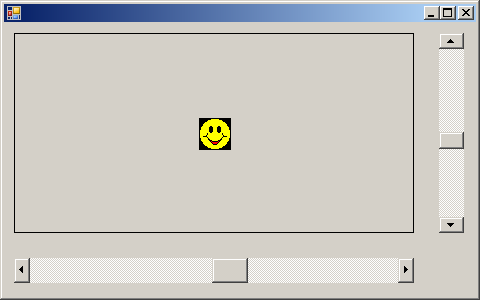
using System;
using System.Drawing;
using System.Windows.Forms;
public class ScrollBarsControlPictureBox : Form
{
Panel pnl;
PictureBox pb;
HScrollBar hbar;
VScrollBar vbar;
Image img;
public ScrollBarsControlPictureBox()
{
Size = new Size(480,300);
img = Image.FromFile("YourFile.gif");
pnl = new Panel();
pnl.Parent = this;
pnl.Size = new Size(400,200);
pnl.Location = new Point(10,10);
pnl.BorderStyle = BorderStyle.FixedSingle;
pb = new PictureBox();
pb.Parent = pnl;
pb.Size = new Size(img.Size.Width, img.Size.Height);
pb.Location = new Point((pnl.Size.Width / 2) - (pb.Size.Width / 2),
(pnl.Size.Height / 2) - (pb.Size.Height / 2));
pb.SizeMode = PictureBoxSizeMode.CenterImage;
pb.Image = img;
hbar = new HScrollBar();
hbar.Parent = this;
hbar.Location = new Point(pnl.Left, pnl.Bottom + 25);
hbar.Size = new Size(pnl.Width, 25);
hbar.Minimum = 0;
hbar.Maximum = 100;
hbar.SmallChange = 1;
hbar.LargeChange = 10;
hbar.Value = (hbar.Maximum - hbar.Minimum) / 2;
hbar.ValueChanged += new EventHandler(hbar_OnValueChanged);
vbar = new VScrollBar();
vbar.Parent = this;
vbar.Location = new Point(pnl.Right + 25, pnl.Top);
vbar.Size = new Size(25, pnl.Height);
vbar.Minimum = 0;
vbar.Maximum = 100;
vbar.SmallChange = 1;
vbar.LargeChange = 10;
vbar.Value = (vbar.Maximum - vbar.Minimum) / 2;
vbar.ValueChanged += new EventHandler(vbar_OnValueChanged);
}
private void hbar_OnValueChanged(object sender, EventArgs e)
{
pb.Location = new Point((pnl.Size.Width - img.Size.Width) * (hbar.Value) / (hbar.Maximum - hbar.LargeChange + 1), pb.Top);
}
private void vbar_OnValueChanged(object sender, EventArgs e)
{
pb.Location = new Point(pb.Left, (pnl.Size.Height - img.Size.Height) * vbar.Value / (vbar.Maximum - vbar.LargeChange + 1));
}
static void Main()
{
Application.Run(new ScrollBarsControlPictureBox());
}
}