : System.Windows.Forms.Form
{
private int currFontSize = 12;
private ContextMenu popUpMenu;
private MenuItem currentCheckedItem;
private MenuItem checkedHuge;
private MenuItem checkedNormal;
private MenuItem checkedTiny;
private System.ComponentModel.Container components;
public FormPopupMenu()
{
InitializeComponent();
popUpMenu = new ContextMenu();
popUpMenu.MenuItems.Add("Huge", new EventHandler(PopUp_Clicked));
popUpMenu.MenuItems.Add("Normal", new EventHandler(PopUp_Clicked));
popUpMenu.MenuItems.Add("Tiny", new EventHandler(PopUp_Clicked));
this.ContextMenu = popUpMenu;
checkedHuge = this.ContextMenu.MenuItems[0];
checkedNormal = this.ContextMenu.MenuItems[1];
checkedTiny = this.ContextMenu.MenuItems[2];
currentCheckedItem = checkedNormal;
currentCheckedItem.Checked = true;
this.Resize += new System.EventHandler(this.FormPopupMenu_Resize);
this.Paint += new System.Windows.Forms.PaintEventHandler(this.FormPopupMenu_Paint);
}
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
private void InitializeComponent()
{
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(292, 273);
}
[STAThread]
static void Main()
{
Application.Run(new FormPopupMenu());
}
private void PopUp_Clicked(object sender, EventArgs e)
{
currentCheckedItem.Checked = false;
MenuItem miClicked = null;
if (sender is MenuItem)
miClicked = (MenuItem)sender;
else
return;
string item = miClicked.Text;
if(item == "Huge")
{
currFontSize = 18;
currentCheckedItem = checkedHuge;
}
if(item == "Normal")
{
currFontSize = 12;
currentCheckedItem = checkedNormal;
}
if(item == "Tiny")
{
currFontSize = 8;
currentCheckedItem = checkedTiny;
}
currentCheckedItem.Checked = true;
Invalidate();
}
private void FormPopupMenu_Paint(object sender, System.Windows.Forms.PaintEventArgs e)
{
Graphics g = e.Graphics;
g.DrawString("string",
new Font("Times New Roman", (float)currFontSize),
new SolidBrush(Color.Black),
this.DisplayRectangle);
}
private void FormPopupMenu_Resize(object sender, System.EventArgs e)
{
Invalidate();
}
}