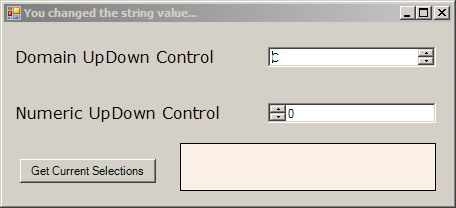
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
public class UpDownForm : System.Windows.Forms.Form
{
private System.ComponentModel.Container components = null;
private System.Windows.Forms.Label lblCurrSel;
private System.Windows.Forms.Button btnGetSelections;
private System.Windows.Forms.Label label2;
private System.Windows.Forms.Label label1;
private System.Windows.Forms.NumericUpDown numericUpDown;
private System.Windows.Forms.DomainUpDown domainUpDown;
public UpDownForm()
{
InitializeComponent();
domainUpDown.SelectedIndex = 2;
}
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
private void InitializeComponent()
{
this.label1 = new System.Windows.Forms.Label();
this.label2 = new System.Windows.Forms.Label();
this.numericUpDown = new System.Windows.Forms.NumericUpDown();
this.domainUpDown = new System.Windows.Forms.DomainUpDown();
this.btnGetSelections = new System.Windows.Forms.Button();
this.lblCurrSel = new System.Windows.Forms.Label();
((System.ComponentModel.ISupportInitialize)(this.numericUpDown)).BeginInit();
this.SuspendLayout();
//
// label1
//
this.label1.Font = new System.Drawing.Font("Verdana", 12F);
this.label1.Location = new System.Drawing.Point(8, 24);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(224, 32);
this.label1.TabIndex = 2;
this.label1.Text = "Domain UpDown Control";
//
// label2
//
this.label2.Font = new System.Drawing.Font("Verdana", 12F);
this.label2.Location = new System.Drawing.Point(8, 80);
this.label2.Name = "label2";
this.label2.Size = new System.Drawing.Size(232, 32);
this.label2.TabIndex = 3;
this.label2.Text = "Numeric UpDown Control";
//
// numericUpDown
//
this.numericUpDown.Location = new System.Drawing.Point(264, 80);
this.numericUpDown.Maximum = new System.Decimal(new int[] {
5000,
0,
0,
0});
this.numericUpDown.Name = "numericUpDown";
this.numericUpDown.Size = new System.Drawing.Size(168, 20);
this.numericUpDown.TabIndex = 1;
this.numericUpDown.ThousandsSeparator = true;
this.numericUpDown.UpDownAlign = System.Windows.Forms.LeftRightAlignment.Left;
this.numericUpDown.ValueChanged += new System.EventHandler(this.numericUpDown_ValueChanged);
//
// domainUpDown
//
this.domainUpDown.Items.Add("A");
this.domainUpDown.Items.Add("B");
this.domainUpDown.Items.Add("C");
this.domainUpDown.Items.Add("D");
this.domainUpDown.Location = new System.Drawing.Point(264, 24);
this.domainUpDown.Name = "domainUpDown";
this.domainUpDown.Size = new System.Drawing.Size(168, 20);
this.domainUpDown.Sorted = true;
this.domainUpDown.TabIndex = 0;
this.domainUpDown.Text = "domainUpDown1";
this.domainUpDown.Wrap = true;
this.domainUpDown.SelectedItemChanged += new System.EventHandler(this.domainUpDown_SelectedItemChanged);
//
// btnGetSelections
//
this.btnGetSelections.Location = new System.Drawing.Point(16, 136);
this.btnGetSelections.Name = "btnGetSelections";
this.btnGetSelections.Size = new System.Drawing.Size(136, 24);
this.btnGetSelections.TabIndex = 4;
this.btnGetSelections.Text = "Get Current Selections";
this.btnGetSelections.Click += new System.EventHandler(this.btnGetSelections_Click);
//
// lblCurrSel
//
this.lblCurrSel.BackColor = System.Drawing.Color.Linen;
this.lblCurrSel.BorderStyle = System.Windows.Forms.BorderStyle.FixedSingle;
this.lblCurrSel.Location = new System.Drawing.Point(176, 120);
this.lblCurrSel.Name = "lblCurrSel";
this.lblCurrSel.Size = new System.Drawing.Size(256, 48);
this.lblCurrSel.TabIndex = 5;
//
// UpDownForm
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(448, 181);
this.Controls.Add(this.lblCurrSel);
this.Controls.Add(this.btnGetSelections);
this.Controls.Add(this.label2);
this.Controls.Add(this.label1);
this.Controls.Add(this.numericUpDown);
this.Controls.Add(this.domainUpDown);
this.Name = "UpDownForm";
this.Text = "Spin Controls";
((System.ComponentModel.ISupportInitialize)(this.numericUpDown)).EndInit();
this.ResumeLayout(false);
}
[STAThread]
static void Main()
{
Application.Run(new UpDownForm());
}
protected void numericUpDown_ValueChanged (object sender, System.EventArgs e)
{
this.Text = "You changed the numeric value...";
}
protected void domainUpDown_SelectedItemChanged (object sender, System.EventArgs e)
{
this.Text = "You changed the string value...";
}
protected void btnGetSelections_Click (object sender, System.EventArgs e)
{
// Get info from updowns...
lblCurrSel.Text = string.Format("String: {0}\nNumber: {1}", domainUpDown.Text, numericUpDown.Value);
}
}