<Window xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
x:Class="Height_MinHeight_MaxHeight.Window1"
Title="Height Properties Sample">
<StackPanel Margin="10">
<Canvas Height="200" MinWidth="200" Name="myCanvas">
<Rectangle HorizontalAlignment="Center" Canvas.Top="50" Canvas.Left="50" Name="rect1" Fill="#4682b4" Height="100" Width="100"/>
</Canvas>
<Button Name="Button1" Click="clipRect">Canvas.ClipToBounds="True"</Button>
<Button Name="Button2" Margin="0,5,5,5" Click="unclipRect">Canvas.ClipToBounds="False"</Button>
<TextBlock Grid.Row="1" Grid.Column="2" Margin="10,0,0,0" TextWrapping="Wrap">Set the Rectangle MinHeight:</TextBlock>
<ListBox Grid.Column="3" Grid.Row="1" Margin="10,0,0,0" Height="50" Width="50" SelectionChanged="changeMinHeight">
<ListBoxItem>25</ListBoxItem>
<ListBoxItem>50</ListBoxItem>
<ListBoxItem>75</ListBoxItem>
<ListBoxItem>100</ListBoxItem>
<ListBoxItem>125</ListBoxItem>
<ListBoxItem>150</ListBoxItem>
<ListBoxItem>175</ListBoxItem>
<ListBoxItem>200</ListBoxItem>
</ListBox>
</StackPanel>
</Window>
//File:Window.xaml.cs
using System;
using System.Windows;
using System.Windows.Media;
using System.Windows.Controls;
using System.Windows.Documents;
namespace Height_MinHeight_MaxHeight
{
public partial class Window1 : Window
{
public void changeMinHeight(object sender, SelectionChangedEventArgs args)
{
ListBoxItem li = ((sender as ListBox).SelectedItem as ListBoxItem);
Double sz1 = Double.Parse(li.Content.ToString());
rect1.MinHeight = sz1;
rect1.UpdateLayout();
Console.WriteLine("ActualHeight is set to " + rect1.ActualHeight);
Console.WriteLine("Height is set to " + rect1.Height);
Console.WriteLine("MinHeight is set to " + rect1.MinHeight);
Console.WriteLine("MaxHeight is set to " + rect1.MaxHeight);
}
public void clipRect(object sender, RoutedEventArgs args)
{
myCanvas.ClipToBounds = true;
Console.WriteLine("Canvas.ClipToBounds is set to " + myCanvas.ClipToBounds);
}
public void unclipRect(object sender, RoutedEventArgs args)
{
myCanvas.ClipToBounds = false;
Console.WriteLine("Canvas.ClipToBounds is set to " + myCanvas.ClipToBounds);
}
}
}
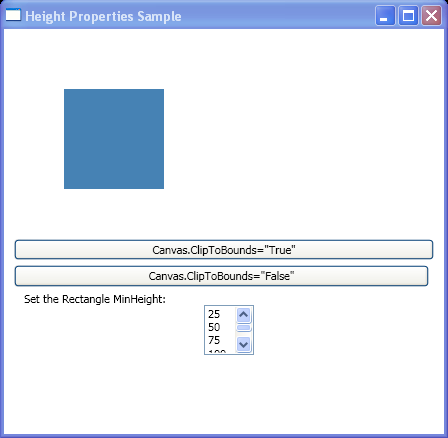